Created
August 21, 2023 09:36
-
-
Save kalimag/54e6364244d87dab02996d9fc609151c to your computer and use it in GitHub Desktop.
BizHawk API LuaCATS/LuaLS definitions
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
--Generated with BizHawk 2.9.2 | |
---@meta _ | |
---@class color : userdata | |
---A color in one of the following formats: | |
--- - Number in the format `0xAARRGGBB` | |
--- - String in the format `"#RRGGBB"` or `"#AARRGGBB"` | |
--- - A CSS3/X11 color name e.g. `"blue"`, `"palegoldenrod"` | |
--- - Color created with `forms.createcolor` | |
---@alias luacolor integer | string | color | |
---A library for performing standard bitwise operations. | |
---@class bit | |
bit = {} | |
---Arithmetic shift right of 'val' by 'amt' bits | |
---@param val integer | |
---@param amt integer | |
---@return integer | |
function bit.arshift(val, amt) end | |
---Bitwise AND of 'val' against 'amt' | |
---@deprecated | |
---@param val integer | |
---@param amt integer | |
---@return integer | |
function bit.band(val, amt) end | |
---Bitwise NOT of 'val' | |
---@deprecated | |
---@param val integer | |
---@return integer | |
function bit.bnot(val) end | |
---Bitwise OR of 'val' against 'amt' | |
---@deprecated | |
---@param val integer | |
---@param amt integer | |
---@return integer | |
function bit.bor(val, amt) end | |
---Bitwise XOR of 'val' against 'amt' | |
---@deprecated | |
---@param val integer | |
---@param amt integer | |
---@return integer | |
function bit.bxor(val, amt) end | |
---Byte swaps 'short', i.e. bit.byteswap_16(0xFF00) would return 0x00FF | |
---@param val integer | |
---@return integer | |
function bit.byteswap_16(val) end | |
---Byte swaps 'dword' | |
---@param val integer | |
---@return integer | |
function bit.byteswap_32(val) end | |
---Byte swaps 'long' | |
---@param val integer | |
---@return integer | |
function bit.byteswap_64(val) end | |
---Returns result of bit 'pos' being set in 'num' | |
---@param num integer | |
---@param pos integer | |
---@return boolean | |
function bit.check(num, pos) end | |
---Clears the bit 'pos' in 'num' | |
---@param num integer | |
---@param pos integer | |
---@return integer | |
function bit.clear(num, pos) end | |
---Logical shift left of 'val' by 'amt' bits | |
---@deprecated | |
---@param val integer | |
---@param amt integer | |
---@return integer | |
function bit.lshift(val, amt) end | |
---Left rotate 'val' by 'amt' bits | |
---@param val integer | |
---@param amt integer | |
---@return integer | |
function bit.rol(val, amt) end | |
---Right rotate 'val' by 'amt' bits | |
---@param val integer | |
---@param amt integer | |
---@return integer | |
function bit.ror(val, amt) end | |
---Logical shift right of 'val' by 'amt' bits | |
---@deprecated | |
---@param val integer | |
---@param amt integer | |
---@return integer | |
function bit.rshift(val, amt) end | |
---Sets the bit 'pos' in 'num' | |
---@param num integer | |
---@param pos integer | |
---@return integer | |
function bit.set(num, pos) end | |
---A library exposing standard .NET string methods | |
---@class bizstring | |
bizstring = {} | |
---Converts the number to a string representation of the binary value of the given number | |
---@param num integer | |
---@return string | |
function bizstring.binary(num) end | |
---Returns whether or not str contains str2 | |
---@param str string | |
---@param str2 string | |
---@return boolean | |
function bizstring.contains(str, str2) end | |
---Returns whether str ends wth str2 | |
---@param str string | |
---@param str2 string | |
---@return boolean | |
function bizstring.endswith(str, str2) end | |
---Converts the number to a string representation of the hexadecimal value of the given number | |
---@param num integer | |
---@return string | |
function bizstring.hex(num) end | |
---Converts the number to a string representation of the octal value of the given number | |
---@param num integer | |
---@return string | |
function bizstring.octal(num) end | |
---Appends zero or more of pad_char to the end (right) of str until it's at least length chars long. If pad_char is not a string exactly one char long, its first char will be used, or ' ' if it's empty. | |
---@param str string | |
---@param length integer | |
---@param pad_char string | |
---@return string | |
function bizstring.pad_end(str, length, pad_char) end | |
