Created
April 1, 2020 13:12
-
-
Save geekykant/7b96bfd3621edcbc5042f9e44d9889fd to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/* | |
Group 4 (10,12,14,16) | |
A publishing company markets both book and CD versions of its works. Create a class | |
publication that stores the title ( type char *) and price (type float) of publication. From this | |
class derive two classes: book, which adds a page count (type int); and CD, which adds size | |
(type int). Each of these three classes should have a getdata() function to get its data from the | |
user, and a putdata() function to display its data. | |
Test the classes by creating an array of pointers to class Publication and filling it with objects | |
of both derived classes getting the data from the user. Display the details of the array using a | |
single function call in a loop. | |
*/ | |
#include<iostream> | |
#include<cstring> | |
using namespace std; | |
class Book | |
{ | |
int page_count; | |
public: | |
void getdata(){ | |
cout<<"Enter page count: "; | |
cin>>page_count; | |
} | |
void putdata(){ | |
cout<<"PageCount: "<<page_count<<endl; | |
} | |
}; | |
class CD | |
{ | |
int size; | |
public: | |
void getdata(); | |
void putdata(){ | |
cout<<"Size: "<<size<<endl; | |
} | |
}; | |
class Publication:Book,CD | |
{ | |
char* title; | |
float price; | |
public: | |
void getdata(); | |
void putdata(); | |
}; | |
void Publication::getdata(){ | |
char b_name[40]; | |
cout<<"Enter name & price of book: "; | |
cin>>b_name; | |
cin>>price; | |
title = new char[strlen(b_name)]; | |
strcpy(title, b_name); | |
Book::getdata(); | |
CD::getdata(); | |
} | |
void CD::getdata(){ | |
cout<<"Enter size of CD: "; | |
cin>>size; | |
} | |
void Publication :: putdata(){ | |
cout<<"Title: "<<title<<" Price: "<<price<<" "; | |
Book::putdata(); | |
CD::putdata(); | |
} | |
int main() | |
{ | |
Publication* pObj[25]; | |
int nCount = 0; //To keep track of how many publication instances are created. | |
while(1) | |
{ | |
cout<<"\n1.Add Publication"<<"\n2.Display All\n"<<endl; | |
int option; | |
cout<<"Enter your option: "; | |
cin>>option; | |
if(option==1){ | |
//Add new book | |
pObj[nCount] = new Publication; | |
pObj[nCount]->getdata(); | |
nCount++; | |
}else if(option == 2){ | |
//print all publications | |
for(int i=0; i<nCount;i++){ | |
pObj[i]->putdata(); | |
} | |
}else{ | |
exit(0); | |
} | |
}; | |
return 0; | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
INPUT
1
Return_Of_Devil 12.00
32
14
1
Wings_Of_Fire 90.60
144
32
2
OUPUT
Title: Return_Of_Devil Price: 12 PageCount: 32 Size: 14
Title: Wings_Of_Fire Price: 90.6 PageCount: 144 Size: 32
SCREENSHOT
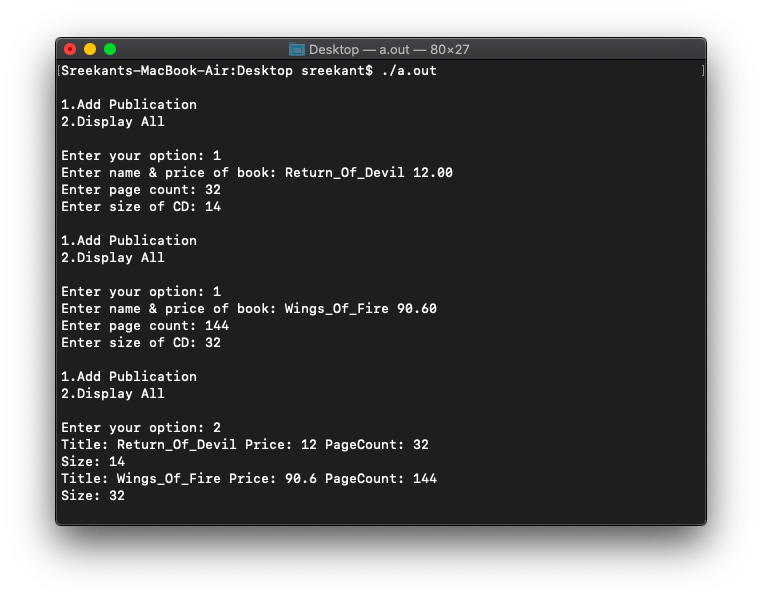