Last active
March 14, 2019 02:53
-
-
Save hubertgrzeskowiak/5afc0d27562c0fc27cb09e2b5819b08a to your computer and use it in GitHub Desktop.
Script for removing unused local branches
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/usr/bin/env bash | |
# Cleaner for local branches that have no remotes any more. | |
# Not yet pushed local branches are untouched. | |
# | |
# For convencience, add to ~/.gitconfig as: | |
# | |
# [alias] | |
# cleanup-branches = !~/bin/git-cleanup-branches.sh | |
# | |
set -eu | |
set -o pipefail | |
red=$(tput setaf 1; tput bold) | |
green=$(tput setaf 2; tput bold) | |
norm=$(tput sgr0) | |
ask_delete_local_branches() { | |
local branches=( "$@" ) | |
echo | |
echo "These branches are going to be ${red}DELETED${norm} locally. Continue? [yN]" | |
local answer | |
read answer | |
if [[ "${answer}" =~ ^(y|Y)$ ]]; then | |
git branch -D ${branches[@]} | |
echo "${green}✓ Done!${norm}" | |
echo | |
else | |
echo "${red}▩ Aborting${norm}" | |
fi | |
} | |
stale_local_branches() { | |
local -a branches | |
#echo "🔎 Searching for stale local branches." | |
readarray branches < <(git branch -vv | grep ': gone] ' | grep -v '^* ' | cut -d " " -f 3) | |
if [[ "${#branches[@]}" -eq 0 ]]; then | |
echo "${green}✓ No stale local branches found. All good.${norm}" | |
return | |
fi | |
# Remove newlines from entries | |
branches=("${branches[@]//$'\n'/}") | |
# Remove spaces from entries | |
branches=("${branches[@]// /}") | |
echo "These branches have no remotes any more:" | |
printf " - %s\n" "${branches[@]}" | |
ask_delete_local_branches "${branches[@]}" | |
} | |
# This assumes a release-process that involves "release-" prefixed shared branches. | |
stale_release_branches() { | |
local -a branches | |
#echo "🔎 Searching for stale release branches." | |
# Shellcheck disable=SC2063 | |
readarray branches < <(git branch | sed 's/ //g' | grep -v '^* ' | grep '^release-') | |
if [[ "${#branches[@]}" -eq 0 ]]; then | |
echo "${green}✓ No stale release branches found. All good.${norm}" | |
return | |
fi | |
# Remove newlines from entries | |
branches=("${branches[@]//$'\n'/}") | |
# Remove spaces from entries | |
branches=("${branches[@]// /}") | |
echo "You have the following local release branches:" | |
printf " - %s\n" "${branches[@]}" | |
ask_delete_local_branches "${branches[@]}" | |
} | |
stale_local_branches | |
stale_release_branches |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Example output
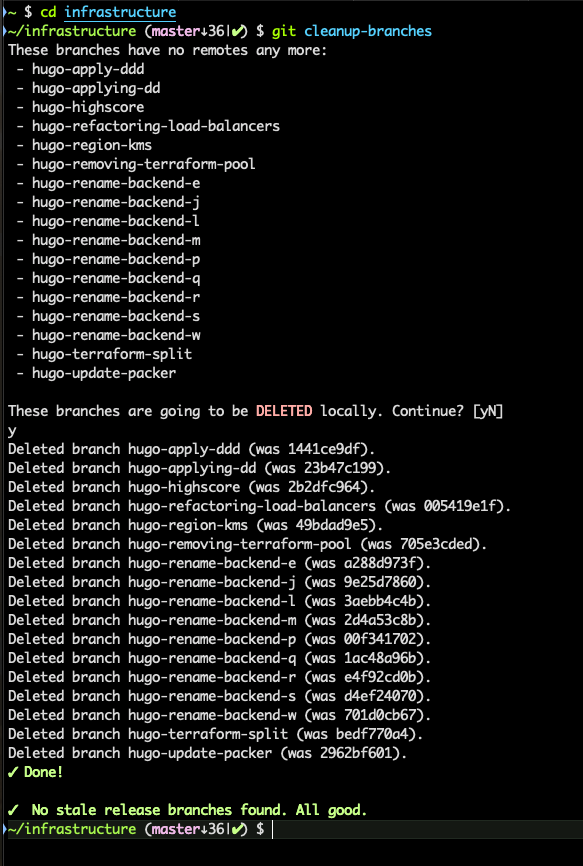