Last active
October 18, 2022 06:31
-
-
Save gusakovgiorgi/302ba656006f16294bfb6d6f6eae67bd to your computer and use it in GitHub Desktop.
Composable BubbleEmitter
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import androidx.compose.animation.core.Animatable | |
import androidx.compose.animation.core.Easing | |
import androidx.compose.animation.core.LinearEasing | |
import androidx.compose.animation.core.tween | |
import androidx.compose.foundation.Canvas | |
import androidx.compose.foundation.layout.BoxWithConstraints | |
import androidx.compose.foundation.layout.fillMaxSize | |
import androidx.compose.foundation.layout.offset | |
import androidx.compose.material.Text | |
import androidx.compose.runtime.Composable | |
import androidx.compose.runtime.LaunchedEffect | |
import androidx.compose.runtime.remember | |
import androidx.compose.ui.Modifier | |
import androidx.compose.ui.geometry.Offset | |
import androidx.compose.ui.graphics.Color | |
import androidx.compose.ui.layout.onSizeChanged | |
import androidx.compose.ui.text.font.FontWeight | |
import androidx.compose.ui.tooling.preview.Preview | |
import androidx.compose.ui.unit.IntOffset | |
import androidx.compose.ui.unit.IntSize | |
import java.lang.Math.abs | |
import kotlin.properties.Delegates | |
import kotlin.random.Random | |
@Preview(showBackground = true) | |
@Composable | |
fun DefaultPreview() { | |
BubbleEmitter {requiredModifier-> | |
Text( | |
// This modifier must be applied in order to move composable inside a bubble | |
modifier = requiredModifier, | |
text = "+2", | |
color = Color.White, | |
fontWeight = FontWeight.Bold | |
) | |
} | |
} | |
@Composable | |
fun BubbleEmitter( | |
modifier: Modifier = Modifier.fillMaxSize(), | |
size: Int = 300, | |
durationMillis: Int = 4000, | |
animationEasing: Easing = LinearEasing, | |
backgroundColor: Color = Color.DarkGray.copy(alpha = 0.8f), | |
glossColor: Color = Color.LightGray.copy(alpha = 0.6f), | |
insideComposable: @Composable (appliedModifier: Modifier) -> Unit | |
) { | |
BoxWithConstraints(modifier) { | |
val bubble = remember { | |
val radius = abs(size) / 2f | |
Bubble(radius) | |
} | |
val calcEngine = remember { | |
CalculationsEngine( | |
constraints.maxWidth.toFloat(), constraints.maxHeight.toFloat(), bubble | |
) | |
} | |
val yOffset = remember { | |
Animatable(0f) | |
} | |
