Last active
November 28, 2021 21:49
-
-
Save josephmcasey/e5a7a0e0c16be48eb4f5cfaacb2a76d8 to your computer and use it in GitHub Desktop.
A script to setup my local Ubuntu environment for the purpose of development
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# DEPENDENCY: | |
# - Requires OS of Ubuntu 21.04 | |
# - Requires ethernet connection. | |
# This gist shows what is required from a simple script to setup a procedurally generate my local development environment from GitHub. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/usr/bin/env bash | |
# Alias definitions. | |
echo "Configuring Aliases on Local System" | |
# You may want to put all your additions into a separate file like this. | |
# See /usr/share/doc/bash-doc/examples in the bash-doc package. | |
# Single Character Shortcuts | |
alias c='clear' | |
alias g='git' | |
alias h='history' | |
alias j='jobs -l' | |
alias k='kubectl' | |
alias l='ls -lAh' | |
# Safety Nets | |
alias mv='mv -i' | |
alias cp='cp -i' | |
alias ln='ln -i' | |
alias rm='rm -I --preserve-root' | |
# Applications | |
alias notes=/opt/Notable/notable | |
alias chrome='/opt/google/chrome/chrome' | |
# Overrides | |
alias ls='ls -alF' | |
# Utilities | |
alias ..='cd ..' | |
alias ...='cd ../../../' | |
alias ....='cd ../../../../' | |
alias .....='cd ../../../../' | |
# System Commands | |
alias reboot='sudo /sbin/reboot' | |
alias poweroff='sudo /sbin/poweroff' | |
alias halt='sudo /sbin/halt' | |
alias shutdown='sudo /sbin/shutdown' |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# ~/.bashrc: executed by bash(1) for non-login shells. | |
# see /usr/share/doc/bash/examples/startup-files (in the package bash-doc) | |
# for examples | |
# If not running interactively, don't do anything | |
case $- in | |
*i*) ;; | |
*) return;; | |
esac | |
# append to the history file, don't overwrite it | |
shopt -s histappend | |
# External Configuration | |
set -a | |
source ~/.env | |
source ~/.aliases | |
source ~/.cfg_bash | |
source ~/.cfg_node | |
source ~/.cfg_go | |
source ~/.cfg_python | |
set +a | |
# check the window size after each command and, if necessary, | |
# update the values of LINES and COLUMNS. | |
shopt -s checkwinsize | |
# make less more friendly for non-text input files, see lesspipe(1) | |
[ -x /usr/bin/lesspipe ] && eval "$(SHELL=/bin/sh lesspipe)" | |
# enable programmable completion features (you don't need to enable | |
# this, if it's already enabled in /etc/bash.bashrc and /etc/profile | |
# sources /etc/bash.bashrc). | |
if ! shopt -oq posix; then | |
if [ -f /usr/share/bash-completion/bash_completion ]; then | |
. /usr/share/bash-completion/bash_completion | |
elif [ -f /etc/bash_completion ]; then | |
. /etc/bash_completion | |
fi | |
fi | |
# Ease-of-Access Command Recommendations | |
echo 'Remember to authenticate OnePassword CLI with: op_off && op_on' |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/usr/bin/bash | |
op_on() { | |
if [[ -z $OP_SESSION_my ]]; then | |
eval $(op signin my) | |
fi | |
} | |
op_off() { | |
op signout | |
unset OP_SESSION_my | |
} | |
op_get() { | |
op get item "$1" |jq -r '.details.fields[] |select(.designation=="password").value' | |
} | |
op_env() { | |
op get item ENV --fields $1 | |
} | |
github_token() { | |
export GIT_TOKEN=$(op get item "GitHub"|jq -r '.details.sections[] | select(.fields).fields[] | select(.t== "Personal Access Token").v') | |
} | |
op_mfa() { | |
op get totp "$1" | |
} | |
mycli() | |
{ | |
# Display documentation on custom commands | |
echo 'This provides command line interface documentation for custom commands on this machine:' | |
echo | |
echo 'OnePassword CLI:' | |
echo ' op_on - Authenticate OnePassword CLI' | |
echo ' op_off - Unauthenticate OnePassword CLI' | |
echo ' op_get $1 - Retrieve password value for provided argument from OnePassword' | |
