Last active
April 6, 2023 11:16
-
-
Save jkcorrea/365daf1c60a88d07902ecad1bdad131d to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<!-- Add this to the Head section of your super site --> | |
<script> | |
// Say we host our Super site in a subdirectory e.g. https://example.com/blog | |
// We can redirect it properly in Cloudflare Worker, but relative links will break | |
// So we require some custom code to override absolute href paths & history state changes | |
if (window.location.origin === 'https://example.com') { | |
var baseDir = '/blog'; | |
function historyMethodOverride(target, thisArg, argArray) { | |
// prepend baseDir if it isn't there | |
var newPath = argArray[2]; | |
if (newPath && !newPath.startsWith(baseDir)) { | |
argArray[2] = baseDir + (newPath === '/' ? '' : newPath) | |
} | |
target.apply(thisArg, argArray); | |
// Needs a timeout for doc to load | |
setTimeout(updateAllAnchors, 0) | |
} | |
// Super uses pushState/replaceState to navigate. Override it to prepend our baseDir | |
window.history.pushState = new Proxy(window.history.pushState, { apply: historyMethodOverride }); | |
window.history.replaceState = new Proxy(window.history.replaceState, { apply: historyMethodOverride }); | |
function updateAllAnchors() { | |
var anchors = document.getElementsByTagName("a"); | |
for (var i = 0; i < anchors.length; i++) { | |
var a = anchors[i]; | |
var href = a.getAttribute('href'); | |
if (href.substring(0, 1) === '/' && !href.startsWith(baseDir)) { | |
a.setAttribute('href', baseDir + (href === '/' ? '' : href)); | |
} | |
} | |
} | |
// Also rewrite anchor href's to prepend baseDir, in case anyone right clicks the links or for googlebot | |
window.addEventListener('pageshow', updateAllAnchors); | |
// We also need to trigger it on back/forward calls | |
window.addEventListener('popstate', updateAllAnchors); | |
} | |
</script> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// Add this in your cloudflare worker | |
const TO_DOMAIN = 'example.super.site' | |
const FROM_DOMAIN = 'example.com' | |
const FROM_SUBDIR = '/blog' | |
const ADDITIONAL_PATHS = ['/_next'] // Don't forget to load assets referenced with relative paths | |
addEventListener("fetch", event => { | |
event.respondWith(handleRequest(event.request)) | |
}) | |
async function handleRequest(request) { | |
const sourceUrl = new URL(request.url) | |
const reqPath = sourceUrl.pathname | |
if (sourceUrl.pathname.startsWith(FROM_SUBDIR) | |
|| ADDITIONAL_PATHS.some(p => sourceUrl.pathname.startsWith(p))) { | |
sourceUrl.hostname = TO_DOMAIN; | |
sourceUrl.pathname = sourceUrl.pathname.replace(FROM_SUBDIR, '') | |
return fetch(sourceUrl, request, { | |
cf: { resolveOverride: FROM_DOMAIN } | |
}) | |
} | |
return fetch(request) | |
} |
This is working like a charm 💯
working thanks
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Enables hosting your super.so site on a subdirectory of your domain (e.g.
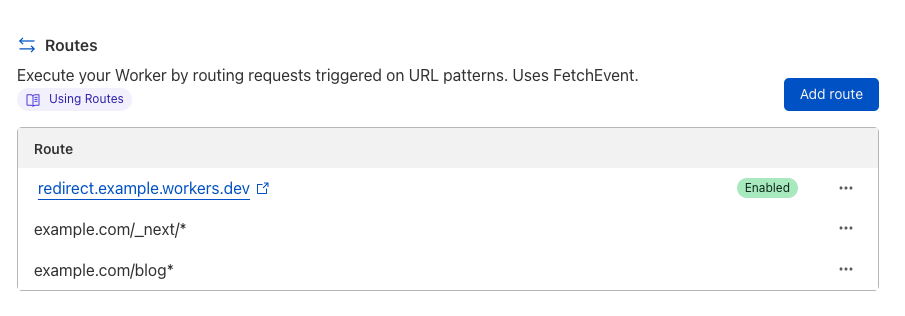
https://example.com/blog
) using Cloudflare Workers. Cloudflare Worker config should look something like this: