Rotate puzzle
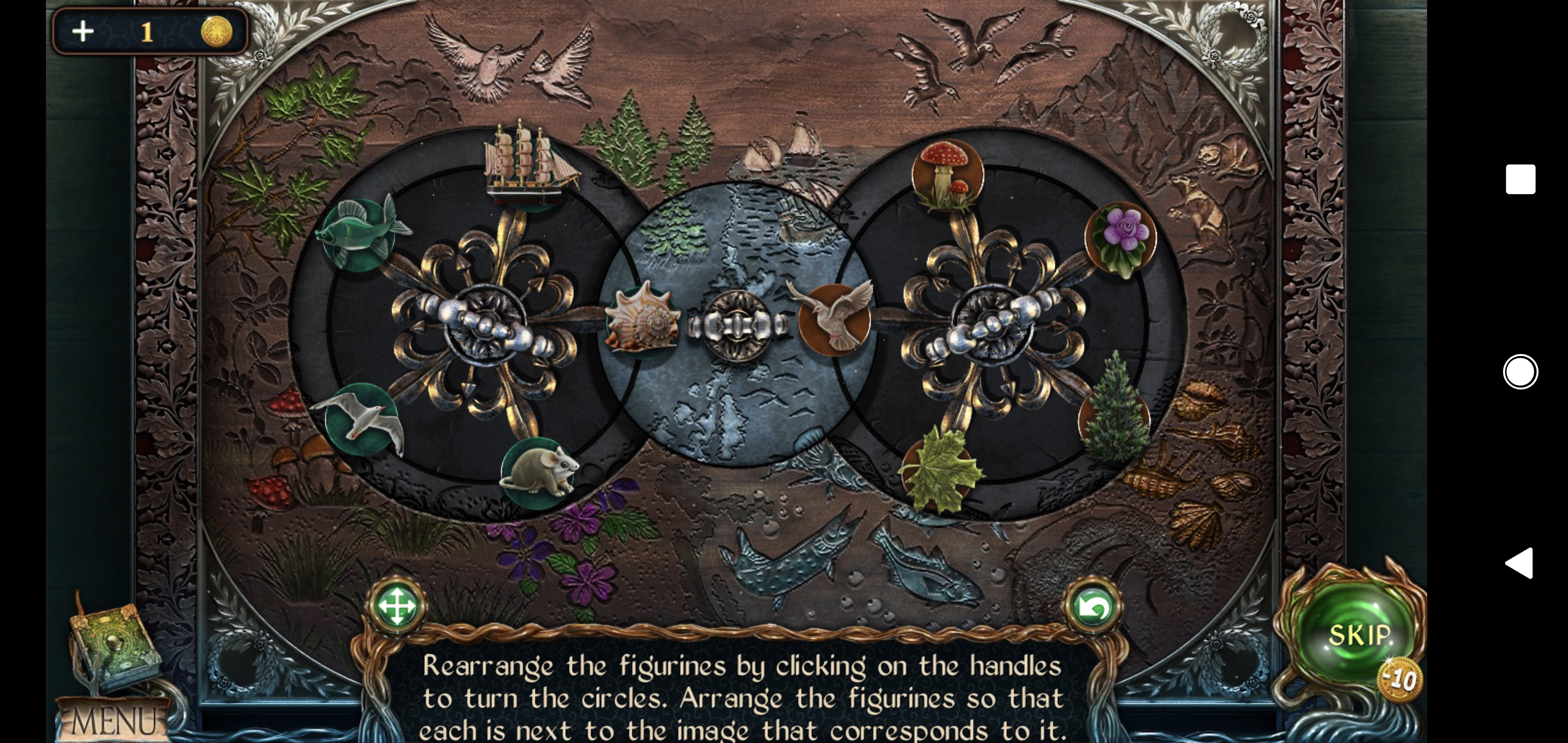
'''
Init None
swap (('snail', 'boat', 'fish', 'seagull', 'mouse'), ('dove', 'maple', 'pine', 'flower', 'mushroom'))
rotate right (('dove', 'boat', 'fish', 'seagull', 'mouse'), ('snail', 'maple', 'pine', 'flower', 'mushroom'))
rotate right (('dove', 'boat', 'fish', 'seagull', 'mouse'), ('mushroom', 'snail', 'maple', 'pine', 'flower'))
swap (('dove', 'boat', 'fish', 'seagull', 'mouse'), ('flower', 'mushroom', 'snail', 'maple', 'pine'))
rotate right (('flower', 'boat', 'fish', 'seagull', 'mouse'), ('dove', 'mushroom', 'snail', 'maple', 'pine'))
swap (('flower', 'boat', 'fish', 'seagull', 'mouse'), ('pine', 'dove', 'mushroom', 'snail', 'maple'))
rotate left (('pine', 'boat', 'fish', 'seagull', 'mouse'), ('flower', 'dove', 'mushroom', 'snail', 'maple'))
swap (('mouse', 'pine', 'boat', 'fish', 'seagull'), ('flower', 'dove', 'mushroom', 'snail', 'maple'))
rotate left (('flower', 'pine', 'boat', 'fish', 'seagull'), ('mouse', 'dove', 'mushroom', 'snail', 'maple'))
swap (('seagull', 'flower', 'pine', 'boat', 'fish'), ('mouse', 'dove', 'mushroom', 'snail', 'maple'))
rotate right (('mouse', 'flower', 'pine', 'boat', 'fish'), ('seagull', 'dove', 'mushroom', 'snail', 'maple'))
