Created
June 24, 2022 17:18
-
-
Save jdhitsolutions/39785364aa66e0f1cf83eb9c33b99e19 to your computer and use it in GitHub Desktop.
This is a revised version of an AnyBox example.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#requires -version 5.1 | |
#requires -RunasAdministrator | |
#requires -module AnyBox | |
# https://www.fresh2.dev/doc/anybox/ | |
# this is a revised version of the example at https://github.com/fresh2dev/AnyBox/blob/main/Examples/Process-Mgr.ps1 | |
Param([string]$Computername = $env:Computername) | |
$anybox = New-Object AnyBox.AnyBox | |
$anybox.Title = 'Process Mgr' | |
$anybox.ResizeMode = 'CanResizeWithGrip' | |
$anybox.MaxHeight = 800 | |
$anybox.MaxWidth = 600 | |
$anybox.Topmost = $true | |
$anybox.AccentColor = 'Black' | |
$anybox.GridData = @(Get-CimInstance -Class Win32_Process -ea Stop | Select-Object ProcessId, ProcessName, CommandLine) | |
$anybox.NoGridSearch = $true | |
$anybox.SelectionMode = [AnyBox.DataGridSelectionMode]::MultiRow | |
# Define the computer name prompt; the field must not be empty and the computer must be online. | |
$paramHash = @{ | |
Group = 0 | |
Name = 'pcName' | |
Message = 'Computer Name:' | |
MessagePosition = 'Left' | |
DefaultValue = $Computername.ToUpper() | |
ValidateNotEmpty = $True | |
ValidateScript = { Test-Connection $_ -Count 1 -Quiet -ErrorAction SilentlyContinue } | |
} | |
$anybox.Prompts = @(New-AnyBoxPrompt @paramHash) | |
# Define the process filter prompt | |
$paramHash.Name = 'pFilter' | |
$paramhash.Message = ' Process Name:' | |
$paramHash.DefaultValue = "*" | |
$paramHash.Remove('ValidateScript') | |
$anybox.Prompts += @(New-AnyBoxPrompt @paramhash) | |
# Define the 'Refresh' button. | |
$anybox.Buttons = New-AnyBoxButton -Text 'Refresh' -IsDefault -OnClick { | |
# Run 'Test-ValidateInput' to enforce | |
# the validation parameters set on the 'pcName' prompt. | |
$input_test = Test-ValidInput -Prompts $Prompts -Inputs $form.Result | |
if (-not $input_test.Is_Valid) { | |
$null = Show-AnyBox @childWinParams -Message $input_test.Message -Buttons $(New-AnyBoxButton -Text 'OK' -IsDefault) | |
$form['data_grid'].ItemsSource = $null | |
} | |
else { | |
[string]$msg = $null | |
try { | |
# Get all running process matching the filter. | |
# '$_.pcName' will access the text in the 'pcName' prompt. | |
# '$_.pFilter' will access the text in the 'pFilter' prompt. | |
$new_data = @(Get-CimInstance -ComputerName $_.pcName -Class 'Win32_Process' ` | |
-Filter "Name LIKE '$($_.pFilter.Replace('*', '%'))'" -ea Stop | | |
Select-Object ProcessId, ProcessName, CommandLine) | |
if ($new_data.Length -eq 0) { | |
$form['data_grid'].ItemsSource = $null | |
$msg = 'No processes match the provided filter.' | |
} | |
else { | |
# Update the grid with matching processes. | |
$form['data_grid'].ItemsSource = $new_data | |
} | |
} | |
catch { | |
$msg = $_.Exception.Message | |
} | |
# If an error occurs, show another AnyBox. | |
# Show a child window with @childWinParams. | |
if ($msg) { | |
Show-AnyBox @childWinParams -Message $msg -Buttons 'OK' | |
} | |
} | |
} | |
$anybox.Buttons += New-AnyBoxButton -Text "Export to CSV" -onclick { | |
