Last active
April 12, 2018 07:29
-
-
Save irsalshabirin/962eda980fd8719190f9f351043fef67 to your computer and use it in GitHub Desktop.
to create overlap recyclerView items
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/* | |
* Copyright (c) 2018, irsalshabirin | |
* | |
* Licensed under the Apache License, Version 2.0 (the | |
* "License"); you may not use this file except in compliance | |
* with the License. You may obtain a copy of the License at: | |
* | |
* http://www.apache.org/licenses/LICENSE-2.0 | |
* | |
* Unless required by applicable law or agreed to in writing, | |
* software distributed under the License is distributed on | |
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY | |
* KIND, either express or implied. See the License for the | |
* specific language governing permissions and limitations | |
* under the License. | |
*/ | |
/** | |
* Created by irsalshabirin on 23/3/18. | |
*/ | |
class OverlapItemDecoration extends RecyclerView.ItemDecoration { | |
private int mHorizontalSpace = 0; | |
private int mVerticalSpace = 0; | |
/** | |
* @param mHorizontalSpace the value can be either positive or negative | |
* @param mVerticalSpace the value can be either positive or negative | |
*/ | |
public OverlapItemDecoration(int mHorizontalSpace, int mVerticalSpace) { | |
this.mHorizontalSpace = mHorizontalSpace; | |
this.mVerticalSpace = mVerticalSpace; | |
} | |
@Override | |
public void getItemOffsets(Rect outRect, View view, RecyclerView parent, RecyclerView.State state) { | |
int position = parent.getChildAdapterPosition(view); | |
boolean reverseLayout = false; | |
if (parent.getLayoutManager() instanceof LinearLayoutManager) { | |
reverseLayout = ((LinearLayoutManager) parent.getLayoutManager()).getReverseLayout(); | |
} else { | |
return; | |
} | |
if (position != 0) { | |
if (reverseLayout) { | |
// mVerticalSpace must negative value | |
// top left | |
outRect.set( | |
((position) * mHorizontalSpace), | |
0, | |
0, | |
(mVerticalSpace + Math.abs(mHorizontalSpace)) | |
); | |
// top right | |
outRect.set( | |
((position) * Math.abs(mHorizontalSpace)), | |
0, | |
0, | |
(mVerticalSpace + Math.abs(mHorizontalSpace)) | |
); | |
} else { | |
// mVerticalSpace must negative value | |
// TODO: 26/03/18 bottom left | |
// bottom right | |
outRect.set( | |
((position) * Math.abs(mHorizontalSpace)), | |
(mVerticalSpace + Math.abs(mHorizontalSpace)), | |
0, | |
0 | |
); | |
} | |
} | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
example use :
recyclerView.addItemDecoration(new OverlapItemDecoration(-12, -100));
result :
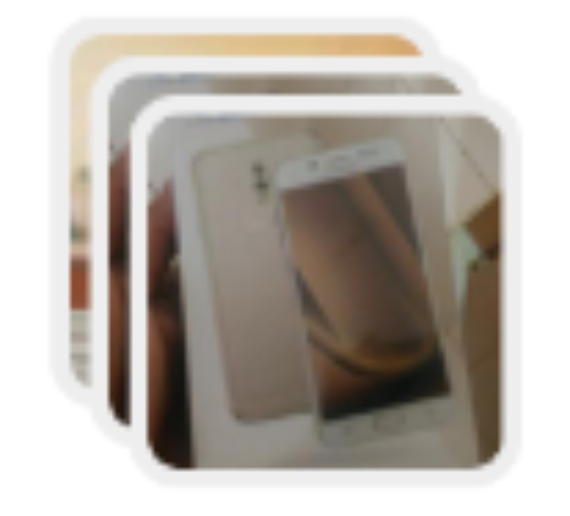