swap (('mouse', 'flower', 'pine', 'boat', 'fish'), ('maple', 'seagull', 'dove', 'mushroom', 'snail'))
rotate right (('maple', 'flower', 'pine', 'boat', 'fish'), ('mouse', 'seagull', 'dove', 'mushroom', 'snail'))
rotate right (('maple', 'flower', 'pine', 'boat', 'fish'), ('snail', 'mouse', 'seagull', 'dove', 'mushroom'))
swap (('maple', 'flower', 'pine', 'boat', 'fish'), ('mushroom', 'snail', 'mouse', 'seagull', 'dove'))
rotate left (('mushroom', 'flower', 'pine', 'boat', 'fish'), ('maple', 'snail', 'mouse', 'seagull', 'dove'))
swap (('fish', 'mushroom', 'flower', 'pine', 'boat'), ('maple', 'snail', 'mouse', 'seagull', 'dove'))
rotate left (('maple', 'mushroom', 'flower', 'pine', 'boat'), ('fish', 'snail', 'mouse', 'seagull', 'dove'))
rotate right (('boat', 'maple', 'mushroom', 'flower', 'pine'), ('fish', 'snail', 'mouse', 'seagull', 'dove'))
swap (('boat', 'maple', 'mushroom', 'flower', 'pine'), ('dove', 'fish', 'snail', 'mouse', 'seagull'))
rotate left (('dove', 'maple', 'mushroom', 'flower', 'pine'), ('boat', 'fish', 'snail', 'mouse', 'seagull'))
Finished (('pine', 'dove', 'maple', 'mushroom', 'flower'), ('boat', 'fish', 'snail', 'mouse', 'seagull'))
Solved in 23 steps