---Prepends zero or more of pad_char to the start (left) of str until it's at least length chars long. If pad_char is not a string exactly one char long, its first char will be used, or ' ' if it's empty. | |
---@param str string | |
---@param length integer | |
---@param pad_char string | |
---@return string | |
function bizstring.pad_start(str, length, pad_char) end | |
---Returns a string that represents str with the given position and count removed | |
---@param str string | |
---@param position integer | |
---@param count integer | |
---@return string | |
function bizstring.remove(str, position, count) end | |
---Returns a string that replaces all occurrences of str2 in str1 with the value of replace | |
---@param str string | |
---@param str2 string | |
---@param replace string | |
---@return string | |
function bizstring.replace(str, str2, replace) end | |
---Splits str into a Lua-style array using the given separator (consecutive separators in str will NOT create empty entries in the array). If the separator is not a string exactly one char long, ',' will be used. | |
---@param str string | |
---@param separator string | |
---@return table | |
function bizstring.split(str, separator) end | |
---Returns whether str starts with str2 | |
---@param str string | |
---@param str2 string | |
---@return boolean | |
function bizstring.startswith(str, str2) end | |
---Returns a string that represents a substring of str starting at position for the specified length | |
---@param str string | |
---@param position integer | |
---@param length integer | |
---@return string | |
function bizstring.substring(str, position, length) end | |
---Returns an lowercase version of the given string | |
---@param str string | |
---@return string | |
function bizstring.tolower(str) end | |
---Returns an uppercase version of the given string | |
---@param str string | |
---@return string | |
function bizstring.toupper(str) end | |
---returns a string that trims whitespace on the left and right ends of the string | |
---@param str string | |
---@return string | |
function bizstring.trim(str) end | |
---A library for manipulating the EmuHawk client UI | |
---@class client | |
client = {} | |
---adds a cheat code, if supported | |
---@param code string | |
function client.addcheat(code) end | |
---Gets the current height in pixels of the letter/pillarbox area (top side only) around the emu display surface, excluding the gameExtraPadding you've set. This function (the whole lot of them) should be renamed or refactored since the padding areas have got more complex. | |
---@return integer | |
function client.borderheight() end | |
---Gets the current width in pixels of the letter/pillarbox area (left side only) around the emu display surface, excluding the gameExtraPadding you've set. This function (the whole lot of them) should be renamed or refactored since the padding areas have got more complex. | |
---@return integer | |
function client.borderwidth() end | |
---Gets the visible height of the emu display surface (the core video output). This excludes the gameExtraPadding you've set. | |
---@return integer | |
function client.bufferheight() end | |
---Gets the visible width of the emu display surface (the core video output). This excludes the gameExtraPadding you've set. | |
---@return integer | |
function client.bufferwidth() end | |
---Clears all autohold keys | |
function client.clearautohold() end | |
---Closes the loaded Rom | |
function client.closerom() end | |
---returns a default instance of the given type of object if it exists (not case sensitive). Note: This will only work on objects which have a parameterless constructor. If no suitable type is found, or the type does not have a parameterless constructor, then nil is returned | |
---@param name string | |
---@return table | |
function client.createinstance(name) end | |
---sets whether or not on screen messages will display | |
---@param value boolean | |
function client.displaymessages(value) end | |
---Sets whether or not the rewind feature is enabled | |
---@param enabled boolean | |
function client.enablerewind(enabled) end | |
---sleeps exactly for n milliseconds | |
---@param millis integer | |
function client.exactsleep(millis) end | |
---Closes the emulator | |
function client.exit() end | |
---Closes the emulator and returns the provided code | |
---@param exitCode integer | |
function client.exitCode(exitCode) end | |
---Sets the frame skip value of the client UI (use 0 to disable) | |
---@param numFrames integer | |
function client.frameskip(numFrames) end | |
---Gets the (host) framerate, approximated from frame durations. | |
---@return integer | |
function client.get_approx_framerate() end | |
---returns the name of the Lua engine currently in use | |
---@return string | |
function client.get_lua_engine() end | |
---Returns a list of the tools currently open | |
---@return table | |
function client.getavailabletools() end | |
---gets the current config settings object | |
---@return any | |
function client.getconfig() end | |
---Gets the state of the Sound On toggle | |
---@return boolean | |
function client.GetSoundOn() end | |
---Gets the current scanline intensity setting, used for the scanline display filter | |
---@return integer | |
function client.gettargetscanlineintensity() end | |
---Returns an object that represents a tool of the given name (not case sensitive). If the tool is not open, it will be loaded if available. Use getavailabletools to get a list of names | |
---@param name string | |
---@return table | |
function client.gettool(name) end | |
---Returns the current stable BizHawk version | |
---@return string | |
function client.getversion() end | |
---Gets the main window's size Possible values are 1, 2, 3, 4, 5, and 10 | |
---@return integer | |
function client.getwindowsize() end | |
---Disables and enables emulator updates | |
---@param invisible boolean | |
function client.invisibleemulation(invisible) end | |
---Returns true if emulator is paused, otherwise, false | |
---@return boolean | |
function client.ispaused() end | |
---Returns true if emulator is seeking, otherwise, false | |
---@return boolean | |
function client.isseeking() end | |
---Returns true if emulator is in turbo mode, otherwise, false | |
---@return boolean | |
function client.isturbo() end | |
---opens the Cheats dialog | |
function client.opencheats() end | |
---opens the Hex Editor dialog | |
function client.openhexeditor() end | |
---opens the RAM Search dialog | |
function client.openramsearch() end | |
---opens the RAM Watch dialog | |
function client.openramwatch() end | |
---Loads a ROM from the given path. Returns true if the ROM was successfully loaded, otherwise false. | |
---@param path string | |
---@return boolean | |
function client.openrom(path) end | |
---opens the TAStudio dialog | |
function client.opentasstudio() end | |
---opens the Toolbox Dialog | |
function client.opentoolbox() end | |
---opens the tracelogger if it is available for the given core | |
function client.opentracelogger() end | |
---Pauses the emulator | |
function client.pause() end | |
---If currently capturing Audio/Video, this will suspend the record. Frames will not be captured into the AV until client.unpause_av() is called | |
function client.pause_av() end | |
---Reboots the currently loaded core | |
function client.reboot_core() end | |
---removes a cheat, if it already exists | |
---@param code string | |
function client.removecheat(code) end | |
---flushes save ram to disk | |
function client.saveram() end | |
---Gets the current height in pixels of the emulator's drawing area | |
---@return integer | |
function client.screenheight() end | |
---if a parameter is passed it will function as the Screenshot As menu item of EmuHawk, else it will function as the Screenshot menu item | |
---@param path? string | |
function client.screenshot(path) end | |
---Performs the same function as EmuHawk's Screenshot To Clipboard menu item | |
function client.screenshottoclipboard() end | |
---Gets the current width in pixels of the emulator's drawing area | |
---@return integer | |
function client.screenwidth() end | |
---Makes the emulator seek to the frame specified | |
---@param frame integer | |
function client.seekframe(frame) end | |
---Sets the extra padding added to the 'native' surface so that you can draw HUD elements in predictable placements | |
---@param left integer | |
---@param top integer | |
---@param right integer | |
---@param bottom integer | |
function client.SetClientExtraPadding(left, top, right, bottom) end | |
---Sets the extra padding added to the 'emu' surface so that you can draw HUD elements in predictable placements | |
---@param left integer | |
---@param top integer | |
---@param right integer | |
---@param bottom integer | |
