Last active
March 20, 2018 18:49
-
-
Save joemsak/b1b39945770ac54bd14f71813a18a7ae to your computer and use it in GitHub Desktop.
v-select in rails 5.1 webpacker vue
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<!DOCTYPE html> | |
<html> | |
<head> | |
<!-- other HEAD stuff omitted --> | |
<%= stylesheet_link_tag("//fonts.googleapis.com/css?family=Roboto:300,400,500,700|Material+Icons") %> | |
<%= yield :css %> | |
<%= yield :js %> | |
</head> | |
<body> | |
<%= yield %> | |
</body> | |
</html> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<% provide :title, "Admin Data • Participants" %> | |
<% provide :js, javascript_pack_tag("admin") %> | |
<% provide :css, stylesheet_pack_tag("admin") %> | |
<div class="panel"> | |
<div id="app"> | |
<v-app> | |
<v-container> | |
<!-- other filters --> | |
<season-filter></season-filter> | |
</v-container> | |
</v-app> | |
</div> | |
</div> | |
<!-- results from searching with filters --> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import TurbolinksAdapter from 'vue-turbolinks'; | |
import Vue from 'vue/dist/vue.esm'; | |
import Vuex from 'vuex' | |
import Vuetify from 'vuetify' | |
import _ from 'lodash' | |
Vue.use(Vuex) | |
Vue.use(Vuetify) | |
import VTooltip from 'v-tooltip'; | |
import SeasonFilter from '../admin/SeasonFilter' | |
import "../components/tooltip.scss"; | |
Vue.use(TurbolinksAdapter); | |
Vue.use(VTooltip); | |
import 'vuetify/dist/vuetify.min.css' | |
import '../admin/main.scss' | |
const store = new Vuex.Store({ | |
state: { | |
searchFilters: { | |
seasons: // filter values and properties..., | |
}, | |
}, | |
getters: { | |
searchFilters: (state) => (opts) => { | |
// return filter from opts... | |
} | |
}, | |
mutations: { | |
searchFilters (state, opts) { | |
// remote ajax with filters | |
}, | |
}, | |
}) | |
document.addEventListener('DOMContentLoaded', () => { | |
new Vue({ | |
el: "#app", | |
store, | |
computed: { | |
}, | |
components: { | |
SeasonFilter, | |
}, | |
mounted () { | |
console.log('admin participants') | |
}, | |
}); | |
}); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<template> | |
<div> | |
<v-subheader>Seasons</v-subheader> | |
<v-select | |
label="Select" | |
:items="seasons" | |
v-model="selected" | |
multiple | |
chips | |
deletable-chips | |
hint="Choose seasons" | |
persistent-hint | |
></v-select> | |
</div> | |
</template> | |
<script> | |
export default { | |
name: 'SeasonFilter', | |
data () { | |
return { | |
seasons: this.$store.getters.searchFilters({ | |
name: 'seasons', | |
}), | |
selected: this.$store.getters.searchFilters({ | |
name: 'selectedSeasons', | |
}), | |
} | |
}, | |
watch: { | |
selected (collection) { | |
this.$store.commit('searchFilters', { | |
filterRoot: 'seasons', | |
value: collection | |
}) | |
}, | |
}, | |
props: [], | |
components: { | |
}, | |
methods: { | |
}, | |
mounted () { | |
console.log('season filter') | |
}, | |
} | |
</script> |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Chrome Dev Tools inspecting the checkboxes in Codepen
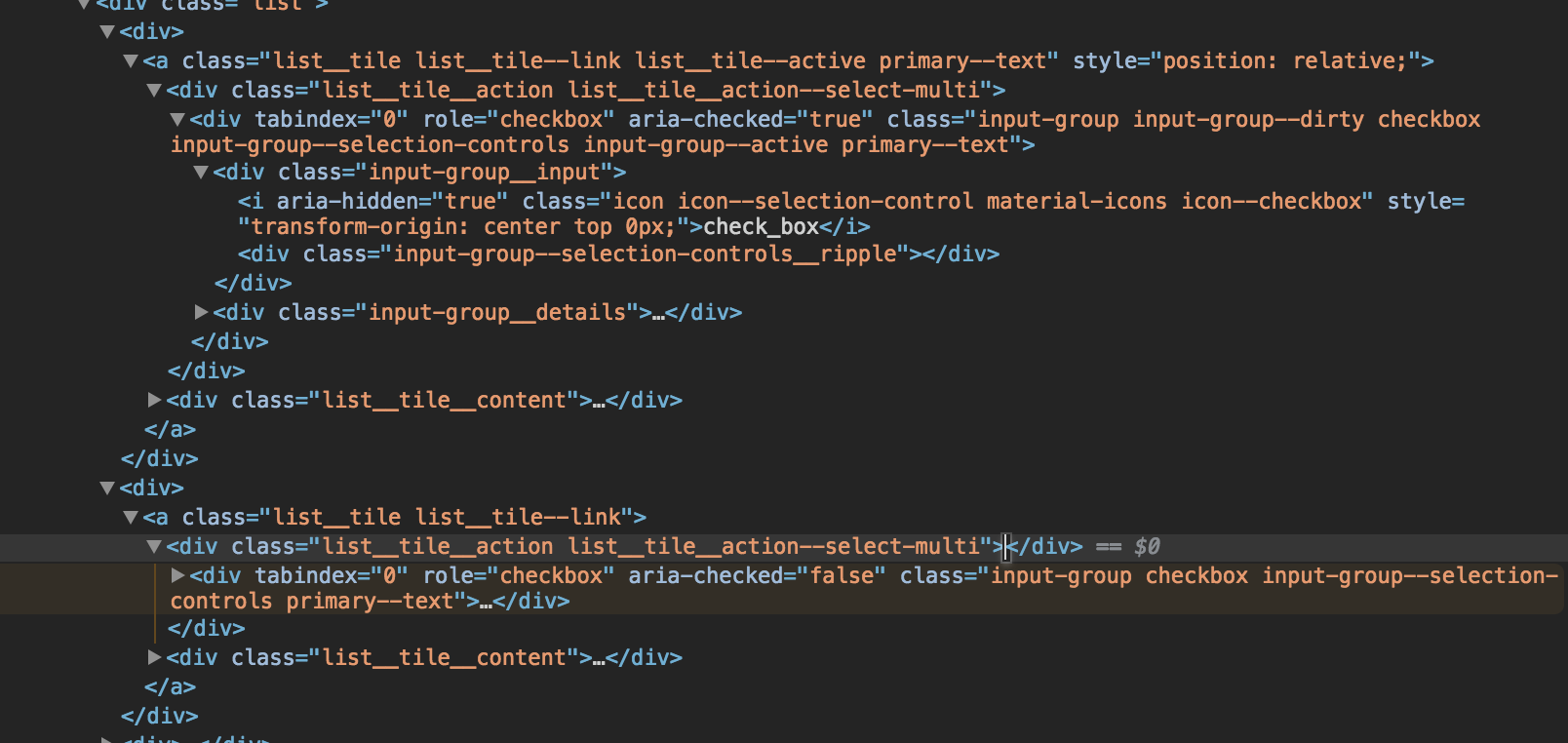