Created
January 11, 2023 13:17
-
-
Save isuke01/ebaebb3c5fc5c4804fdad3982973ba50 to your computer and use it in GitHub Desktop.
Test Extend Core paragraph with footnote (just attribute)
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/** | |
* WordPress dependencies | |
*/ | |
import { __ } from '@wordpress/i18n'; | |
import { PanelBody, PanelRow, TextControl } from '@wordpress/components'; | |
// eslint-disable-next-line import/no-extraneous-dependencies | |
import { InspectorControls } from '@wordpress/block-editor'; | |
function InspectorControl({ attributes, setAttributes }) { | |
const { footnote } = attributes; | |
return ( | |
<InspectorControls> | |
<PanelBody title={__('Extra settings', 'norskpensjon')}> | |
<PanelRow> | |
<TextControl | |
label={__('Footnote', 'norskpensjon')} | |
value={footnote} | |
onChange={(value) => | |
setAttributes({ footnote: value }) | |
} | |
help={__( | |
'Add footnote', | |
'norskpensjon', | |
)} | |
/> | |
</PanelRow> | |
</PanelBody> | |
</InspectorControls> | |
); | |
} | |
export default InspectorControl; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/* eslint-disable import/no-unresolved */ | |
/** | |
* WordPress dependencies | |
*/ | |
import { __ } from '@wordpress/i18n'; | |
import { addFilter } from '@wordpress/hooks'; | |
// eslint-disable-next-line import/no-extraneous-dependencies | |
import { createHigherOrderComponent } from '@wordpress/compose'; | |
// eslint-disable-next-line import/no-extraneous-dependencies | |
import TokenList from '@wordpress/token-list'; | |
/** | |
* Internal dependencies | |
*/ | |
import InspectorControl from './core-media-text.backend.inspector-control'; | |
/** | |
* Add extra class, maybe to indicate in backedn that has the footnote? | |
*/ | |
function addSaveProps(props, blockType, attributes) { | |
if (blockType.name !== 'core/paragraph') { | |
return props; | |
} | |
const { footnote } = attributes; | |
// Use TokenList to dedupe classes. | |
const classes = new TokenList(props.className); | |
if (footnote) { | |
classes.add('has-footnote'); | |
} | |
props.className = classes?.value || undefined; | |
return props; | |
} | |
addFilter( | |
'blocks.getSaveContent.extraProps', | |
'core/paragraph/addSaveProps', | |
addSaveProps, | |
); | |
/** | |
* Filters registered block settings to expand the block edit wrapper | |
* by applying the desired styles and classnames. | |
* | |
* @param {Object} settings Original block settings. | |
* @return {Object} Filtered block settings. | |
*/ | |
function addEditProps(settings) { | |
const existingGetEditWrapperProps = settings.getEditWrapperProps; | |
if (settings.name !== 'core/paragraph') { | |
return settings; | |
} | |
return Object.assign( {}, settings, { | |
attributes: Object.assign( {}, settings.attributes, { | |
footnote: { type: 'string' } | |
} ), | |
} ); | |
} | |
addFilter( | |
'blocks.registerBlockType', | |
'core/paragraph/addEditProps', | |
addEditProps, | |
); | |
/** | |
* Override the default edit UI to include new inspector controls for | |
* all the custom styles configs. | |
* | |
* @param {Function} BlockEdit Original component. | |
* @return {Function} Wrapped component. | |
*/ | |
export const withInspectorControls = createHigherOrderComponent( | |
(BlockEdit) => (props) => { | |
const { name: blockName, isSelected } = props; | |
// eslint-disable-next-line prettier/prettier | |
const canShowControll = 'core/paragraph' === blockName && isSelected; | |
return ( | |
<> | |
{canShowControll && <InspectorControl {...props} />} | |
<BlockEdit {...props} /> | |
</> | |
); | |
}, | |
'withToolbarControls', | |
); | |
addFilter( | |
'editor.BlockEdit', | |
'core/paragraph/with-block-controls', | |
withInspectorControls, | |
); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?php | |
// override tempalte becasue I have no idea how to do it in the block editor hooks :D | |
add_filter( 'render_block', __NAMESPACE__ . '\\override_core_paragraph', 10, 2 ); | |
function override_core_paragraph( string $block_content, array $block ) { | |
if ( $block['blockName'] === 'core/paragraph' ) : | |
// update the $block_content here | |
if( isset( $block['attrs']['footnote'] ) ) { | |
// do something | |
$original_content = $block_content; | |
$block_content = '<div>'; | |
$block_content .= $original_content; | |
$block_content .= '<em>' . $block['attrs']['footnote'] . '</em>'; | |
$block_content .= '</div>'; | |
} | |
endif; | |
return $block_content; | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
IT goint to add inspecotr block with text field
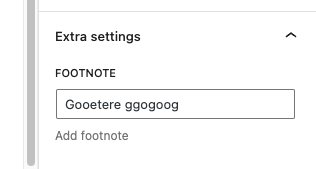
Also you can add button to quick edit element maybe with popup or open sidebar and focus input ... :) https://mariecomet.fr/en/2021/12/14/adding-options-controls-existing-gutenberg-block/