function client.SetGameExtraPadding(left, top, right, bottom) end | |
---Sets the screenshot Capture OSD property of the client | |
---@param value boolean | |
function client.setscreenshotosd(value) end | |
---Sets the state of the Sound On toggle | |
---@param enable boolean | |
function client.SetSoundOn(enable) end | |
---Sets the current scanline intensity setting, used for the scanline display filter | |
---@param val integer | |
function client.settargetscanlineintensity(val) end | |
---Sets the main window's size to the give value. Accepted values are 1, 2, 3, 4, 5, and 10 | |
---@param size integer | |
function client.setwindowsize(size) end | |
---sleeps for n milliseconds | |
---@param millis integer | |
function client.sleep(millis) end | |
---Sets the speed of the emulator (in terms of percent) | |
---@param percent integer | |
function client.speedmode(percent) end | |
---Toggles the current pause state | |
function client.togglepause() end | |
---Transforms a point (x, y) in emulator space to a point in client space | |
---@param x integer | |
---@param y integer | |
---@return table | |
function client.transformPoint(x, y) end | |
---Unpauses the emulator | |
function client.unpause() end | |
---If currently capturing Audio/Video this resumes capturing | |
function client.unpause_av() end | |
---Returns the x value of the screen position where the client currently sits | |
---@return integer | |
function client.xpos() end | |
---Returns the y value of the screen position where the client currently sits | |
---@return integer | |
function client.ypos() end | |
---A library for communicating with other programs | |
---@class comm | |
comm = {} | |
---returns a list of implemented functions | |
---@return string | |
function comm.getluafunctionslist() end | |
---makes a HTTP GET request | |
---@param url string | |
---@return string | |
function comm.httpGet(url) end | |
---Gets HTTP GET URL | |
---@return string | |
function comm.httpGetGetUrl() end | |
---Gets HTTP POST URL | |
---@return string | |
function comm.httpGetPostUrl() end | |
---makes a HTTP POST request | |
---@param url string | |
---@param payload string | |
---@return string | |
function comm.httpPost(url, payload) end | |
---HTTP POST screenshot | |
---@return string | |
function comm.httpPostScreenshot() end | |
---Sets HTTP GET URL | |
---@param url string | |
function comm.httpSetGetUrl(url) end | |
---Sets HTTP POST URL | |
---@param url string | |
function comm.httpSetPostUrl(url) end | |
---Sets HTTP timeout in milliseconds | |
---@param timeout integer | |
function comm.httpSetTimeout(timeout) end | |
---tests HTTP connections | |
---@return string | |
function comm.httpTest() end | |
---tests the HTTP GET connection | |
---@return string | |
function comm.httpTestGet() end | |
---Copy a section of the memory to a memory mapped file | |
---@param mmf_filename string | |
---@param addr integer | |
---@param length integer | |
---@param domain string | |
---@return integer | |
function comm.mmfCopyFromMemory(mmf_filename, addr, length, domain) end | |
---Copy a memory mapped file to a section of the memory | |
---@param mmf_filename string | |
---@param addr integer | |
---@param length integer | |
---@param domain string | |
function comm.mmfCopyToMemory(mmf_filename, addr, length, domain) end | |
---Gets the filename for the screenshots | |
---@return string | |
function comm.mmfGetFilename() end | |
---Reads a string from a memory mapped file | |
---@param mmf_filename string | |
---@param expectedSize integer | |
---@return string | |
function comm.mmfRead(mmf_filename, expectedSize) end | |
---Reads bytes from a memory mapped file | |
---@param mmf_filename string | |
---@param expectedSize integer | |
---@return table | |
function comm.mmfReadBytes(mmf_filename, expectedSize) end | |
---Saves screenshot to memory mapped file | |
---@return integer | |
function comm.mmfScreenshot() end | |
---Sets the filename for the screenshots | |
---@param filename string | |
function comm.mmfSetFilename(filename) end | |
---Writes a string to a memory mapped file | |
---@param mmf_filename string | |
---@param outputString string | |
---@return integer | |
function comm.mmfWrite(mmf_filename, outputString) end | |
---Write bytes to a memory mapped file | |
---@param mmf_filename string | |
---@param byteArray table | |
---@return integer | |
function comm.mmfWriteBytes(mmf_filename, byteArray) end | |