// Set new offset that recalculates the bubble object | |
calcEngine.setNewOffset(yOffset.value) | |
LaunchedEffect(Unit) { | |
yOffset.animateTo( | |
constraints.maxHeight.toFloat(), | |
tween(easing = animationEasing, durationMillis = durationMillis) | |
) | |
} | |
Canvas(modifier = Modifier.fillMaxSize()) { | |
drawCircle(backgroundColor, bubble.radius, Offset(bubble.x, bubble.y)) | |
drawCircle( | |
glossColor, | |
bubble.radius / 4, | |
Offset(bubble.x + bubble.radius / 2.5F, bubble.y - bubble.radius / 2.5f) | |
) | |
} | |
// Size of composable. We need this to center the composable inside the bubble | |
var detectedSize: IntSize? = null | |
insideComposable(Modifier | |
.onSizeChanged { | |
detectedSize = it | |
} | |
.offset { | |
IntOffset( | |
bubble.x.toInt() - (detectedSize?.width ?: 50) / 2, | |
bubble.y.toInt() - (detectedSize?.height ?: 50) / 2 | |
) | |
}) | |
} | |
} | |
class CalculationsEngine( | |
private val width: Float, private val height: Float, private val bubble: Bubble | |
) { | |
private var initialY by Delegates.notNull<Float>() | |
private var movingDirection = getRandomMovingDirection() | |
init { | |
val bubbleWidth = bubble.radius * 2; | |
bubble.x = Random.nextInt(bubbleWidth.toInt(), (width - bubbleWidth).toInt()).toFloat() | |
bubble.y = height - 5 | |
initialY = bubble.y | |
movingDirection = getRandomMovingDirection() | |
} | |
fun setNewOffset(yOffsetFromInitial: Float) { | |
bubble.y = initialY - yOffsetFromInitial | |
val xOffsetFromCurrent: Float = when (movingDirection) { | |
MovingDirection.POSITIVE -> { | |
2f | |
} | |
MovingDirection.NEGATIVE -> { | |
-2f | |
} | |
MovingDirection.NEUTRAL -> { | |
0f | |
} | |
} | |
val potentialOffset = bubble.x + xOffsetFromCurrent | |
bubble.x = if (potentialOffset >= (width - bubble.radius)) width - bubble.radius | |
else if (potentialOffset <= bubble.radius) bubble.radius | |
else potentialOffset | |
} | |
} | |
private const val NO_VALUE = -1f | |
enum class MovingDirection { | |
NEGATIVE, NEUTRAL, POSITIVE | |
} | |
class Bubble(val radius: Float, var x: Float = NO_VALUE, var y: Float = NO_VALUE) | |
fun getRandomMovingDirection() = when ((0..1).random()) { | |
0 -> MovingDirection.NEGATIVE | |
1 -> MovingDirection.POSITIVE | |
else -> error("incorrect value were generated") | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Result:
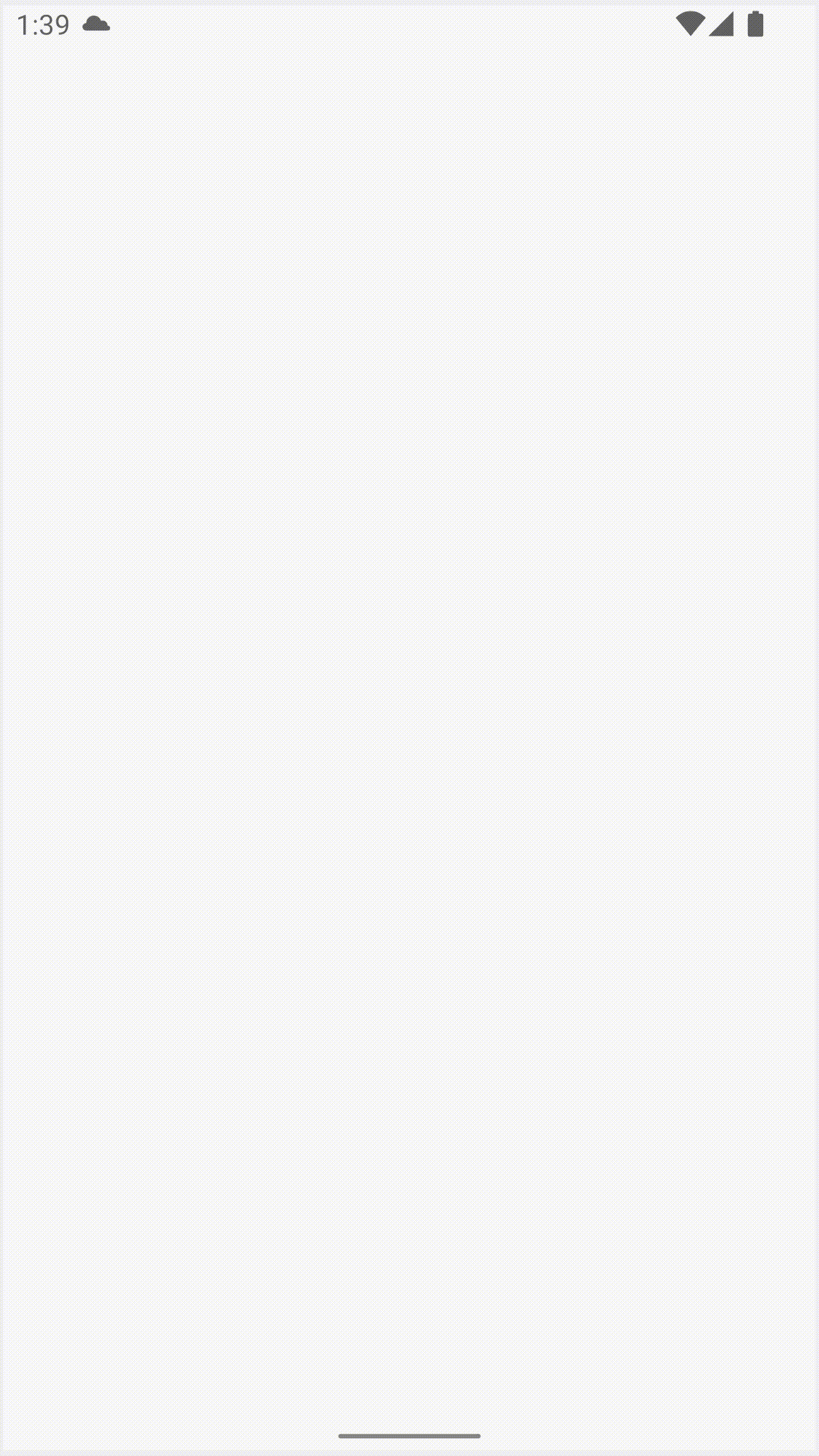