$savePrompt = Show-AnyBox @childwinparams -MinWidth 350 -Buttons 'Cancel', 'Export' -Prompt @((New-AnyBoxPrompt -InputType 'FileSave' -Message 'Specify the filename and path for the CSV file.' -ReadOnly)) | |
if ($savePrompt.Export) { | |
$form['data_grid'].ItemsSource | | |
Add-member -MemberType NoteProperty -name Computername -value $_.pcname.toUpper() -passthru | | |
Export-CSV -path $savePrompt.Input_0 | |
Show-Anybox @childWinParams -title 'CSV Export' -Messages "Data saved to $($savePrompt.Input_0)" -buttons "OK" -MinWidth 250 | |
} | |
} | |
$anybox.Buttons += New-AnyBoxButton -Text 'Kill' -OnClick { | |
# Run 'Test-ValidateInput' to enforce | |
# the validation parameters set on the 'pcName' prompt. | |
$input_test = Test-ValidInput -Prompts $Prompts -Inputs $form.Result | |
if (-not $input_test.Is_Valid) { | |
$null = Show-AnyBox @childWinParams -Message $input_test.Message -Buttons $(New-AnyBoxButton -Text 'OK' -IsDefault) | |
$form['data_grid'].ItemsSource = $null | |
} | |
else { | |
# Access selected grid rows with 'grid_select'. | |
[array]$toKill = @($_.grid_select | Select-Object ProcessId, ProcessName) | |
if ($toKill.Length -eq 0) { | |
$null = Show-AnyBox @childWinParams -Message 'Select processes to kill.' -Buttons 'OK' | |
} | |
else { | |
# Confirm before killing the selected processes. | |
$answer = Show-AnyBox @childWinParams -Message 'Are you sure you want to kill the following processes?' ` | |
-GridData $toKill -Buttons 'Cancel', 'Confirm' | |
if ($answer['Confirm']) { | |
[string]$pcName = $_.pcName | |
$killed = @($toKill | ForEach-Object { | |
[int]$code = 0 | |
[string]$msg = $null | |
try { | |
$code = Get-CimInstance -ClassName win32_process -Filter "processid= $($_.processid)" -ComputerName $PCName | | |
Invoke-CimMethod -Name Terminate | Select-Object -ExpandProperty ReturnValue | |
#([wmi]"\\$pcName\root\cimv2:Win32_Process.Handle='$($_.ProcessId)'").Terminate().ReturnValue | |
if ($code -eq 0) { | |
$msg = 'Successfully closed.' | |
} | |
} | |
catch { | |
$code = -1 | |
$msg = $_.Exception.Message | |
} | |
$_ | Select-Object ProcessId, ProcessName, @{Name = 'Code'; Expression = { $code } }, @{Name = 'Message'; Expression = { $msg } } | |
}) | |
[string]$msg = $null | |
try { | |
$new_data = @(Get-CimInstance -ComputerName $_.pcName -Class 'Win32_Process' ` | |
-Filter "Name LIKE '$($_.pFilter.Replace('*', '%'))'" -ea Stop | | |
Select-Object ProcessId, ProcessName, CommandLine) | |
if ($new_data.Length -eq 0) { | |
$form['data_grid'].ItemsSource = $null | |
} | |
else { | |
$form['data_grid'].ItemsSource = $new_data | |
} | |
} | |
catch { | |
$msg = $_.Exception.Message | |
} | |
# Output the results in child AnyBox with a DataGrid. | |
$null = Show-AnyBox @childWinParams -GridData $killed -Buttons 'OK' | |
# If an error occurred, show it in a child AnyBox. | |
if ($msg) { | |
$null = Show-AnyBox @childWinParams -Message $msg -Buttons 'OK' | |
} | |
} | |
} | |
} | |
} | |
$anybox.Buttons += New-AnyBoxButton -Text Quit -IsCancel | |
[void]($anybox | Show-AnyBox) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Some screen shots:
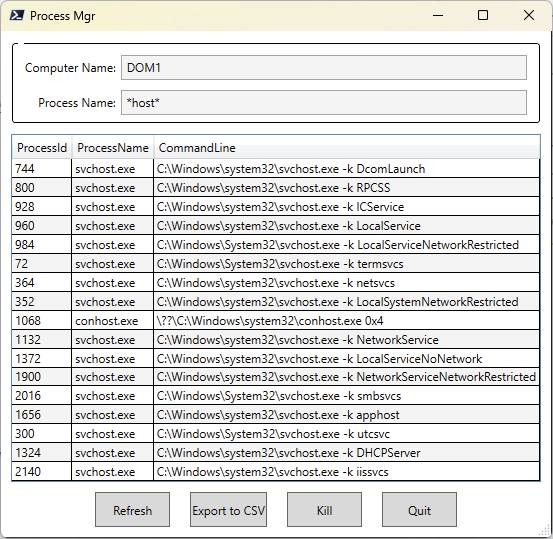
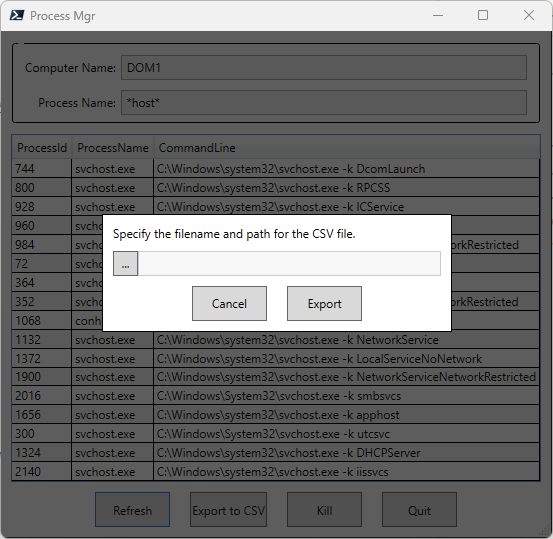