Created
August 4, 2011 14:22
-
-
Save jfromaniello/1125265 to your computer and use it in GitHub Desktop.
The powershell continuously watch for file changes in a directory, then it executes PhantomJs with the Run-Qunit.js attached to your htm qunit test harness.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# watch a file changes in the current directory, | |
# execute all tests when a file is changed or renamed | |
$watcher = New-Object System.IO.FileSystemWatcher | |
$watcher.Path = get-location | |
$watcher.IncludeSubdirectories = $true | |
$watcher.EnableRaisingEvents = $false | |
$watcher.NotifyFilter = [System.IO.NotifyFilters]::LastWrite -bor [System.IO.NotifyFilters]::FileName | |
while($TRUE){ | |
$result = $watcher.WaitForChanged([System.IO.WatcherChangeTypes]::Changed -bor [System.IO.WatcherChangeTypes]::Renamed -bOr [System.IO.WatcherChangeTypes]::Created, 1000); | |
if($result.TimedOut){ | |
continue; | |
} | |
write-host "Change in " + $result.Name | |
phantomjs.exe run-qunit.js All.Tests.htm | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/** | |
* Wait until the test condition is true or a timeout occurs. Useful for waiting | |
* on a server response or for a ui change (fadeIn, etc.) to occur. | |
* | |
* @param testFx javascript condition that evaluates to a boolean, | |
* it can be passed in as a string (e.g.: "1 == 1" or "$('#bar').is(':visible')" or | |
* as a callback function. | |
* @param onReady what to do when testFx condition is fulfilled, | |
* it can be passed in as a string (e.g.: "1 == 1" or "$('#bar').is(':visible')" or | |
* as a callback function. | |
* @param timeOutMillis the max amount of time to wait. If not specified, 3 sec is used. | |
*/ | |
function waitFor(testFx, onReady, timeOutMillis) { | |
var maxtimeOutMillis = timeOutMillis ? timeOutMillis : 3001, //< Default Max Timout is 3s | |
start = new Date().getTime(), | |
condition = false, | |
interval = setInterval(function() { | |
if ( (new Date().getTime() - start < maxtimeOutMillis) && !condition ) { | |
// If not time-out yet and condition not yet fulfilled | |
condition = (typeof(testFx) === "string" ? eval(testFx) : testFx()); //< defensive code | |
} else { | |
if(!condition) { | |
// If condition still not fulfilled (timeout but condition is 'false') | |
console.log("'waitFor()' timeout"); | |
phantom.exit(1); | |
} else { | |
// Condition fulfilled (timeout and/or condition is 'true') | |
console.log("'waitFor()' finished in " + (new Date().getTime() - start) + "ms."); | |
typeof(onReady) === "string" ? eval(onReady) : onReady(); //< Do what it's supposed to do once the condition is fulfilled | |
clearInterval(interval); //< Stop this interval | |
} | |
} | |
}, 100); //< repeat check every 250ms | |
}; | |
if (phantom.args.length === 0 || phantom.args.length > 2) { | |
console.log('Usage: run-qunit.js URL'); | |
phantom.exit(1); | |
} | |
var page = new WebPage(); | |
// Route "console.log()" calls from within the Page context to the main Phantom context (i.e. current "this") | |
page.onConsoleMessage = function(msg) { | |
console.log(msg); | |
}; | |
page.open(phantom.args[0], function(status){ | |
if (status !== "success") { | |
console.log("Unable to access network"); | |
phantom.exit(1); | |
} else { | |
waitFor(function(){ | |
return page.evaluate(function(){ | |
var el = document.getElementById('qunit-testresult'); | |
if (el && el.innerText.match('completed')) { | |
return true; | |
} | |
return false; | |
}); | |
}, function(){ | |
var failedNum = page.evaluate(function(){ | |
var tests = document.getElementById("qunit-tests").childNodes; | |
console.log("\nTest name (failed, passed, total)\n"); | |
for(var i in tests){ | |
var text = tests[i].innerText; | |
if(text !== undefined){ | |
if(/Rerun$/.test(text)) text = text.substring(0, text.length - 5); | |
console.log(text + "\n"); | |
} | |
} | |
var el = document.getElementById('qunit-testresult'); | |
console.log(el.innerText); | |
try { | |
return el.getElementsByClassName('failed')[0].innerHTML; | |
} catch (e) { } | |
return 10000; | |
}); | |
phantom.exit((parseInt(failedNum, 10) > 0) ? 1 : 0); | |
}); | |
} | |
}); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Hi Jose! Thanks a lot for sharing here continous-qunit.ps1
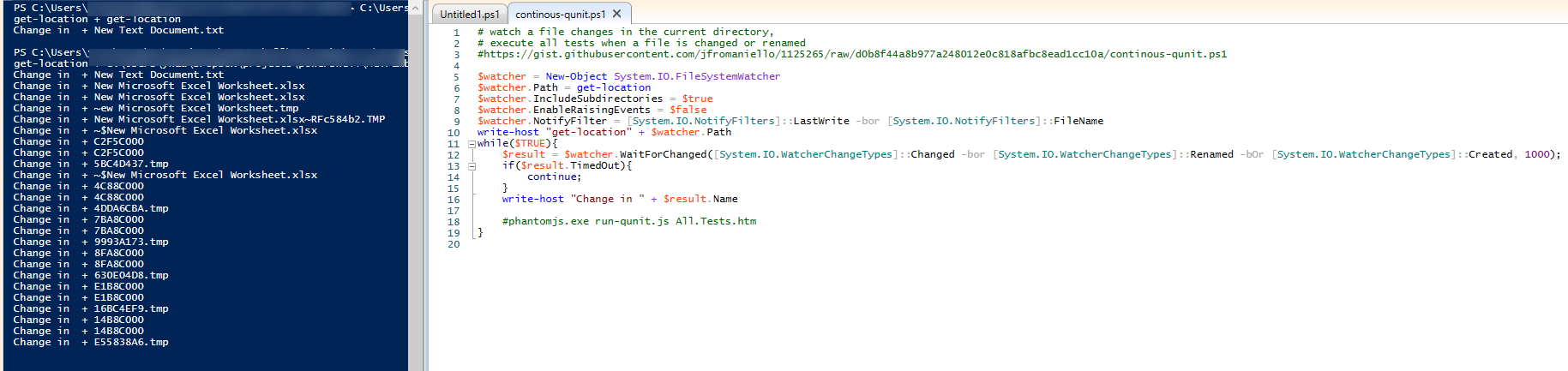
I am trying to track changes in xlsx file by your script.
for some reason it tracks .tmp files changes (which are created by xlsx file), but does not track changes in the original xlsx itself.
thanks, Yermek