Created
April 1, 2020 05:32
-
-
Save geekykant/cb7748b8c4eeef0b91773e90e94ef609 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/* | |
Group 12 (42,44,46,48) | |
Create a class called CDriver that contains: | |
■ Data members for the name(tvpe char*) and age(tvpe int); | |
■ A constructor function that is used to intialize age and then allocates space on the heap for | |
the name; | |
■ A copy constructor function; | |
■ A print( ) function that displays the name and age; | |
■ A destructor function that releases the space on the heap; | |
Implement the following main() to implement a menu. | |
a. Add driver // Allows to create one driver instance at a time. Call Parameterized constructor | |
b. Display Drivers //Display the entire driver objects created. | |
void main() | |
{ | |
CDriver* dObj[25]; | |
int nCount = 0; //To keep track of how many driver instances are | |
//created. } | |
*/ | |
#include<iostream> | |
#include<cstring> | |
using namespace std; | |
class CDriver{ | |
char* m_name; | |
int m_age; | |
public: | |
CDriver(char name[], int age){ | |
m_name = new char[strlen(name)]; | |
strcpy(m_name, name); | |
m_age = age; | |
} | |
CDriver(CDriver &driver){ | |
m_name = driver.m_name; | |
m_age = driver.m_age; | |
} | |
void print(){ | |
cout<<"Name: "<<m_name<<" Age: "<<m_age<<endl; | |
} | |
~CDriver(){ | |
delete m_name; | |
} | |
}; | |
int main() | |
{ | |
CDriver* dObj[25]; | |
int nCount = 0; //To keep track of how many driver instances are created. | |
while(1) | |
{ | |
cout<<"\n1. Add driver"<<"\n2.Display drivers\n"<<endl; | |
int option; | |
cout<<"Enter your option: "; | |
cin>>option; | |
if(option==1){ | |
//Add new driver | |
char name[30]; | |
int age; | |
cout<<"Enter name and Age: "; | |
cin>>name; | |
cin>>age; | |
dObj[nCount] = new CDriver(name,age); | |
nCount++; | |
}else if(option == 2){ | |
//print all drivers | |
for(int i=0; i<nCount;i++){ | |
dObj[i]->print(); | |
} | |
}else{ | |
exit(0); | |
} | |
}; | |
return 0; | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
INPUT
1
Sreekant 21
1
Sangeedh 22
2
6
OUPUT
Name: Sreekant Age: 21
Name: Sangeedh Age: 22
PROGRAM
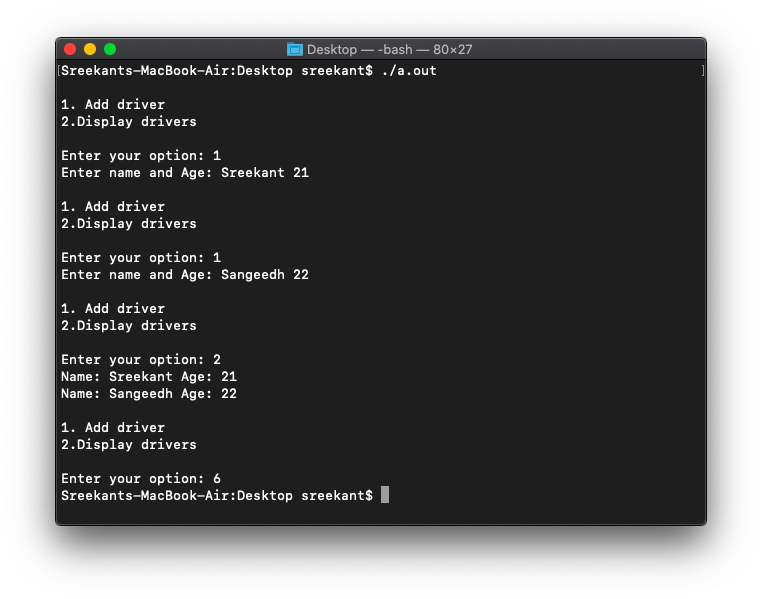