Last active
October 9, 2018 17:52
-
-
Save floooh/5f44cce30912142e726bb4ff783e24b8 to your computer and use it in GitHub Desktop.
Decode Bomb Jack tile ROMs into PNGs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#include <stdint.h> | |
#include "bombjack-roms.h" | |
#define STB_IMAGE_WRITE_IMPLEMENTATION | |
#include "stb_image_write.h" | |
#define BYTES_PER_PIXEL (3) | |
uint8_t buf[256 * 256 * BYTES_PER_PIXEL]; | |
uint8_t buf_x4[1024 * 1024 * BYTES_PER_PIXEL]; | |
void decode(int w, int h, uint8_t* rom0, uint8_t* rom1, uint8_t* rom2) { | |
for (int y = 0; y < h; y++) { | |
for (int x = 0; x < w; x++) { | |
int tile_index = y * w + x; | |
for (int yy = 0; yy < 8; yy++) { | |
uint8_t bm0 = rom0[tile_index * 8 + yy]; | |
uint8_t bm1 = rom1[tile_index * 8 + yy]; | |
uint8_t bm2 = rom2[tile_index * 8 + yy]; | |
int py = y*8 + yy; | |
int px = x*8; | |
uint8_t* ptr = buf + (py*w*8 + px) * BYTES_PER_PIXEL; | |
for (int xx=7; xx>=0; xx--) { | |
uint8_t pen = ((bm2>>xx)&1) | (((bm1>>xx)&1)<<1) | (((bm0>>xx)&1)<<2); | |
uint8_t c = pen << 5; | |
*ptr++ = c; | |
*ptr++ = c; | |
*ptr++ = c; | |
} | |
} | |
} | |
} | |
} | |
void resize() { | |
for (uint32_t y = 0; y < 1024; y++) { | |
for (uint32_t x = 0; x < 1024; x++) { | |
uint32_t x0 = x>>2; | |
uint32_t y0 = y>>2; | |
uint8_t* src = buf + (y0*256 + x0) * BYTES_PER_PIXEL; | |
uint8_t* dst = buf_x4 + (y*1024 + x) * BYTES_PER_PIXEL; | |
for (int i = 0; i < BYTES_PER_PIXEL; i++) { | |
*dst++ = *src++; | |
} | |
} | |
} | |
} | |
int main() { | |
// foreground tiles | |