echo ' op_env $1 - Retrieve Known Key for ENV from OnePassword' | |
echo ' github_token - Retrieve GitHub Account Token from OnePassword' | |
echo ' op_mfa - Use OnePassword ' | |
echo | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/usr/bin/env bash | |
echo "Configuring Go on Local System" | |
GOCACHE=/tmp/ |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/usr/bin/env bash | |
# Contains any local configuration for the Node/JavaScript GPPL | |
echo "Configuring NodeJS on Local System" | |
[ -s "$NVM_DIR/nvm.sh" ] && \. "$NVM_DIR/nvm.sh" # This loads nvm | |
[ -s "$NVM_DIR/bash_completion" ] && \. "$NVM_DIR/bash_completion" # This loads nvm bash_completion |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/usr/bin/env bash | |
echo "Configuring Python on Local System" |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/usr/bin/env bash | |
echo "Set Environment Variables for Local System" | |
ACCOUNT="josephmcasey" | |
NAME="Joseph Casey" | |
EMAIL="me@josephmcasey.com" | |
# colored GCC warnings and errors | |
GCC_COLORS='error=01;31:warning=01;35:note=01;36:caret=01;32:locus=01:quote=01' | |
# Colorful Commands | |
alias ls='ls --color=auto' | |
alias grep='grep --color=auto' | |
alias fgrep='fgrep --color=auto' | |
alias egrep='egrep --color=auto' | |
# don't put duplicate lines or lines starting with space in the history. | |
# for setting history length see HISTSIZE and HISTFILESIZE in bash(1) | |
HISTCONTROL=ignoreboth | |
HISTSIZE=1000 | |
HISTFILESIZE=2000 | |
# Terminal Prompt | |
PS1='${debian_chroot:+($debian_chroot)}\[\033[01;32m\]\u@\h\[\033[00m\]:\[\033[01;34m\]\w\[\033[00m\]\$ ' | |
# Terminal Editor Options: | |
# /bin/nano | |
# /usr/bin/vim.basic | |
# /usr/bin/vim.tiny | |
# /usr/bin/emacs | |
# /bin/ed | |
SELECTED_EDITOR="/bin/nano" | |
# ====== | |
# Directories | |
# ====== | |
# NodeJS Version Manager | |
NVM_DIR="$HOME/.nvm" | |
GITHUB="$HOME/github.com" | |
GIST="$HOME/gist.github.com" | |
GITLAB="$HOME/gitlab.com" | |
# ====== | |
# Cloud | |
# ====== | |
# AWS - https://docs.aws.amazon.com/cli/latest/userguide/cli-configure-envvars.html#envvars-list | |
AWS_ACCESS_KEY_ID=[...] | |
AWS_SECRET_ACCESS_KEY=[...] | |
AWS_DEFAULT_REGION=us-east-1 | |
# GCP - | |
PROJECT_ID=[...] | |
# Azure - https://docs.microsoft.com/en-us/cli/azure/azure-cli-configuration#cli-configuration-file | |
AZURE_CONFIG_DIR=[...] | |
# ====== | |
# K8s | |
# ====== | |
KUBECONFIG=$HOME/.kube/config | |
KUBECONFIG_SAVED=$KUBECONFIG |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
[core] | |
excludesfile = $HOME/.gitignore | |
[user] | |
email = me@josephmcasey.com | |
name = Joseph Casey | |
[init] | |
defaultBranch = main | |
[credential] | |
helper = store | |
[alias] | |
unstage = reset HEAD -- | |
visual = !gitk | |
co = checkout | |
a = add -p | |
b = branch | |
c = commit | |
d = diff | |
f = fetch -p | |
l = log --color --graph --pretty=format:'%Cred%h%Creset -%C(yellow)%d%Creset %s %Cgreen(%cr) %C(bold blue)<%an>%Creset' --abbrev-commit | |
p = push | |
pl = pull | |
rank = shortlog -sn --no-merges | |
s = status | |
[color] | |
ui = auto |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# Global Ignored Files | |
# IDE Files | |
.idea |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# ~/.profile: executed by the command interpreter for login shells. | |
# This file is not read by bash(1), if ~/.bash_profile or ~/.bash_login | |
# exists. | |
# see /usr/share/doc/bash/examples/startup-files for examples. | |
# the files are located in the bash-doc package. | |
# the default umask is set in /etc/profile; for setting the umask | |
# for ssh logins, install and configure the libpam-umask package. | |
#umask 022 | |
# if running bash | |
if [ -n "$BASH_VERSION" ]; then | |
