Last active
May 31, 2024 07:49
-
-
Save jimevans/d53e0d4150fa784674594c65be152ddf to your computer and use it in GitHub Desktop.
Selenium C# network traffic logging example
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
public async Task LogNetworkRequests(IWebDriver driver) | |
{ | |
INetwork interceptor = driver.Manage().Network; | |
interceptor.NetworkRequestSent += OnNetworkRequestSent; | |
interceptor.NetworkResponseReceived += OnNetworkResponseReceived; | |
await interceptor.StartMonitoring(); | |
driver.Url = "http://the-internet.herokuapp.com/redirect"; | |
await interceptor.StopMonitoring(); | |
} | |
private void OnNetworkRequestSent(object sender, NetworkRequestSentEventArgs e) | |
{ | |
StringBuilder builder = new StringBuilder(); | |
builder.AppendFormat("Request {0}", e.RequestId).AppendLine(); | |
builder.AppendLine("--------------------------------"); | |
builder.AppendFormat("{0} {1}", e.RequestMethod, e.RequestUrl).AppendLine(); | |
foreach (KeyValuePair<string, string> header in e.RequestHeaders) | |
{ | |
builder.AppendFormat("{0}: {1}", header.Key, header.Value).AppendLine(); | |
} | |
builder.AppendLine("--------------------------------"); | |
builder.AppendLine(); | |
Console.WriteLine(builder.ToString()); | |
} | |
private void OnNetworkResponseReceived(object sender, NetworkResponseReceivedEventArgs e) | |
{ | |
StringBuilder builder = new StringBuilder(); | |
builder.AppendFormat("Response {0}", e.RequestId).AppendLine(); | |
builder.AppendLine("--------------------------------"); | |
builder.AppendFormat("{0} {1}", e.ResponseStatusCode, e.ResponseUrl).AppendLine(); | |
foreach (KeyValuePair<string, string> header in e.ResponseHeaders) | |
{ | |
builder.AppendFormat("{0}: {1}", header.Key, header.Value).AppendLine(); | |
} | |
if (e.ResponseResourceType == "Document") | |
{ | |
builder.AppendLine(e.ResponseBody); | |
} | |
else if (e.ResponseResourceType == "Script") | |
{ | |
builder.AppendLine("<JavaScript content>"); | |
} | |
else if (e.ResponseResourceType == "Stylesheet") | |
{ | |
builder.AppendLine("<stylesheet content>"); | |
} | |
else if (e.ResponseResourceType == "Image") | |
{ | |
builder.AppendLine("<image>"); | |
} | |
else | |
{ | |
builder.AppendFormat("Content type: {0}", e.ResponseResourceType).AppendLine(); | |
} | |
builder.AppendLine("--------------------------------"); | |
Console.WriteLine(builder.ToString()); | |
} |
Yes, this is the error! Does not work!
This gist seems alright, but the exception seems to be due to an issue in Selenium itself. A similar bug has been reported under SeleniumHQ/selenium#10054 (comment)
it work for me
Thanks your code, the best...
Thank you.
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Hi its raise exception as below , please help me out solve this issue .
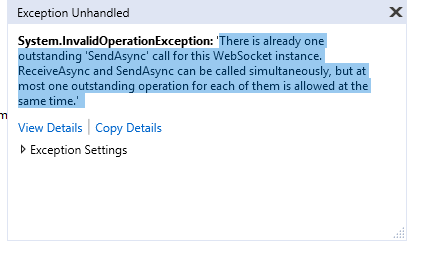