decode(32, 16, dump_03_e08t, dump_04_h08t, dump_05_k08t); | |
resize(); | |
stbi_write_png("bombjack_fg.png", 1024, 512, 3, buf_x4, 1024*3); | |
// background tiles | |
decode(32, 32, dump_06_l08t, dump_07_n08t, dump_08_r08t); | |
resize(); | |
stbi_write_png("bombjack_bg.png", 1024, 1024, 3, buf_x4, 1024*3); | |
// sprite tiles | |
decode(32, 32, dump_16_m07b, dump_15_l07b, dump_14_j07b); | |
resize(); | |
stbi_write_png("bombjack_sprites.png", 1024, 1024, 3, buf_x4, 1024*3); | |
return 0; | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Generated images:
Foreground Layer Tiles:
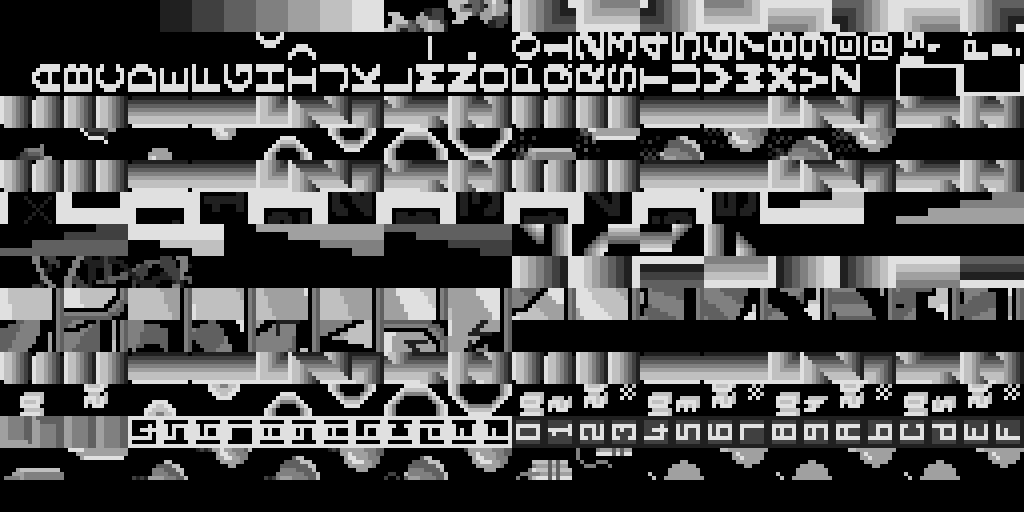
Background Layer Tiles:
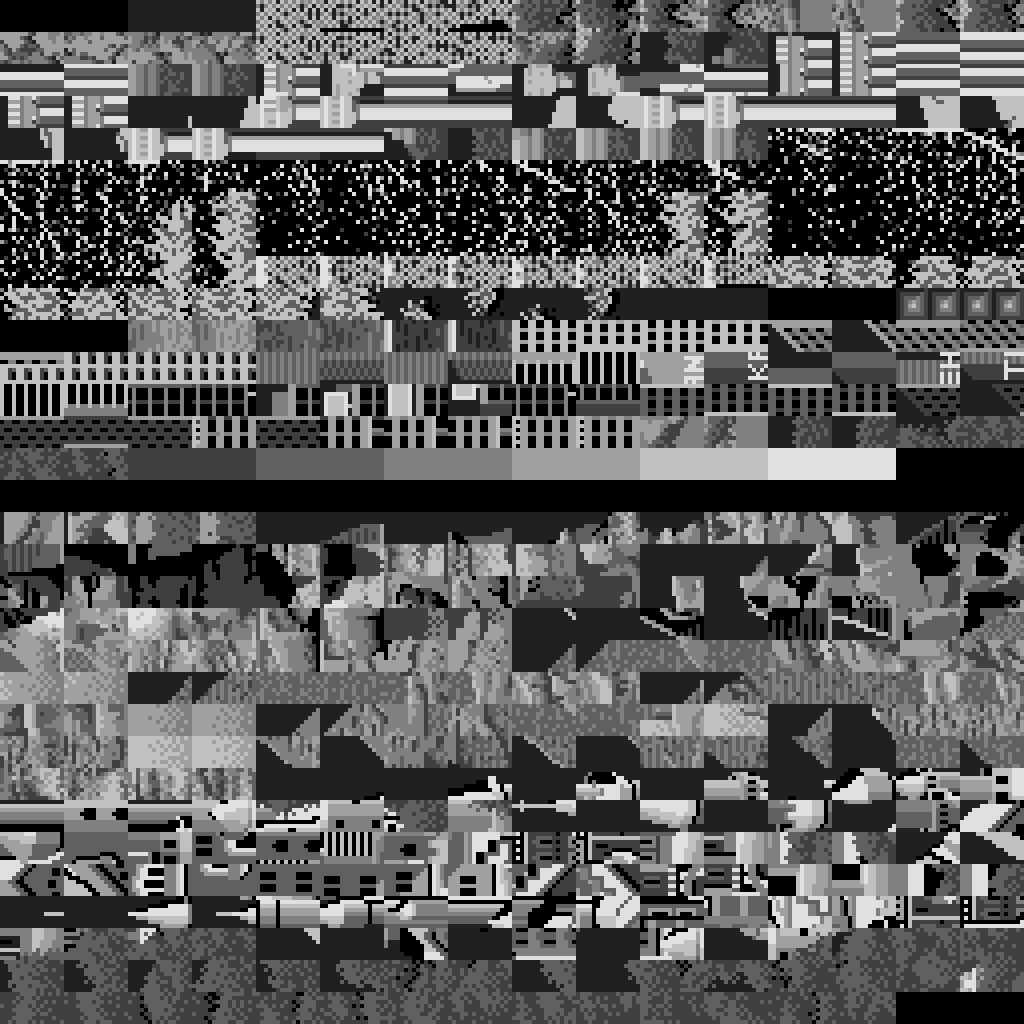
Sprite Generator Tiles:
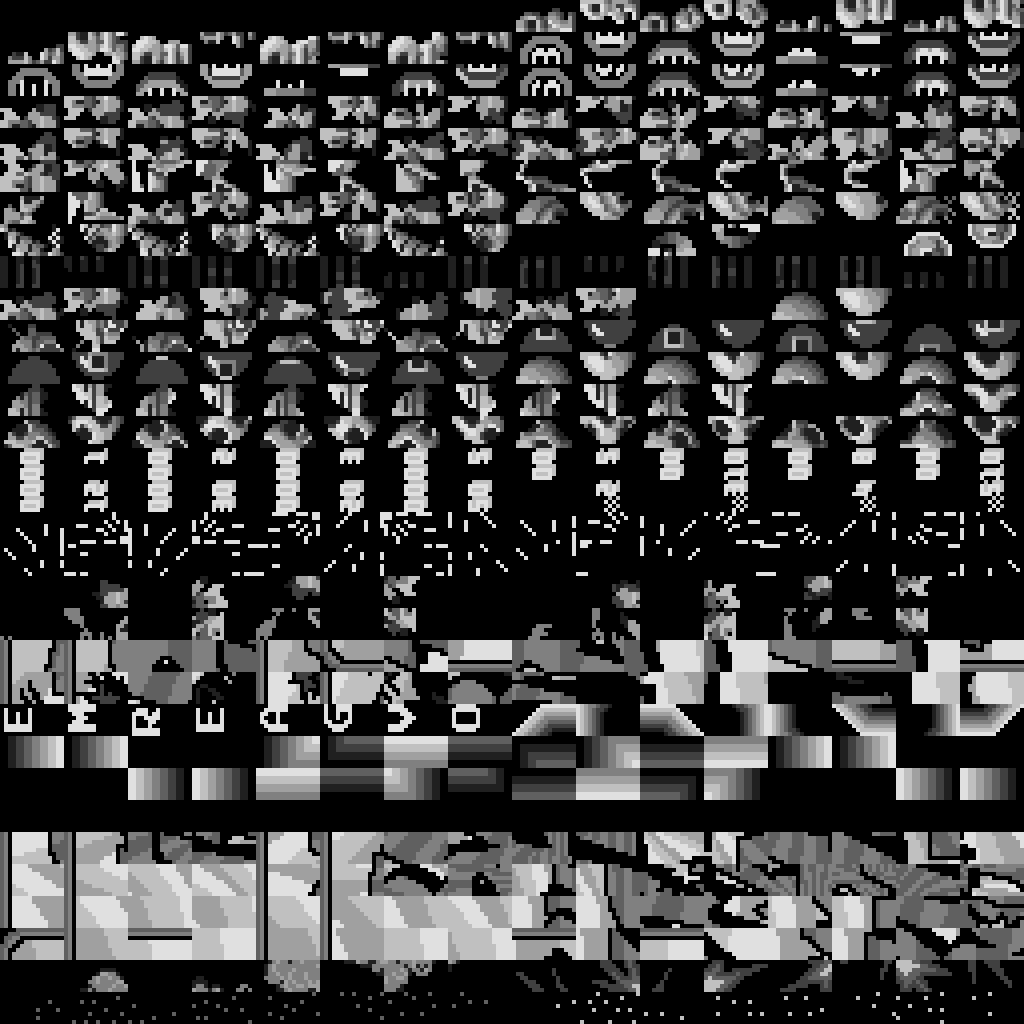