# include .bashrc if it exists | |
if [ -f "$HOME/.bashrc" ]; then | |
. "$HOME/.bashrc" | |
fi | |
fi | |
# set PATH so it includes user's private bin if it exists | |
if [ -d "$HOME/bin" ] ; then | |
PATH="$HOME/bin:$PATH" | |
fi | |
# set PATH so it includes user's private bin if it exists | |
if [ -d "$HOME/.local/bin" ] ; then | |
PATH="$HOME/.local/bin:$PATH" | |
fi | |
eval "$(/home/linuxbrew/.linuxbrew/bin/brew shellenv)" |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
sudo apt update | |
sudo apt install -y \ | |
apt-config-icons-hidpi \ | |
apt-transport-https \ | |
broadcom-sta-common \ | |
broadcom-sta-source \ | |
build-essential \ | |
curl \ | |
git \ | |
gnome-characters \ | |
gnome-system-monitor \ | |
grub-customizer \ | |
hardinfo \ | |
inotify-tools \ | |
linux-headers-$(uname -r) | |
sudo apt autoremove -y |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/usr/bin/env bash | |
if hash brew 2>/dev/null; then | |
echo "Linux Brew Installed. Proceeding to package installation." | |
else | |
echo "Installing Linux Brew" | |
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)" | |
fi | |
eval "$(/home/linuxbrew/.linuxbrew/bin/brew shellenv)" | |
# Terminal | |
brew install tldr \ # Explain | |
gh \ # Git Tools | |
# General Purpose Programming Languages (GPPL) | |
brew install go python node | |
# Container Orchestration & Tools | |
brew install docker \ | |
kubectl \ # Foundation | |
derailed/k9s/k9s \ # K8s Terminal Interface | |
kubeseal \ # Bitnami - K8s Sealed Secrets | |
devspace \ # Install DevSpace - https://devspace.sh/cli/docs/getting-started/installation | |
k3d \ # https://k3d.io/ | |
kops # https://kops.sigs.k8s.io/getting_started/install/ | |
# Cloud Tools | |
brew install terraform \ # Cloud Infrastructure Provisioner | |
cloud-nuke # Terragrunt - Purge AWS Account | |
# Basic Maintenance | |
brew upgrade | |
brew doctor |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/usr/bin/env bash | |
if hash snap 2>/dev/null; then | |
echo "Snap Package Manager Installed. Proceeding to package installation." | |
else | |
echo "Installing Snap package manager on Ubuntu OS" | |
sudo apt install snapd | |
fi | |
# Entertainment Applications | |
sudo snap install spotify | |
# Productivity Applications | |
sudo snap install postman notable | |
sudo snapp install --classic slack | |
# Security Applications | |
sudo snap install 1password | |
# Brew Prerequisite | |
snap install --classic ruby --channel=2.6/stable | |
sudo snap refresh |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/usr/bin/env bash | |
# WARNING: Overwrites any existing $HOME files | |
FILES=".profile .gitconfig .gitignore .bashrc .env .aliases .cfg_node .cfg_python .cfg_go .cfg_bash" | |
for f in $FILES | |
do | |
echo "Adding $f" | |
cp -f $f $HOME/$f | |
chmod a+rx $HOME/$f | |
done | |
# Use updated Bash runcom | |
source $HOME/.bashrc | |
# Run Package Managers | |
# ...for Low-Level Libraries (sort of) | |
sh .setup_apt.sh | |
# ...for Software Applications | |
sh .setup_snap.sh | |
# ...for Software Development Libraries | |
sh .setup_brew.sh | |
# GitHub | |
DIRECTORIES="github.com gist.github.com" | |
for d in $DIRECTORIES | |
do | |
echo "Created directory to $HOME/$d/$ACCOUNT" | |
mkdir -v -p $HOME/$d/$ACCOUNT | |
done | |
# Open browser to manually download other tools | |
# open https://www.jetbrains.com/lp/toolbox/ | |
# open https://notable.app | |
# open https://www.google.com/chrome | |
# open https://support.1password.com/getting-started-browser/ | |
# open https://docs.aws.amazon.com/cli/latest/userguide/cli-chap-install.html | |
# 1Password CLI - open https://app-updates.agilebits.com/product_history/CLI |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Here's a screenshot of the Ubuntu desktop after running this script.