---returns the IP and port of the Lua socket server | |
---@return string | |
function comm.socketServerGetInfo() end | |
---returns the IP address of the Lua socket server | |
---@return string | |
function comm.socketServerGetIp() end | |
---returns the port of the Lua socket server | |
---@return integer | |
function comm.socketServerGetPort() end | |
---socketServerIsConnected | |
---@return boolean | |
function comm.socketServerIsConnected() end | |
---Receives a message from the Socket server. Since BizHawk 2.6.2, all responses must be of the form $"{msg.Length:D} {msg}" i.e. prefixed with the length in base-10 and a space. | |
---@return string | |
function comm.socketServerResponse() end | |
---sends a screenshot to the Socket server | |
---@return string | |
function comm.socketServerScreenShot() end | |
---sends a screenshot to the Socket server and retrieves the response | |
---@return string | |
function comm.socketServerScreenShotResponse() end | |
---sends a string to the Socket server | |
---@param SendString string | |
---@return integer | |
function comm.socketServerSend(SendString) end | |
---sends bytes to the Socket server | |
---@param byteArray table | |
---@return integer | |
function comm.socketServerSendBytes(byteArray) end | |
---sets the IP address of the Lua socket server | |
---@param ip string | |
function comm.socketServerSetIp(ip) end | |
---sets the port of the Lua socket server | |
---@param port integer | |
function comm.socketServerSetPort(port) end | |
---sets the timeout in milliseconds for receiving messages | |
---@param timeout integer | |
function comm.socketServerSetTimeout(timeout) end | |
---returns the status of the last Socket server action | |
---@return boolean | |
function comm.socketServerSuccessful() end | |
---@class console | |
console = {} | |
---clears the output box of the Lua Console window | |
function console.clear() end | |
---returns a list of implemented functions | |
---@return string | |
function console.getluafunctionslist() end | |
---Outputs the given object to the output box on the Lua Console dialog. Note: Can accept a LuaTable | |
---@vararg any | |
function console.log(...) end | |
---Outputs the given object to the output box on the Lua Console dialog. Note: Can accept a LuaTable | |
---@vararg any | |
function console.write(...) end | |
---Outputs the given object to the output box on the Lua Console dialog. Note: Can accept a LuaTable | |
---@vararg any | |
function console.writeline(...) end | |
---A library for interacting with the currently loaded emulator core | |
---@class emu | |
emu = {} | |
---Returns the disassembly object (disasm string and length int) for the given PC address. Uses System Bus domain if no domain name provided | |
---@param pc integer | |
---@param name? string | |
---@return table | |
function emu.disassemble(pc, name) end | |
---Sets the display vsync property of the emulator | |
---@param enabled boolean | |
function emu.displayvsync(enabled) end | |
---Signals to the emulator to resume emulation. Necessary for any lua script while loop or else the emulator will freeze! | |
function emu.frameadvance() end | |
---Returns the current frame count | |
---@return integer | |
function emu.framecount() end | |
---returns (if available) the board name of the loaded ROM | |
---@return string | |
function emu.getboardname() end | |
---returns the display type (PAL vs NTSC) that the emulator is currently running in | |
---@return string | |
function emu.getdisplaytype() end | |
---returns the name of the Lua core currently in use | |
---@deprecated | |
---@return string | |
function emu.getluacore() end | |
---returns the value of a cpu register or flag specified by name. For a complete list of possible registers or flags for a given core, use getregisters | |
---@param name string | |
---@return integer | |
function emu.getregister(name) end | |
---returns the complete set of available flags and registers for a given core | |
---@return table | |
function emu.getregisters() end | |
---Returns the ID string of the current core loaded. Note: No ROM loaded will return the string NULL | |
---@return string | |
function emu.getsystemid() end | |
---Returns whether or not the current frame is a lag frame | |
---@return boolean | |
function emu.islagged() end | |
---Returns the current lag count | |
---@return integer | |
