Created
January 23, 2023 01:12
-
-
Save fpigeonjr/77eb924c17e1052b1c03776b998d73a9 to your computer and use it in GitHub Desktop.
how to add variants to a Button component
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
variants ss:
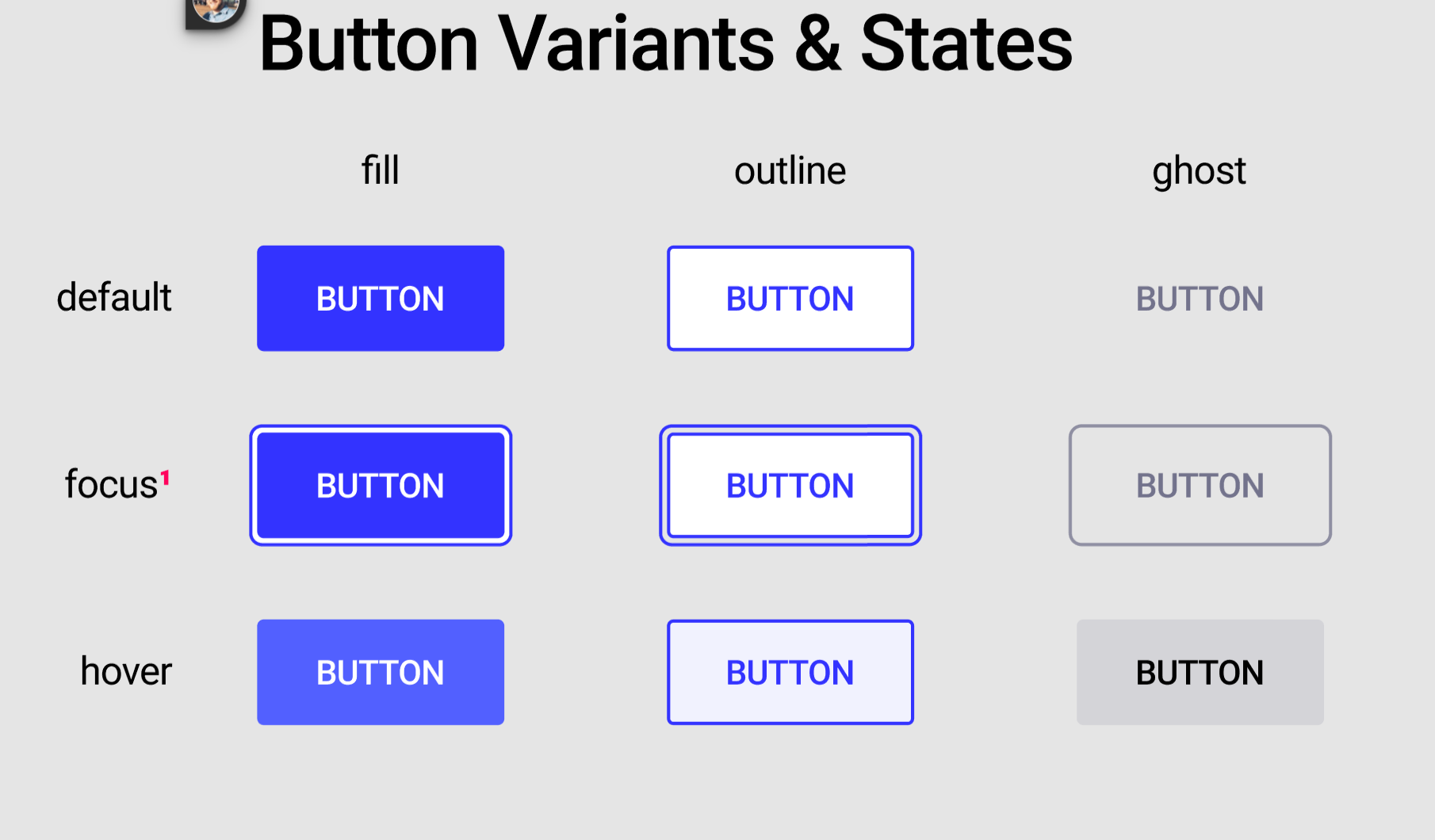