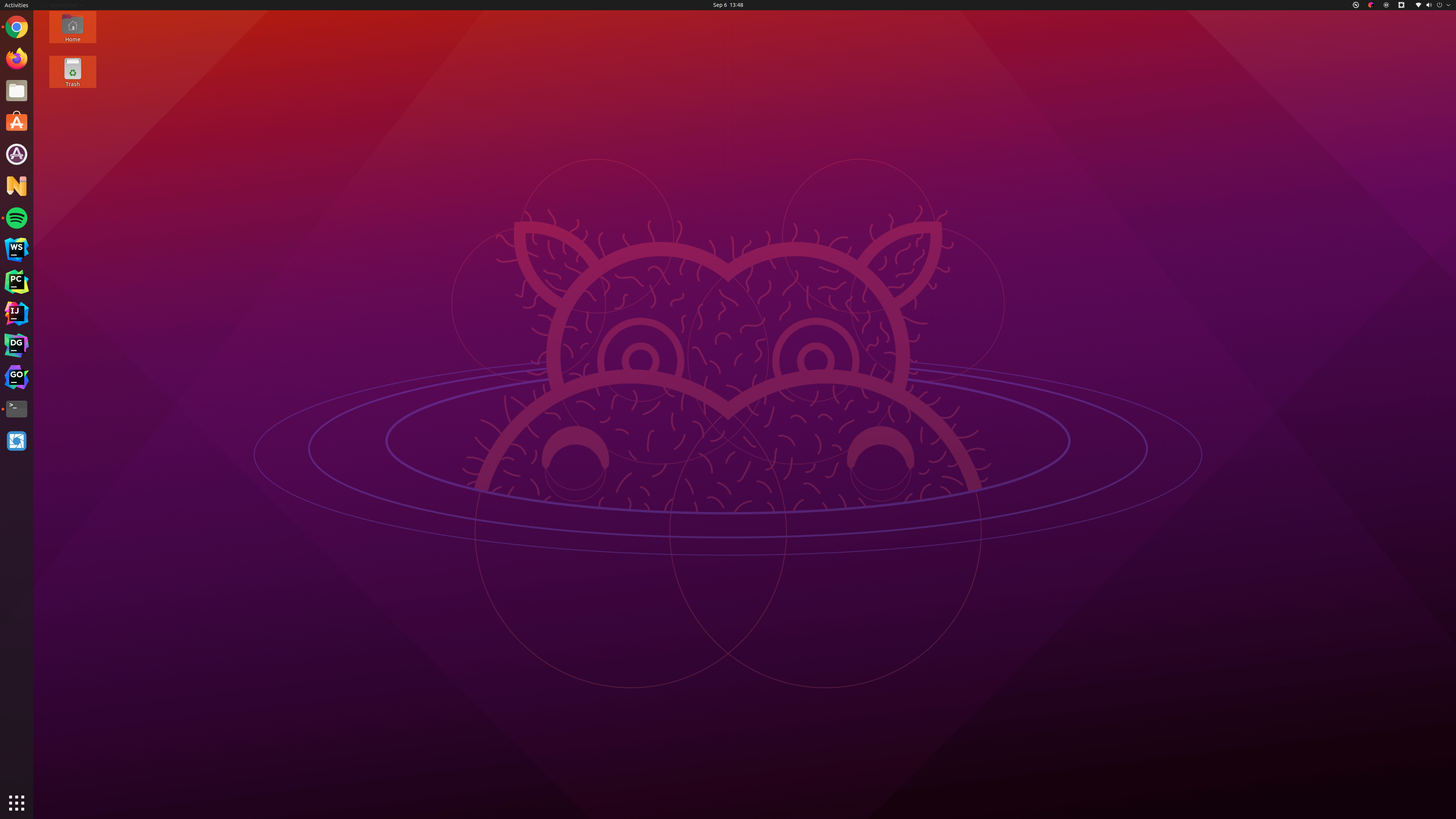