Created
March 24, 2021 02:31
-
-
Save jlumbroso/c0ec0c4f1a0a502e3835c183cbe89c65 to your computer and use it in GitHub Desktop.
Using pyppeteer to screenshot a Single-Page App
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# How to use pyppeteer to screenshot an SPA | |
# Joseph Lou & Jérémie Lumbroso, 2021 | |
# | |
# This example code is hardcoded for https://codepost.io but should | |
# show how to use pyppeteer for other SPAs | |
import asyncio | |
# ref: https://github.com/miyakogi/pyppeteer/issues/219#issuecomment-563077061 | |
import pyppdf.patch_pyppeteer # needed to avoid chromium errors | |
from pyppeteer import launch | |
LOGIN_URL = "https://codepost.io/login" | |
TARGET_URL = "https://codepost.io/code/239403/" # <-- set submission you want to screenshot | |
JWT_KEY = "eyJ0e..." # <-- replace token here | |
async def main(): | |
browser = await launch() | |
page = await browser.newPage() | |
# await page.emulate({ | |
# "viewport": { | |
# "width": 500, | |
# "height": 2400, | |
# }, | |
# "userAgent": "" | |
# }) | |
# Load a page (any page), to set the local storage (with a Javascript console call) | |
# to store the JWT, which subsequent API calls will use | |
await page.goto(LOGIN_URL) | |
await page.evaluate(f"() => {{ localStorage.setItem('token', '{JWT_KEY}'); }}"); | |
# Load the target page and wait to make sure every it rendered (important for | |
# a Single-Page App like codepost.io) | |
# ref: https://stackoverflow.com/a/62859172/408734 | |
await page.goto( | |
TARGET_URL, | |
{ | |
"waitUntil" : [ | |
"load", # when load event is fired. | |
"domcontentloaded", # when the DOMContentLoaded event is fired | |
"networkidle0", # when there are no more than 0 network connections | |
# for at least 500 ms | |
"networkidle2" # when there are no more than 2 network connections | |
# for at least 500 ms | |
]}, | |
) | |
await page.pdf(path="example.pdf") | |
await page.screenshot(path="example.png") | |
await browser.close() | |
print("done") | |
asyncio.get_event_loop().run_until_complete(main()) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Result:
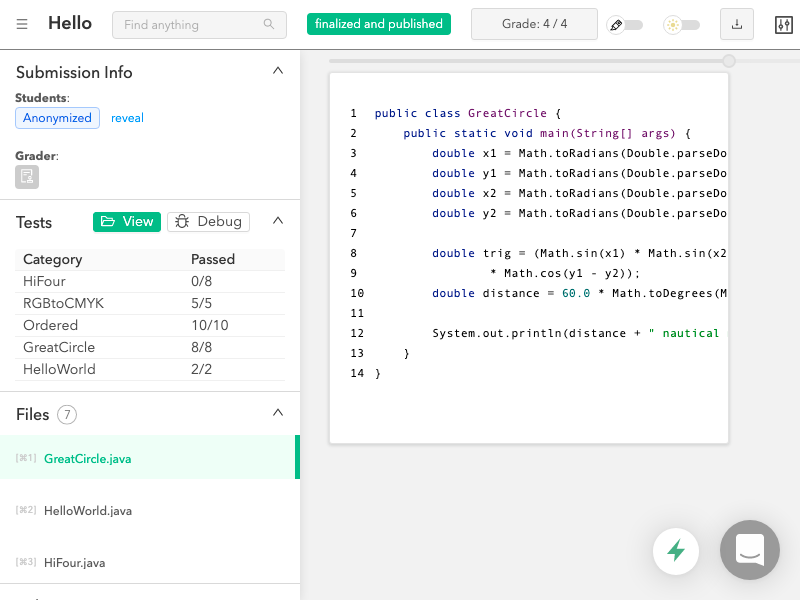