Sleep tracker with a timer, in the terminal. Demo
Download, chmod +x stat-sleep.fish
and put it in your PATH
fish
is required to run this script, but doesn't have to be your SHELL
to do it.
Can suggest a time to go to bed or a time to set alarm, given the tracked
sleeping hours average. See --bed HH:MM
and --wake HH:MM
Healthy sleep average is keyed to 7.5 hours, time for bed defaults to 22:00
and time for alarm defaults to 05:45
Usage: stat-sleep.fish [HOURS_SLEPT]
Indicate running average of hours slept, or update it.
stat-sleep.fish 6.5 # add a day's sleep
stat-sleep.fish +2 # correct this day's number
stat-sleep.fish -t # start sleep timer
# Ctrl-C to abort
set -e fish_sleep_hrs # to reset all
stat-sleep.fish --wake 05:45
# suggest a time to turn in for 7.5 average
# plus the 15 minutes to fall aseleep
stat-sleep.fish --bed 22:00 --verbose
# time for alarm, same as above
License MIT, free to do whatever. Yours to fuck up.
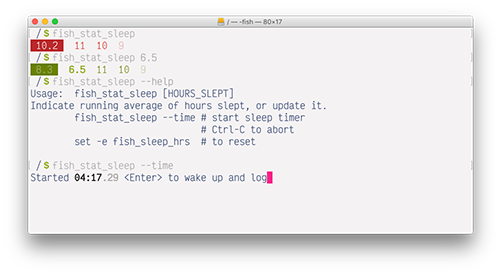
asciinema.org/a/453741