Created
August 22, 2022 08:59
-
-
Save jiacheo/c01ca88ee308a8fe8293452cc71fdcb5 to your computer and use it in GitHub Desktop.
simple rsa demo
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
package org.jiacheo.awesome.blockchain.encrypt.communication; | |
import java.math.BigInteger; | |
import java.util.Random; | |
/** | |
* Created on 2022/8/22. <br/> contact: <a style="color:yellow;text-decoration:none;" | |
* href="mailto:jiacheo@qipeng.com" target="_blank">jiacheo[at]qipeng.com</a> <br/> | |
* | |
* @author jiacheo | |
*/ | |
public class SimpleRSA { | |
public static void main(String[] args) { | |
BigInteger ONE = new BigInteger("1"); | |
BigInteger TWO = new BigInteger("2"); | |
//random p、q,created for generate n、d、e,than drop them。 | |
BigInteger p = new BigInteger(128, 128, new Random()); | |
BigInteger q = new BigInteger(128, 128, new Random()); | |
BigInteger n = q.multiply(p); | |
BigInteger upperLimit = n.subtract(ONE); | |
//选择从1到n-1之间的一个随机数,且该随机数不能是偶数。否则BigInteger.modInverse 会抛异常 | |
BigInteger d; | |
Random random = new Random(); | |
do { | |
d = new BigInteger(upperLimit.bitLength(), random); | |
} | |
while (d.compareTo(upperLimit) >= 0 || d.compareTo(ONE) <= 0 || d.mod(TWO).compareTo(ONE) < 0); | |
System.out.println("p=0x" + p.toString(16)); | |
System.out.println("q=0x" + q.toString(16)); | |
System.out.println("n=0x" + n.toString(16)); | |
System.out.println("d=0x" + d.toString(16)); | |
BigInteger modulo = p.subtract(ONE).multiply(q.subtract(ONE)); | |
System.out.println("m=0x"+modulo.toString(16)); | |
BigInteger e = d.modInverse(modulo); | |
System.out.println("e=0x" + e.toString(16)); | |
//source data | |
BigInteger sourceMessage = BigInteger.probablePrime(128, random); | |
System.out.println("sourceMessage=" + sourceMessage.toString(36)); | |
//encrypt, e/n pair is public key | |
BigInteger data = sourceMessage.modPow(e, n); | |
System.out.println("encryptedData=0x" + data.toString(16)); | |
//decrypt, d/n pair is private key | |
BigInteger decryptedData = data.modPow(d, n); | |
System.out.println("decryptedData=" + decryptedData.toString(36)); | |
//compare equivalent | |
System.out.println("equals?="+sourceMessage.equals(decryptedData)); | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
come from the paper (author by Christian Reitwießner), the theory shows as below screent shots:
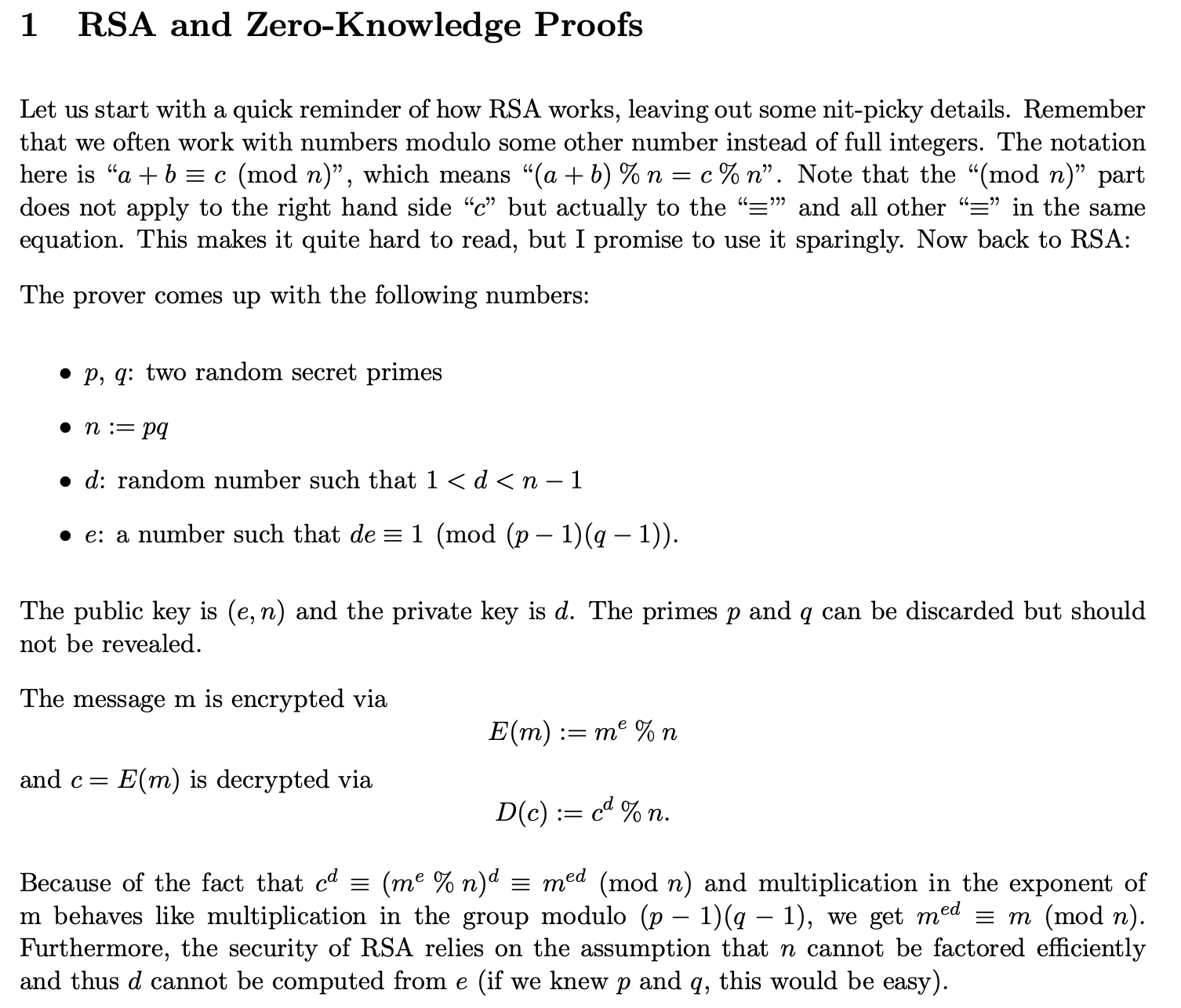