'''
if __name__ == "__main__":
rp = RotaryPuzzle(left_clockwise=True, right_clockwise=True)
init_left = ('owl', 'moon', 'mushroom', 'snail', 'wolf', 'snake', 'spider', 'mouse')
init_right = ('pine', 'moose', 'lotus', 'rabit', 'bear', 'frog', 'maple', 'butterfly')
goal_left = ('rabit', 'butterfly', 'owl', 'maple', 'moose', 'frog', 'lotus', 'bear')
goal_right = ('snake', 'wolf', 'mushroom', 'mouse', 'spider', 'pine', 'moon', 'snail')
solved = rp.solve(
(init_left, init_right),
(goal_left, goal_right)
)
rp.show_steps(solved, (goal_left, goal_right))
#### Ooops to slow to complete, need optimize
Re arrange puzzle
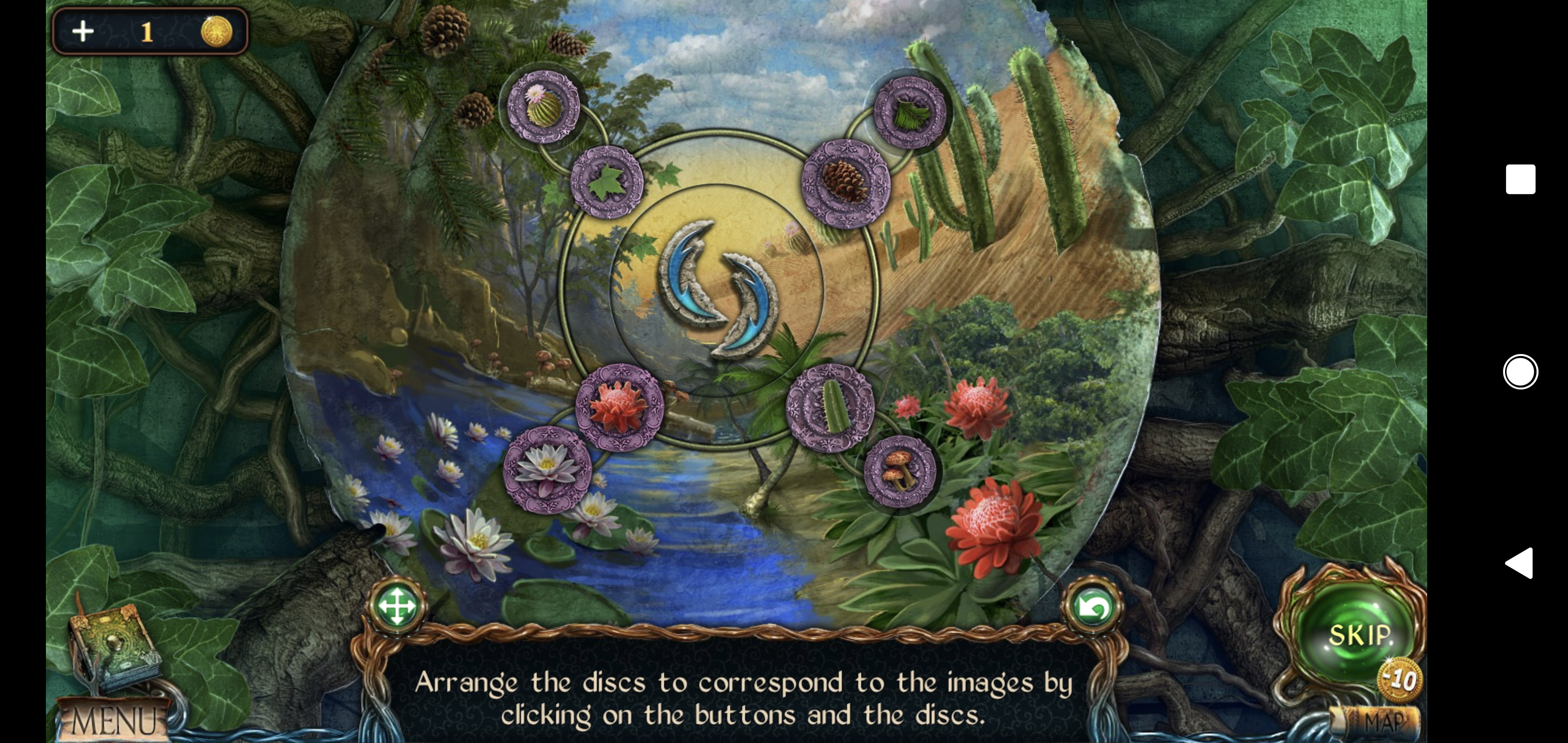
if __name__ == "__main__":
rap = RotaryArrangePuzzle()
init = (
('mapple', 'cactus flower'),
('pine', 'coconut leaf'),
('long cactus', 'mushroom'),
('red flower', 'white flower')
)
goal = (
('mapple', 'pine'),
('cactus flower', 'long cactus'),
('coconut leaf', 'red flower'),
('mushroom', 'white flower')
)
solved = rap.solve(init, goal)
rap.show_steps(solved, goal)
'''
Init None
rotate left (anticlockwise) (('mapple', 'cactus flower'), ('pine', 'coconut leaf'), ('long cactus', 'mushroom'), ('red flower', 'white flower'))
