Created
June 29, 2017 18:33
-
-
Save indexzero/6261ad9292c78cf3c5aa69265e2422bf to your computer and use it in GitHub Desktop.
Generate a 24 hour range of 30-minute time intervals that are i18n compatible
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
let items = []; | |
for (var hour = 0; hour < 24; hour++) { | |
items.push([hour, 0]); | |
items.push([hour, 30]); | |
} | |
const date = new Date(); | |
const formatter = new Intl.DateTimeFormat('en-US', { | |
hour: 'numeric', | |
minute: 'numeric', | |
hour12: false | |
}); | |
const range = items.map(time => { | |
const [hour, minute] = time; | |
date.setHours(hour); | |
date.setMinutes(minute); | |
return formatter.format(date); | |
}); | |
console.dir(range); |
For lazy people like me:
0: "24:00"
1: "24:30"
2: "01:00"
3: "01:30"
4: "02:00"
5: "02:30"
6: "03:00"
7: "03:30"
8: "04:00"
9: "04:30"
10: "05:00"
11: "05:30"
12: "06:00"
13: "06:30"
14: "07:00"
15: "07:30"
16: "08:00"
17: "08:30"
18: "09:00"
19: "09:30"
20: "10:00"
21: "10:30"
22: "11:00"
23: "11:30"
24: "12:00"
25: "12:30"
26: "13:00"
27: "13:30"
28: "14:00"
29: "14:30"
30: "15:00"
31: "15:30"
32: "16:00"
33: "16:30"
34: "17:00"
35: "17:30"
36: "18:00"
37: "18:30"
38: "19:00"
39: "19:30"
40: "20:00"
41: "20:30"
42: "21:00"
43: "21:30"
44: "22:00"
45: "22:30"
46: "23:00"
47: "23:30"
having the option to use start & end time and generate the series between that would be nice.
const generateHoursInterval = (
startHourInMinute,
endHourInMinute,
interval,
) => {
const times = [];
for (let i = 0; startHourInMinute < 24 * 60; i++) {
if (startHourInMinute > endHourInMinute) break;
var hh = Math.floor(startHourInMinute / 60); // getting hours of day in 0-24 format
var mm = startHourInMinute % 60; // getting minutes of the hour in 0-55 format
times[i] = ('0' + (hh % 24)).slice(-2) + ':' + ('0' + mm).slice(-2);
startHourInMinute = startHourInMinute + interval;
}
return times;
};
const interval = 30; //minutes interval
const startDate = 60 * 7; // start time in minutes
const endDate = 60 * 21; // end time in minutes
const foo = generateHoursInterval(startDate, endDate, interval);
console.log(foo);
// ["07:00", "07:30", "08:00", "08:30", "09:00", "09:30", "10:00", "10:30", "11:00", "11:30", "12:00", "12:30", "13:00", "13:30", "14:00", "14:30", "15:00", "15:30", "16:00", "16:30", "17:00", "17:30", "18:00", "18:30", "19:00", "19:30", "20:00", "20:30", "21:00"]
@ridvanaltun +1
Awesome, very useful
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
mmm
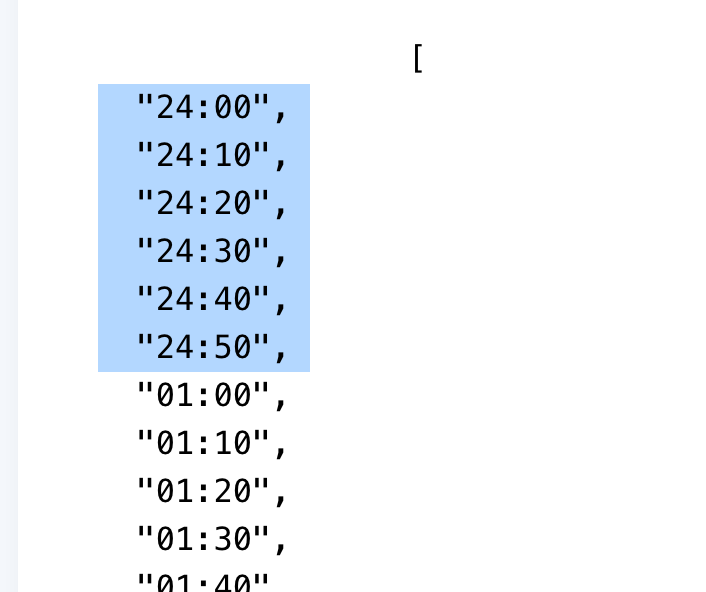