Objective: Print the lyrics for the current playing song.
How: We'll create a small bash
script to do the fetching for us (using curl
) and then we'll
display it either in the terminal or in our $EDITOR
First we'll need to get the name of the current song and its artist:
iTunes (Mountain Lion+):
osascript -e'tell application "iTunes"' -e'get artist of current track' -e'end tell'
osascript -e'tell application "iTunes"' -e'get name of current track' -e'end tell'
iTunes (Lion and older):
arch -i386 osascript -e'tell application "iTunes"' -e'get artist of current track' -e'end tell'
arch -i386 osascript -e'tell application "iTunes"' -e'get name of current track' -e'end tell'
Rythmbox:
rhythmbox-client --print-playing-format %ta
rhythmbox-client --print-playing-format %tt
MPD:
mpc -f %artist% | head -n 1
mpc -f %title% | head -n 1
Banshee:
# you can find a full Banshee script in the comments section.
banshee --query-artist
banshee --query-title
Spotify:
# you can find a full Spotify script for GNU/Linux in the comments section
In Amarok you might be able to do something like this:
qdbus org.mpris.amarok /Player GetMetadata
# match the artist and title lines
Change depending on your editor:
#!/bin/bash
artist=`osascript -e'tell application "iTunes"' -e'get artist of current track' -e'end tell'`
title=`osascript -e'tell application "iTunes"' -e'get name of current track' -e'end tell'`
curl
does the fetching for us:
curl -s --get "https://makeitpersonal.co/lyrics" --data-urlencode "artist=$artist" --data-urlencode "title=$title"
I use something like this on macOS:
#!/bin/bash
artist=`osascript -e'tell application "iTunes"' -e'get artist of current track' -e'end tell'`
title=`osascript -e'tell application "iTunes"' -e'get name of current track' -e'end tell'`
song=`curl -s --get "https://makeitpersonal.co/lyrics" --data-urlencode "artist=$artist" --data-urlencode "title=$title"`
echo -e "$artist - $title\n$song"
To try it just save, chmod
it and make sure something's playing to try it out:
chmod +x lyrics
./lyrics
If all goes well you should see the lyrics on your screen after a second or two.
If you want a cleaner output for the lyrics you can pass the song results to less
and print it
with a bit of formatting (please try it!):
echo -e "$artist_name - $song_title\n$song" | less -FX
Here we are adding an "Artist - Song" header and displaying the results as they were meant to be seen!
[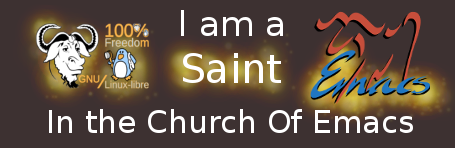](https://stallman.org/saint.html)If you want the output in a *Lyrics* buffer you can add a small function to your Emacs config:
(defun lyrics()
"Prints the lyrics for the current song"
(interactive)
;; replace path below with your own.
(let ((song (shell-command-to-string "/usr/local/bin/lyrics")))
(if (equal song "")
(message "No lyrics - Opening browser.")
(switch-to-buffer (create-file-buffer "Lyrics"))
(insert song)
(goto-line 0))))
M-x lyrics
and you're done.
- @taborj: MPD instructions and
less -FX
. - @arsinux: Banshee script.
- @Glutanimate:
--data-urlencode
forcurl
and Spotify for GNU/Linux script.