swap topleft (('pine', 'cactus flower'), ('long cactus', 'coconut leaf'), ('red flower', 'mushroom'), ('mapple', 'white flower'))
swap topright (('cactus flower', 'pine'), ('long cactus', 'coconut leaf'), ('red flower', 'mushroom'), ('mapple', 'white flower'))
swap lowerright (('cactus flower', 'pine'), ('coconut leaf', 'long cactus'), ('red flower', 'mushroom'), ('mapple', 'white flower'))
rotate right (clockwise) (('cactus flower', 'pine'), ('coconut leaf', 'long cactus'), ('mushroom', 'red flower'), ('mapple', 'white flower'))
Finished (('mapple', 'pine'), ('cactus flower', 'long cactus'), ('coconut leaf', 'red flower'), ('mushroom', 'white flower'))
Solved in 7 steps
'''
Sliding puzzle
Goal
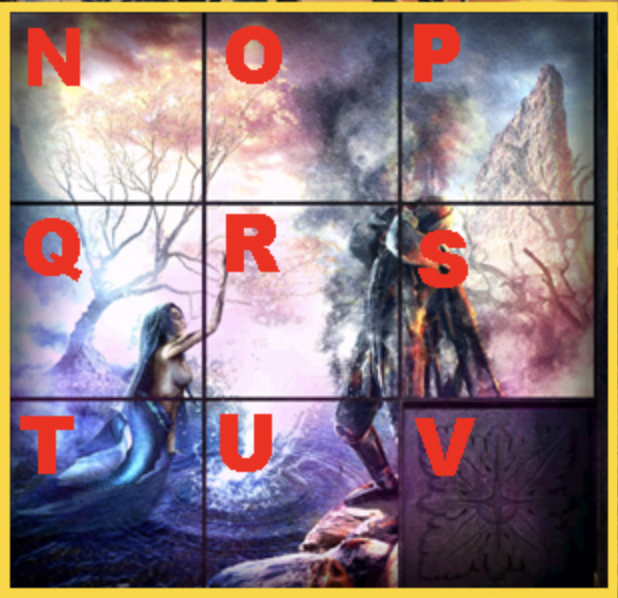
if __name__ == "__main__":
sp = SlidingPuzzle('x')
init = "SPOTxNRQU"
goal = "NOPQRSTUx"
solved = sp.solve(init, goal)
sp.show_steps(solved, goal)
'''
Init None
Down SPOTxNRQU
Right SxOTPNRQU
Up xSOTPNRQU
Left TSOxPNRQU
Left TSOPxNRQU
Down TSOPNxRQU
Right TSxPNORQU
Up TxSPNORQU
Right TNSPxORQU
Down TNSxPORQU
Left xNSTPORQU
Up NxSTPORQU
Left NPSTxORQU
Down NPSTOxRQU
Right NPxTOSRQU
Up NxPTOSRQU
Up NOPTxSRQU
Right NOPTQSRxU
Down NOPTQSxRU
Left NOPxQSTRU
Up NOPQxSTRU
Left NOPQRSTxU
