-
-
Save iconifyit/2cbab3f0dd421b6d4bb520bfcf445f0d to your computer and use it in GitHub Desktop.
function getExportOptionsSVG() { | |
var exportOptions = new ExportOptionsSVG(); | |
/** | |
* A range of artboards to save, if saveMultipleArtboards is true. A comma-delimited list of artboard names., or the empty string to save all artboards. Default: empty String. | |
* {string} | |
* | |
* NOTE: Set to an empty string to export all. Be sure `saveMultipleArtboards` is set to true to use this. | |
*/ | |
exportOptions.artboardRange = '1,2,4,7,10'; // | |
/** | |
* If true, the exported file should be compressed. Default: false. | |
* {boolean} | |
*/ | |
exportOptions.compressed = true; | |
/** | |
* The decimal precision for element coordinate values. Range: 1 to 7. Default: 3. | |
* {number} | |
*/ | |
exportOptions.coordinatePrecision = 3; | |
/** | |
* How the CSS properties of the document should be included in the exported file. Default: SVGCSSPropertyLocation.STYLEATTRIBUTES. | |
* {SVGCSSPropertyLocation} | |
* | |
* Possible values: | |
* exportOptions.cssProperties = SVGCSSPropertyLocation.PRESENTATIONATTRIBUTES; | |
* exportOptions.cssProperties = SVGCSSPropertyLocation.ENTITIES; | |
* exportOptions.cssProperties = SVGCSSPropertyLocation.STYLEATTRIBUTES; | |
* exportOptions.cssProperties = SVGCSSPropertyLocation.STYLEELEMENTS; | |
*/ | |
exportOptions.cssProperties = SVGCSSPropertyLocation.PRESENTATIONATTRIBUTES; | |
/** | |
* How the text in the document should be encoded. Default: SVGDocumentEncoding.ASCII. | |
* {SVGDocumentEncoding} | |
*/ | |
exportOptions.documentEncoding = SVGDocumentEncoding.UTF8; | |
/** | |
* The SVG version to which the file should conform. Default: SVGDTDVersion.SVG1_1. | |
* {SVGDTDVersion} | |
* | |
* Possible values: | |
* exportOptions.DTD = SVGDTDVersion.SVG1_0; | |
* exportOptions.DTD = SVGDTDVersion.SVG1_1; | |
* exportOptions.DTD = SVGDTDVersion.SVGBASIC1_1; | |
* exportOptions.DTD = SVGDTDVersion.SVGTINY1_1; | |
* exportOptions.DTD = SVGDTDVersion.SVGTINY1_1PLUS; | |
* exportOptions.DTD = SVGDTDVersion.SVGTINY1_2; | |
*/ | |
exportOptions.DTD = SVGDTDVersion.SVG1_1; | |
/** | |
* If true, the raster images contained in the document should be embedded in the exported file. Default: false. | |
* {boolean} | |
*/ | |
exportOptions.embedRasterImages = true; | |
/** | |
* Which font glyphs should be included in the exported file. Default: SVGFontSubsetting.ALLGLYPHS. | |
* {SVGFontSubsetting} | |
* | |
* Possible values: | |
* exportOptions.fontSubsetting = SVGFontSubsetting.ALLGLYPHS; | |
* exportOptions.fontSubsetting = SVGFontSubsetting.COMMONENGLISH; | |
* exportOptions.fontSubsetting = SVGFontSubsetting.COMMONROMAN; | |
* exportOptions.fontSubsetting = SVGFontSubsetting.GLYPHSUSED; | |
* exportOptions.fontSubsetting = SVGFontSubsetting.GLYPHSUSEDPLUSENGLISH; | |
* exportOptions.fontSubsetting = SVGFontSubsetting.GLYPHSUSEDPLUSROMAN; | |
* exportOptions.fontSubsetting = SVGFontSubsetting.None; | |
*/ | |
exportOptions.fontSubsetting = SVGFontSubsetting.ALLGLYPHS; | |
/** | |
* The type of font to included in the exported file. Default: SVGFontType.CEFFONT. | |
* {SVGFontType} | |
* | |
* Possible values: | |
* exportOptions.fontType = SVGFontType.CEFFONT; | |
* exportOptions.fontType = SVGFontType.OUTLINEFONT; | |
