Last active
March 15, 2018 13:19
-
-
Save juliengdt/755d791bb8722a24f0b131a9ea156755 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import Foundation | |
import Result | |
import RxSwift | |
enum ResponseErrorType: Error, CustomStringConvertible { | |
case technical | |
case notFound | |
case other(message: Array<String>) | |
var description: String { | |
switch self { | |
case .technical: return "technical" | |
case .notFound: return "not found" | |
case .other(message: let strings): return strings.joined(separator: "\n") | |
} | |
} | |
} | |
protocol UnWrappable { | |
associatedtype U | |
var unwrap: Result<U,ResponseErrorType> { get } | |
} | |
struct ResponseError: Decodable, Error { | |
let property: String | |
let message: String | |
} | |
struct Response<T: Decodable>: Decodable { | |
let errors: Array<ResponseError>? | |
let message: String? | |
let result: T? | |
} | |
extension Response: ReactiveCompatible {} | |
extension Response: UnWrappable { | |
var unwrap: Result<T,ResponseErrorType> { | |
if let err = errors, err.count > 0 { | |
return Result<T, ResponseErrorType>.failure(.other(message: [err.description])) | |
} | |
if let res = result { | |
return Result<T, ResponseErrorType>.success(res) | |
} | |
return Result<T, ResponseErrorType>.failure(.technical) | |
} | |
} | |
extension Reactive where Base: UnWrappable { | |
var just: Observable<Base.U> { | |
switch base.unwrap { | |
case .success(let obj): | |
return Observable<Base.U>.just(obj) | |
case .failure(let err): | |
return Observable<Base.U>.error(err) | |
} | |
} | |
} | |
let resp: Response<String> = Response<String>(errors: [], message: "", result: "This is the result string") | |
resp.rx | |
.just | |
.subscribe(onNext: { next in | |
print("next element: \(next)") | |
}, onError: { err in | |
print("⚠️ error: \(err)") | |
}) |
Edit: Found it:
forgot to conform ReactiveCompatible
on Response
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Here is the deal:
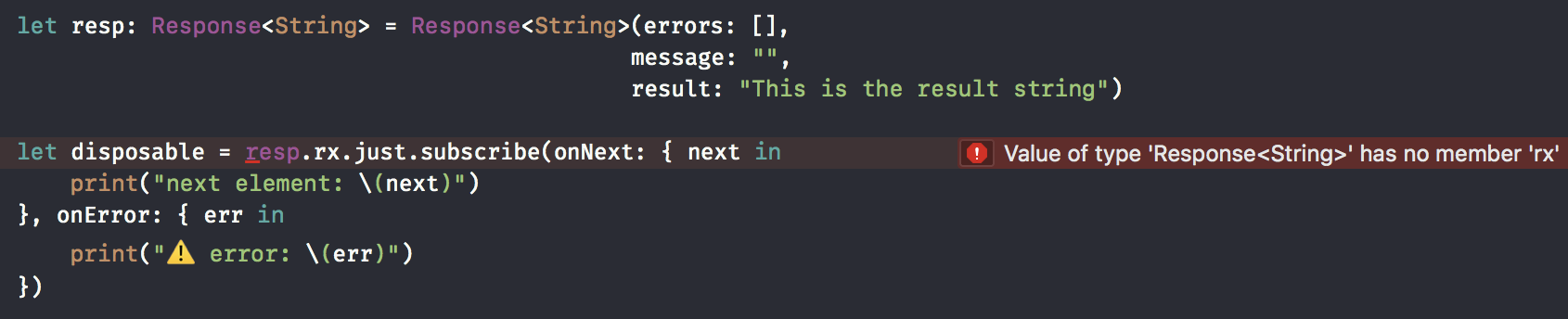