Finished NOPQRSTUx
Solved in 24 steps
'''
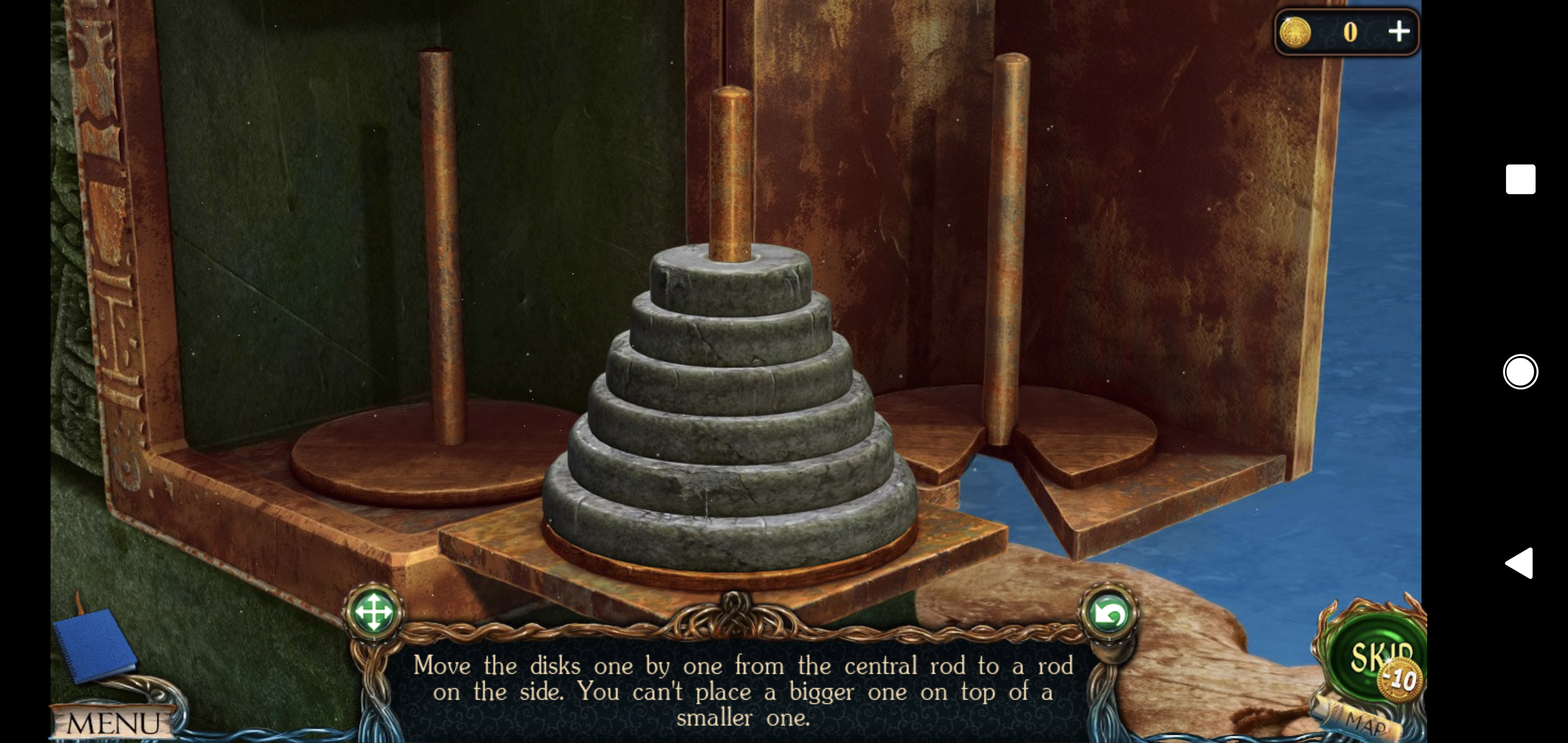
if __name__ == "__main__":
hanoi = TowerOfHanoi()
initial = (
(),
(6, 5, 4, 3, 2, 1),
()
)
goal = (
(6, 5, 4, 3, 2, 1),
(),
()
)
solved = hanoi.solve(initial, goal)
hanoi.show_steps(solved, goal)
'''
Init None
Move 1 to () ((), (6, 5, 4, 3, 2, 1), ())
Move 1 to () ((), (6, 5, 4, 3, 2), (1,))
Move 2 to (2,) ((2,), (6, 5, 4, 3), (1,))
Move 1 to () ((2, 1), (6, 5, 4, 3), ())
Move 0 to (6, 5, 4) ((2, 1), (6, 5, 4), (3,))
Move 0 to (3,) ((2,), (6, 5, 4, 1), (3,))
Move 1 to (3, 2) ((), (6, 5, 4, 1), (3, 2))
Move 1 to () ((), (6, 5, 4), (3, 2, 1))
Move 2 to (4,) ((4,), (6, 5), (3, 2, 1))
Move 2 to (6, 5) ((4, 1), (6, 5), (3, 2))
Move 0 to (6, 5, 2) ((4, 1), (6, 5, 2), (3,))
Move 2 to (4,) ((4,), (6, 5, 2, 1), (3,))
Move 1 to () ((4, 3), (6, 5, 2, 1), ())
Move 1 to (4, 3) ((4, 3), (6, 5, 2), (1,))
Move 2 to (4, 3, 2) ((4, 3, 2), (6, 5), (1,))
Move 1 to () ((4, 3, 2, 1), (6, 5), ())
Move 0 to (6,) ((4, 3, 2, 1), (6,), (5,))
Move 0 to (5,) ((4, 3, 2), (6, 1), (5,))
Move 1 to (5, 2) ((4, 3), (6, 1), (5, 2))
Move 0 to (6,) ((4, 3), (6,), (5, 2, 1))
Move 2 to (4,) ((4,), (6, 3), (5, 2, 1))
Move 2 to (6, 3) ((4, 1), (6, 3), (5, 2))