* exportOptions.fontType = SVGFontType.SVGFONT; | |
*/ | |
exportOptions.fontType = SVGFontType.SVGFONT; | |
/** | |
* If true, file information should be saved in the exported file. Default: false. | |
* {boolean} | |
*/ | |
exportOptions.includeFileInfo = true; | |
/** | |
* If true, save unused styles in the exported file. Default: false. | |
* {boolean} | |
*/ | |
exportOptions.includeUnusedStyles = true; | |
/** | |
* If true, variables and datasets should be saved in the exported file. Default: false. | |
* {boolean} | |
*/ | |
exportOptions.includeVariablesAndDatasets = true; | |
/** | |
* If true, the exported file should be optimized for the SVG Viewer. Default: false. | |
* {boolean} | |
*/ | |
exportOptions.optimizeForSVGViewer = true; | |
/** | |
* If true, Illustrator editing capabilities should be preserved when exporting the document. Default: false. | |
* {boolean} | |
*/ | |
exportOptions.preserveEditability = true; | |
/** | |
* If true, save the artboards specified by artboardRange in the exported file. Default: false. | |
* {boolean} | |
*/ | |
exportOptions.saveMultipleArtboards = true; | |
/** | |
* If true, slice data should be exported with the file. Default: false. | |
* {boolean} | |
*/ | |
exportOptions.slices = true; | |
/** | |
* If true, SVG automatic kerning is allowed in the file. Default: false. | |
* {boolean} | |
*/ | |
exportOptions.sVGAutoKerning = true; | |
/** | |
* If true, the SVG text-on-path construct is allowed in the file. Default: false. | |
* {boolean} | |
*/ | |
exportOptions.sVGTextOnPath = true; | |
/** | |
* The class name of the referenced object. | |
* {ExportType} | |
* | |
* Possible values: | |
* exportOptions.typename = ExportType.AUTOCAD; | |
* exportOptions.typename = ExportType.FLASH; | |
* exportOptions.typename = ExportType.GIF; | |
* exportOptions.typename = ExportType.ILLUSTRATORPATHS; | |
* exportOptions.typename = ExportType.JPEG; | |
* exportOptions.typename = ExportType.PHOTOSHOP; | |
* exportOptions.typename = ExportType.PNG8; | |
* exportOptions.typename = ExportType.PNG24; | |
* exportOptions.typename = ExportType.SAVEFORWEB; | |
* exportOptions.typename = ExportType.SVG; | |
* exportOptions.typename = ExportType.TIFF; | |
*/ | |
exportOptions.typename = ExportType.SVG; | |
} | |
function exportFileToSVG(theTargetFilePath) { | |
if (app.documents.length > 0) { | |
app.activeDocument.exportFile( | |
new File(theTargetFilePath), /* Export file path */ | |
ExportType.SVG, /* Export type */ | |
getExportOptionsSVG() /* Export options */ | |
); | |
} | |
} |
Hi Peter. You are very welcome. I'm happy to share whatever code and knowledge I can. I looked through every piece of documentation I could find and I cannot find any mention about setTypeOfSVG
in any documentation except for the editor. I suspect this may be a documentation error. I have the document ref files for the entire SVG codebase, which is nothing special, just the header files that are publicly available. They have no mention of this property. I'm sorry I don't have better news but this looks like bad documentation probably a carryover from an older version. It could also be from the AppleScript object model. Theoretically they should be the same but are not necessarily. I will keep looking but at the moment I don't have anything helpful.