function emu.lagcount() end | |
---sets the limit framerate property of the emulator | |
---@param enabled boolean | |
function emu.limitframerate(enabled) end | |
---Sets the autominimizeframeskip value of the emulator | |
---@param enabled boolean | |
function emu.minimizeframeskip(enabled) end | |
---Sets the lag flag for the current frame. If no value is provided, it will default to true | |
---@param value? boolean | |
function emu.setislagged(value) end | |
---Sets the current lag count | |
---@param count integer | |
function emu.setlagcount(count) end | |
---sets the given register name to the given value | |
---@param register string | |
---@param value integer | |
function emu.setregister(register, value) end | |
---Toggles the drawing of sprites and background planes. Set to false or nil to disable a pane, anything else will draw them | |
---@vararg boolean | |
function emu.setrenderplanes(...) end | |
---gets the total number of executed cpu cycles | |
---@return integer | |
function emu.totalexecutedcycles() end | |
---allows a script to run while emulation is paused and interact with the gui/main window in realtime | |
function emu.yield() end | |
---A library for registering lua functions to emulator events. | |
--- All events support multiple registered methods. | |
---All registered event methods can be named and return a Guid when registered | |
---@class event | |
event = {} | |
---Lists the available scopes that can be specified for on_bus_* events | |
---@return table | |
function event.availableScopes() end | |
---Returns whether EmuHawk will pass arguments to callbacks. The current version passes arguments to "memory" callbacks (RAM/ROM/bus R/W), so this function will return true for that input. (It returns false for any other input.) This tells you whether it's necessary to enable workarounds/hacks because a script is running in a version without parameter support. | |
---@param subset? string | |
---@return boolean | |
function event.can_use_callback_params(subset) end | |
---Fires immediately before the given address is executed by the core. Your callback can have 3 parameters `(addr, val, flags)`. `val` is the value to be executed (or `0` always, if this feature is only partially implemented). | |
---@param luaf function | |
---@param address integer | |
---@param name? string | |
---@param scope? string | |
---@return string | |
function event.on_bus_exec(luaf, address, name, scope) end | |
---Fires immediately before every instruction executed (in the specified scope) by the core (CPU-intensive). Your callback can have 3 parameters `(addr, val, flags)`. `val` is the value to be executed (or `0` always, if this feature is only partially implemented). | |
---@param luaf function | |
---@param name? string | |
---@param scope? string | |
---@return string | |
function event.on_bus_exec_any(luaf, name, scope) end | |
---Fires immediately before the given address is read by the core. Your callback can have 3 parameters `(addr, val, flags)`. `val` is the value read. If no address is given, it will fire on every memory read. | |
---@param luaf function | |
---@param address? integer | |
---@param name? string | |
---@param scope? string | |
---@return string | |
function event.on_bus_read(luaf, address, name, scope) end | |
---Fires immediately before the given address is written by the core. Your callback can have 3 parameters `(addr, val, flags)`. `val` is the value to be written (or `0` always, if this feature is only partially implemented). If no address is given, it will fire on every memory write. | |
---@param luaf function | |
---@param address? integer | |
---@param name? string | |
---@param scope? string | |
---@return string | |
function event.on_bus_write(luaf, address, name, scope) end | |
---Fires when the emulator console closes | |
---@param luaf function | |
---@param name? string | |
---@return string | |
function event.onconsoleclose(luaf, name) end | |
---Fires after the calling script has stopped | |
---@param luaf function | |
---@param name? string | |
---@return string | |
function event.onexit(luaf, name) end | |
---Calls the given lua function at the end of each frame, after all emulation and drawing has completed. Note: this is the default behavior of lua scripts | |
---@param luaf function | |
---@param name? string | |
---@return string | |
function event.onframeend(luaf, name) end | |