Move 0 to (6, 3, 2) ((4, 1), (6, 3, 2), (5,))
Move 0 to (5,) ((4,), (6, 3, 2, 1), (5,))
Move 1 to (5, 4) ((), (6, 3, 2, 1), (5, 4))
Move 1 to () ((), (6, 3, 2), (5, 4, 1))
Move 2 to (2,) ((2,), (6, 3), (5, 4, 1))
Move 1 to (5, 4) ((2, 1), (6, 3), (5, 4))
Move 0 to (6,) ((2, 1), (6,), (5, 4, 3))
Move 0 to (5, 4, 3) ((2,), (6, 1), (5, 4, 3))
Move 1 to (5, 4, 3, 2) ((), (6, 1), (5, 4, 3, 2))
Move 1 to () ((), (6,), (5, 4, 3, 2, 1))
Move 2 to (6,) ((6,), (), (5, 4, 3, 2, 1))
Move 2 to () ((6, 1), (), (5, 4, 3, 2))
Move 0 to (2,) ((6, 1), (2,), (5, 4, 3))
Move 2 to (6,) ((6,), (2, 1), (5, 4, 3))
Move 1 to (5, 4) ((6, 3), (2, 1), (5, 4))
Move 1 to (6, 3) ((6, 3), (2,), (5, 4, 1))
Move 2 to (6, 3, 2) ((6, 3, 2), (), (5, 4, 1))
Move 2 to () ((6, 3, 2, 1), (), (5, 4))
Move 0 to (4,) ((6, 3, 2, 1), (4,), (5,))
Move 0 to (5,) ((6, 3, 2), (4, 1), (5,))
Move 1 to (5, 2) ((6, 3), (4, 1), (5, 2))
Move 0 to (4,) ((6, 3), (4,), (5, 2, 1))
Move 2 to (6,) ((6,), (4, 3), (5, 2, 1))
Move 2 to (4, 3) ((6, 1), (4, 3), (5, 2))
Move 0 to (4, 3, 2) ((6, 1), (4, 3, 2), (5,))
Move 2 to (6,) ((6,), (4, 3, 2, 1), (5,))
Move 1 to () ((6, 5), (4, 3, 2, 1), ())
Move 1 to (6, 5) ((6, 5), (4, 3, 2), (1,))