Thanks for this. Do you know anything about the SetTypeOfSVG property? It supposedly targets the way object IDs are written, but I cant find how to write it. here it is in the Script Editor library for Illustrator
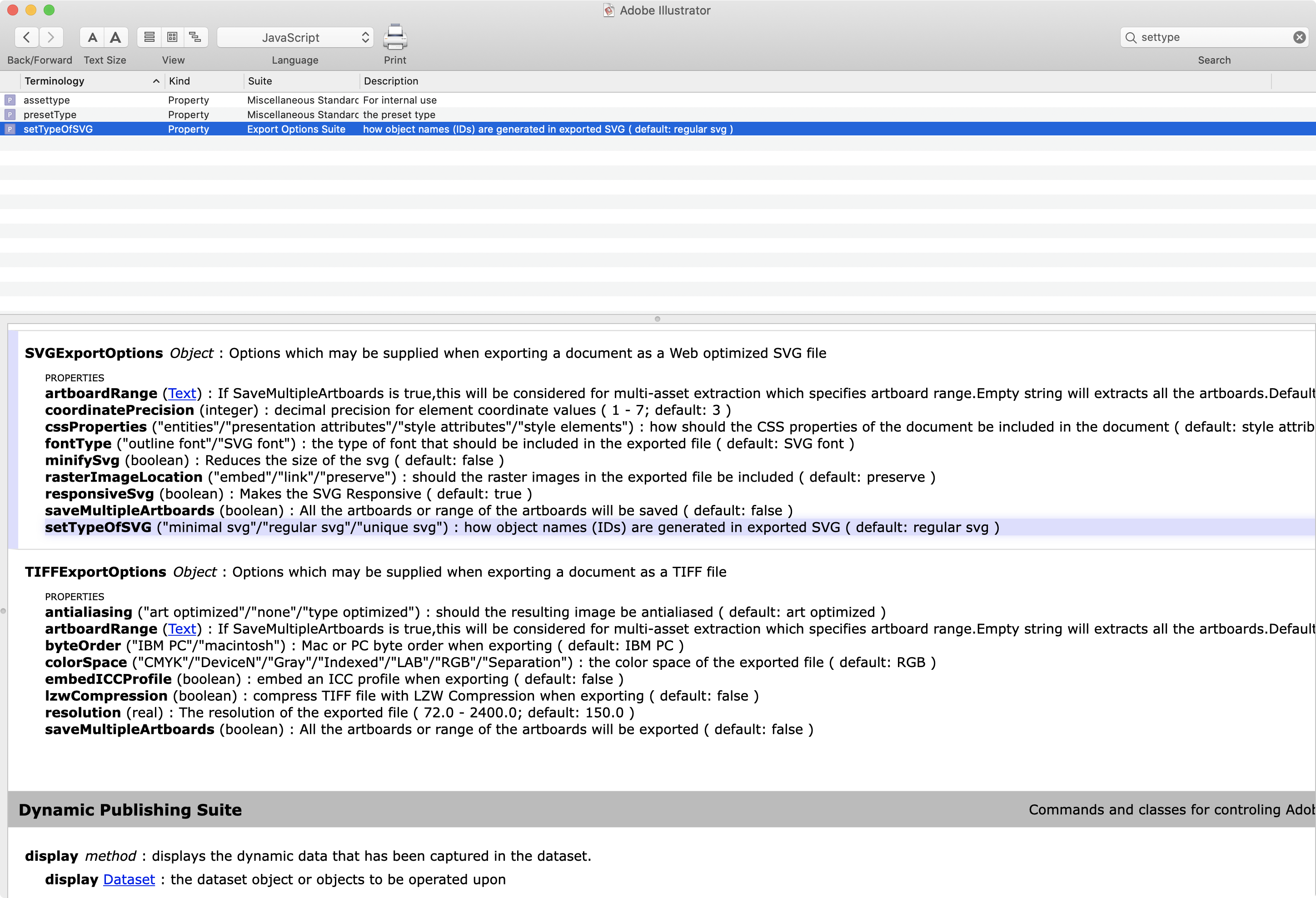