These instructions set up a way to publish tweets to Twitter's OAuth2 API from a Ruby on Rails app.
-
Set up a twitter account via the Twitter account setup instructions here: https://github.com/jkotchoff/twitter_oauth2#twitter-account-setup
-
Add the twitter_oauth2 gem and something to issue HTTP requests (like Typhoeus) to your Gemfile:
gem 'twitter_oauth2'
gem 'typhoeus'
- Introduce a rails migration to persist your oauth2 token in a single database record in a table (and update it when it needs refreshing)
class CreateTwitterTokens < ActiveRecord::Migration[7.0]
def change
create_table :twitter_tokens do |t|
t.string :marshaled_client, null: false
t.string :marshaled_token, null: false
t.timestamps
end
end
end
-
Retrieve and persist your token (refer one-off.rb in this gist)
-
Use the token to write tweets to the twitter API, refreshing and persisting the token when it expires (refer app/models/twitter.rb in this gist)
hey @jkotchoff I am trying to use this code for authorization and I have a sample like you put here. Just inside controller index action I put all the code from [2-one-off.rb] file and now I come to code and I stuck there I also put my real callback of my site live there
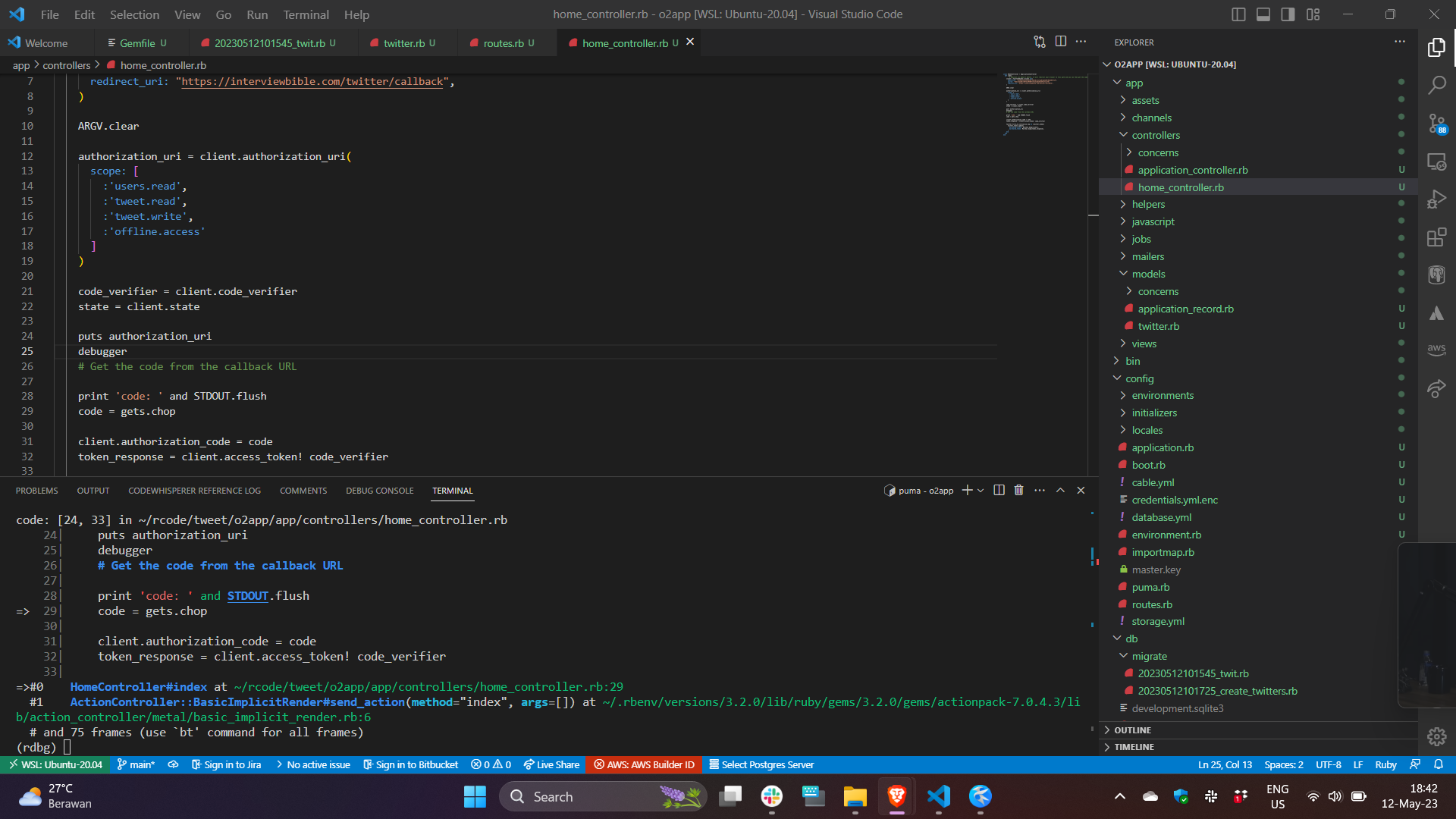
SO I suppose I would need to see somewhere this code and put here in console but I dont see it, also I dont see any request to my callback. What am I doing wrong here?