Move 2 to (6, 5, 2) ((6, 5, 2), (4, 3), (1,))
Move 1 to () ((6, 5, 2, 1), (4, 3), ())
Move 0 to (4,) ((6, 5, 2, 1), (4,), (3,))
Move 0 to (3,) ((6, 5, 2), (4, 1), (3,))
Move 1 to (3, 2) ((6, 5), (4, 1), (3, 2))
Move 1 to (6, 5) ((6, 5), (4,), (3, 2, 1))
Move 2 to (6, 5, 4) ((6, 5, 4), (), (3, 2, 1))
Move 2 to () ((6, 5, 4, 1), (), (3, 2))
Move 0 to (2,) ((6, 5, 4, 1), (2,), (3,))
Move 2 to (6, 5, 4) ((6, 5, 4), (2, 1), (3,))
Move 1 to () ((6, 5, 4, 3), (2, 1), ())
Move 1 to (6, 5, 4, 3) ((6, 5, 4, 3), (2,), (1,))
Move 2 to (6, 5, 4, 3, 2) ((6, 5, 4, 3, 2), (), (1,))
Finished ((6, 5, 4, 3, 2, 1), (), ())
Solved in 65 steps
'''
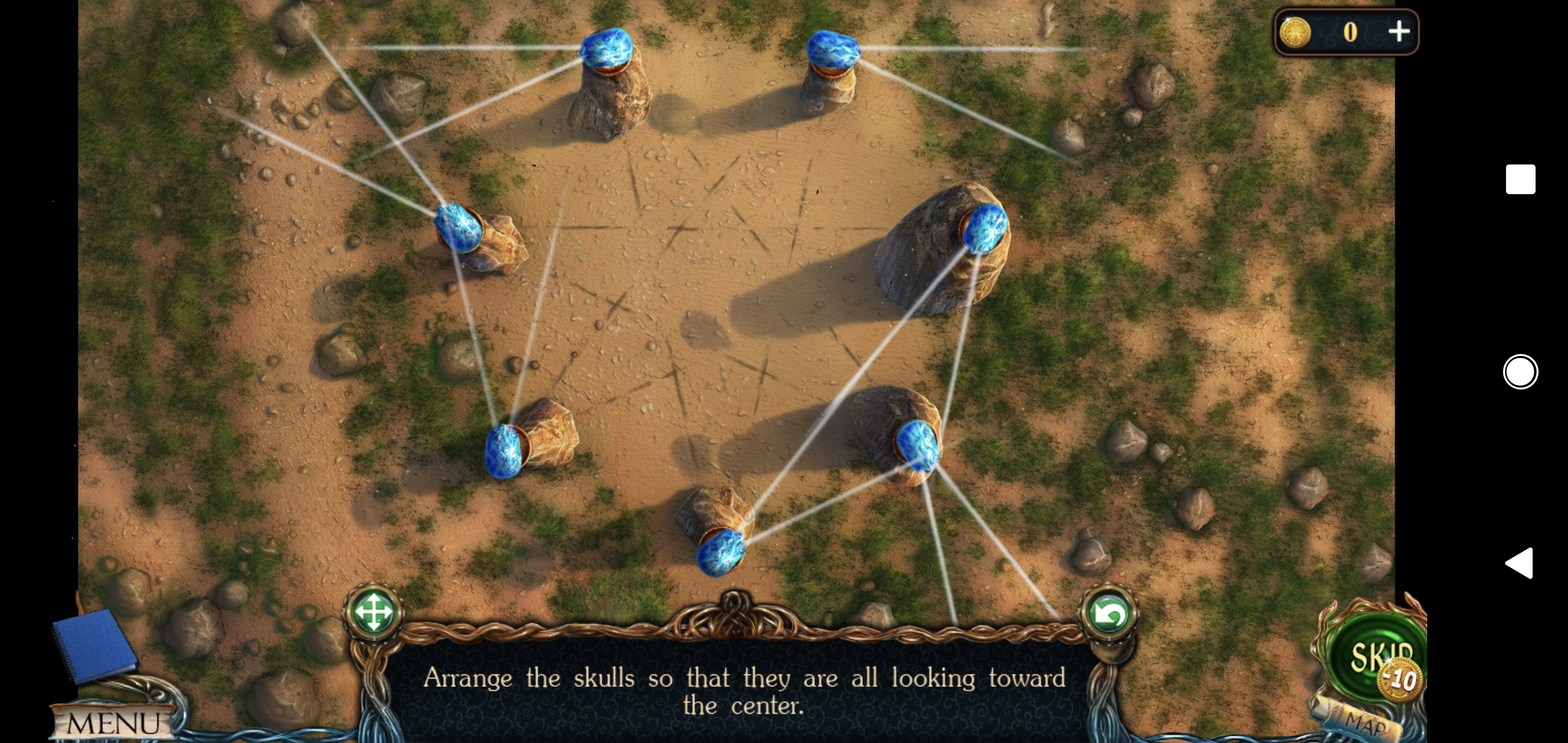
if __name__ == "__main__":
star = SevenPointStar()
init = "3721654"
goal = "1234567"
solved = star.solve(init, goal)
star.show_steps(solved, goal)
'''
Init None
Swap 0 3 3721654
Swap 1 5 1723654
Swap 1 4 1523674
Swap 2 5 1623574
Swap 1 5 1673524
Swap 3 6 1273564
Swap 2 6 1274563
Finished 1234567
Solved in 9 steps
'''
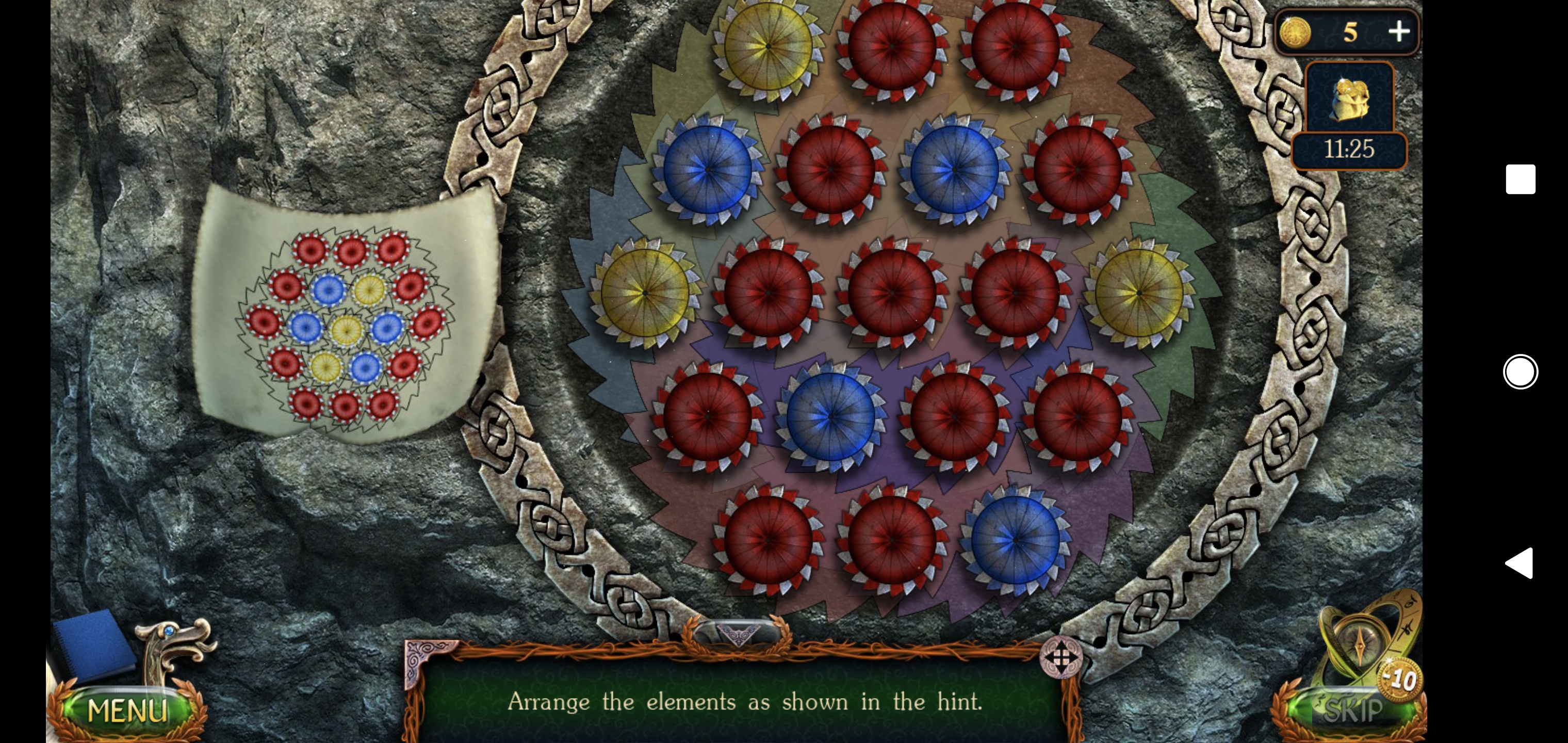
if __name__ == "__main__":
r = RotateRBY()
init = "YRRBRBRYRRRYRBRRRRB"
goal = "RRRRBYRRBYBRRYBRRRR"
solved = r.solve(init, goal)
r.show_steps(solved, goal)
'''
Init None
Click 8 YRRBRBRYRRRYRBRRRRB
