Created
January 11, 2023 17:59
-
-
Save free2bcreative/7c9befbeaf0b6155b3b83d43af968b01 to your computer and use it in GitHub Desktop.
Jetpack Compose RightModalDrawer (right-to-left)
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import androidx.compose.foundation.layout.Column | |
import androidx.compose.foundation.layout.ColumnScope | |
import androidx.compose.foundation.layout.fillMaxWidth | |
import androidx.compose.foundation.layout.padding | |
import androidx.compose.material.Button | |
import androidx.compose.material.DrawerDefaults | |
import androidx.compose.material.DrawerState | |
import androidx.compose.material.DrawerValue | |
import androidx.compose.material.MaterialTheme | |
import androidx.compose.material.ModalDrawer | |
import androidx.compose.material.Scaffold | |
import androidx.compose.material.Text | |
import androidx.compose.material.contentColorFor | |
import androidx.compose.material.rememberDrawerState | |
import androidx.compose.runtime.Composable | |
import androidx.compose.runtime.CompositionLocalProvider | |
import androidx.compose.runtime.rememberCoroutineScope | |
import androidx.compose.ui.Modifier | |
import androidx.compose.ui.graphics.Color | |
import androidx.compose.ui.graphics.Shape | |
import androidx.compose.ui.platform.LocalLayoutDirection | |
import androidx.compose.ui.tooling.preview.Preview | |
import androidx.compose.ui.unit.Dp | |
import androidx.compose.ui.unit.LayoutDirection | |
import kotlinx.coroutines.launch | |
@Composable | |
fun RightModalDrawer( | |
modifier: Modifier = Modifier, | |
drawerState: DrawerState = rememberDrawerState(DrawerValue.Closed), | |
gesturesEnabled: Boolean = true, | |
drawerShape: Shape = MaterialTheme.shapes.large, | |
drawerElevation: Dp = DrawerDefaults.Elevation, | |
drawerBackgroundColor: Color = MaterialTheme.colors.surface, | |
drawerContentColor: Color = contentColorFor(drawerBackgroundColor), | |
scrimColor: Color = DrawerDefaults.scrimColor, | |
drawerContent: @Composable ColumnScope.() -> Unit, | |
content: @Composable () -> Unit, | |
) { | |
CompositionLocalProvider(LocalLayoutDirection provides LayoutDirection.Rtl) { | |
ModalDrawer( | |
modifier = modifier, | |
drawerState = drawerState, | |
gesturesEnabled = gesturesEnabled, | |
drawerShape = drawerShape, | |
drawerElevation = drawerElevation, | |
drawerBackgroundColor = drawerBackgroundColor, | |
drawerContentColor = drawerContentColor, | |
scrimColor = scrimColor, | |
drawerContent = { | |
CompositionLocalProvider(LocalLayoutDirection provides LayoutDirection.Ltr) { | |
// under the hood, drawerContent is wrapped in a Column, but it would be under the Rtl layout | |
// so we create new column filling max width under the Ltr layout | |
Column( | |
modifier = Modifier.fillMaxWidth(), | |
content = drawerContent | |
) | |
} | |
}, | |
content = { | |
CompositionLocalProvider(LocalLayoutDirection provides LayoutDirection.Ltr) { | |
content() | |
} | |
}, | |
) | |
} | |
} | |
@Preview | |
@Composable | |
private fun RightModelDrawerPreview() { | |
val drawerState = rememberDrawerState(initialValue = DrawerValue.Open) | |
val coroutineScope = rememberCoroutineScope() | |
MaterialTheme { | |
RightModalDrawer( | |
drawerState = drawerState, | |
drawerContent = { | |
Text(text = "Model text") | |
Text(text = "Model text2") | |
} | |
) { | |
Scaffold { scaffoldPaddingValues -> | |
Column(modifier = Modifier.padding(scaffoldPaddingValues)) { | |
Text(text = "Content text") | |
Button(onClick = { coroutineScope.launch { drawerState.open() } }) { | |
Text(text = "Open Right Modal") | |
} | |
} | |
} | |
} | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Here's a screenshot of the preview:
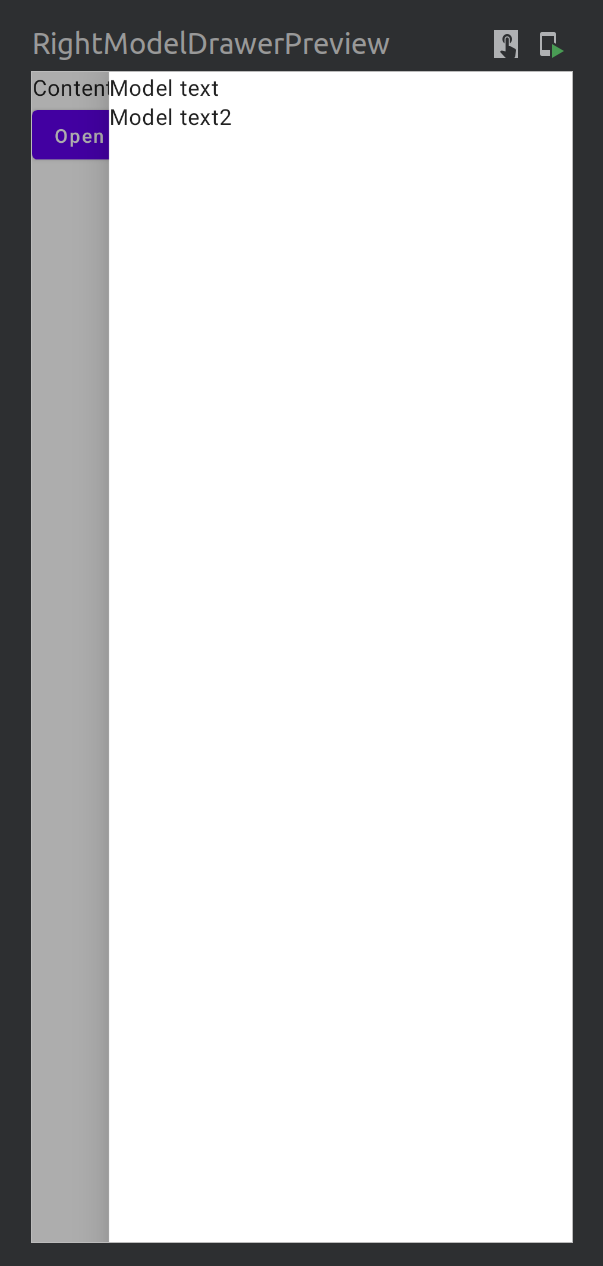