Click 4 YRRYBBRRRRRYBRRRRRB
Click 13 YYRRBRRRRBRYBRRRRRB
Click 10 YYRRBRRRBRRYRRBRRRB
Click 14 YYRRBRRRBBRRRRRYRRB
Click 9 YYRRBRRRBRBRRRRRRBY
Click 14 YYRRBBRRRRRRRRBRRBY
Click 4 YYRRBBRRRRRRRBBRRYR
Click 14 RYRRBYRRRBRRRBBRRYR
Click 4 RYRRBYRRRBBRRYBRRRR
Finished RRRRBYRRBYBRRYBRRRR
Solved in 12 steps
'''
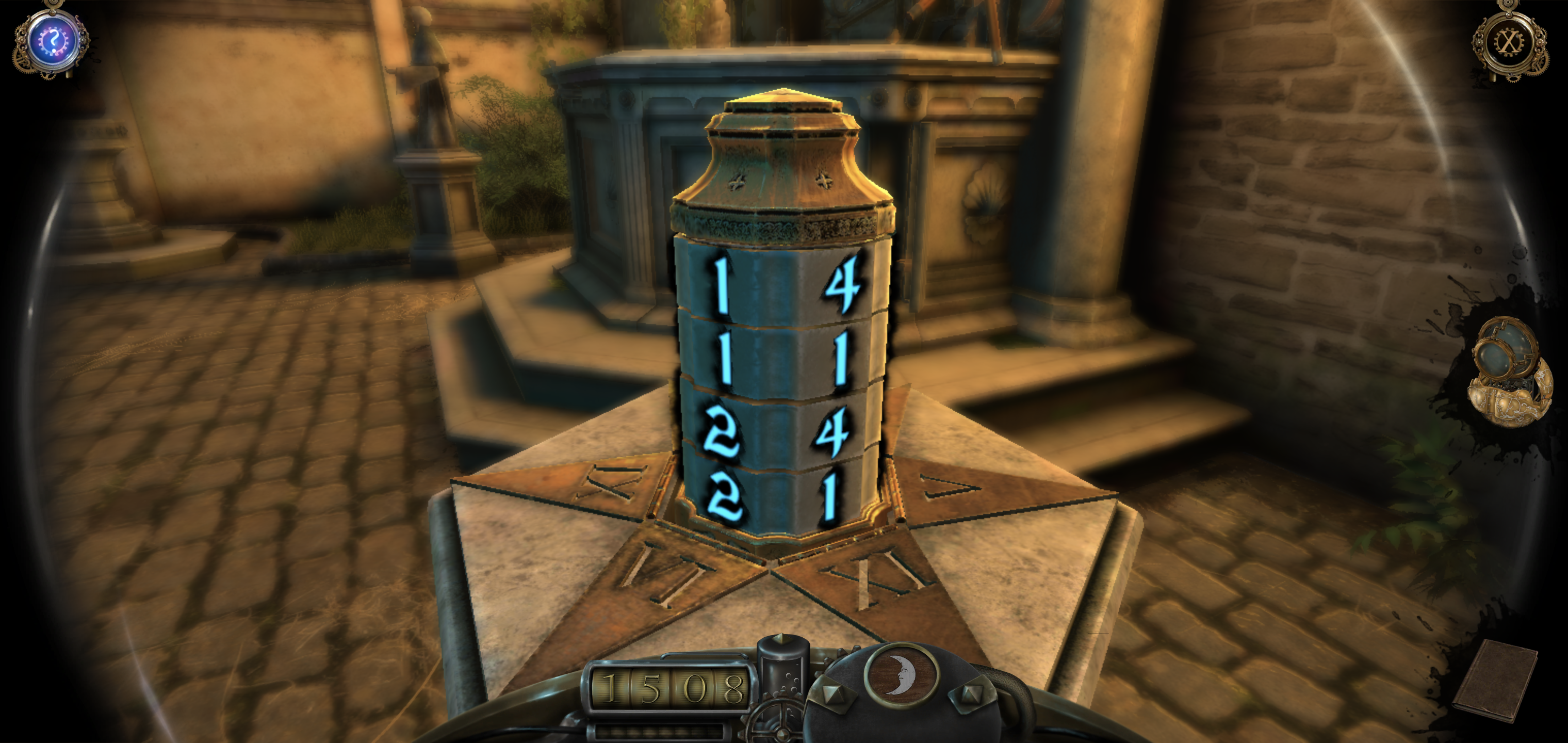
if __name__ == '__main__':
sr = SumRotate()
init = (
(4, 1, 1, 1, 3, 1),
(1, 1, 2, 1, 3, 1),
(4, 1, 3, 1, 2, 2),
(1, 2, 3, 1, 3, 2)
)
goal = (11, 5, 10, 4, 9, 6)
solved = sr.solve(init, goal, max_depth=2000)
goal_state = sr.goal_state
if goal_state:
sr.show_steps(solved, goal_state)
else:
print("Cannot solve")
Init None
'''
Rotate 1 clockwise ((4, 1, 1, 1, 3, 1), (1, 1, 2, 1, 3, 1), (4, 1, 3, 1, 2, 2), (1, 2, 3, 1, 3, 2))
Rotate 1 clockwise ((4, 1, 1, 1, 3, 1), (1, 2, 1, 3, 1, 1), (4, 1, 3, 1, 2, 2), (1, 2, 3, 1, 3, 2))
Finished ((4, 1, 1, 1, 3, 1), (2, 1, 3, 1, 1, 1), (4, 1, 3, 1, 2, 2), (1, 2, 3, 1, 3, 2))
Solved in 4 steps
'''
if __name__ == '__main__':
solver = LinkedRotaryPuzzle(
((0, 6), (0, 6), (0, 6)),
{
0: {
2: -1
},
1: {
0: -1
},
2: {
1: 1
}
}
)
visited = solver.solve((1, 0, 0), (0, 0, 0))
solver.show_steps(visited, (0, 0, 0))