---Calls the given lua function at the beginning of each frame before any emulation and drawing occurs | |
---@param luaf function | |
---@param name? string | |
---@return string | |
function event.onframestart(luaf, name) end | |
---Calls the given lua function after each time the emulator core polls for input | |
---@param luaf function | |
---@param name? string | |
---@return string | |
function event.oninputpoll(luaf, name) end | |
---Fires after a state is loaded. Your callback can have 1 parameter, which will be the name of the loaded state. | |
---@param luaf function | |
---@param name? string | |
---@return string | |
function event.onloadstate(luaf, name) end | |
---Fires immediately before the given address is executed by the core. Your callback can have 3 parameters `(addr, val, flags)`. `val` is the value to be executed (or `0` always, if this feature is only partially implemented). | |
---@deprecated | |
---@param luaf function | |
---@param address integer | |
---@param name? string | |
---@param scope? string | |
---@return string | |
function event.onmemoryexecute(luaf, address, name, scope) end | |
---Fires immediately before every instruction executed (in the specified scope) by the core (CPU-intensive). Your callback can have 3 parameters `(addr, val, flags)`. `val` is the value to be executed (or `0` always, if this feature is only partially implemented). | |
---@deprecated | |
---@param luaf function | |
---@param name? string | |
---@param scope? string | |
---@return string | |
function event.onmemoryexecuteany(luaf, name, scope) end | |
---Fires immediately before the given address is read by the core. Your callback can have 3 parameters `(addr, val, flags)`. `val` is the value read. If no address is given, it will fire on every memory read. | |
---@deprecated | |
---@param luaf function | |
---@param address? integer | |
---@param name? string | |
---@param scope? string | |
---@return string | |
function event.onmemoryread(luaf, address, name, scope) end | |
---Fires immediately before the given address is written by the core. Your callback can have 3 parameters `(addr, val, flags)`. `val` is the value to be written (or `0` always, if this feature is only partially implemented). If no address is given, it will fire on every memory write. | |
---@deprecated | |
---@param luaf function | |
---@param address? integer | |
---@param name? string | |
---@param scope? string | |
---@return string | |
function event.onmemorywrite(luaf, address, name, scope) end | |
---Fires after a state is saved. Your callback can have 1 parameter, which will be the name of the saved state. | |
---@param luaf function | |
---@param name? string | |
---@return string | |
function event.onsavestate(luaf, name) end | |
---Removes the registered function that matches the guid. If a function is found and remove the function will return true. If unable to find a match, the function will return false. | |
---@param guid string | |
---@return boolean | |
function event.unregisterbyid(guid) end | |
---Removes the first registered function that matches Name. If a function is found and remove the function will return true. If unable to find a match, the function will return false. | |
---@param name string | |
---@return boolean | |
function event.unregisterbyname(name) end | |
---A library for creating and managing custom dialogs | |
---@class forms | |
forms = {} | |
---adds the given lua function as a click event to the given control | |
---@param handle integer | |
---@param clickEvent function | |
function forms.addclick(handle, clickEvent) end | |
---Creates a button control on the given form. The caption property will be the text value on the button. clickEvent is the name of a Lua function that will be invoked when the button is clicked. x, and y are the optional location parameters for the position of the button within the given form. The function returns the handle of the created button. Width and Height are optional, if not specified they will be a default size | |
---@param formHandle integer | |
---@param caption string | number | |
---@param clickEvent function | |
---@param x? integer | |
---@param y? integer | |
---@param width? integer | |
---@param height? integer | |
---@return integer | |
function forms.button(formHandle, caption, clickEvent, x, y, width, height) end | |
---Creates a checkbox control on the given form. The caption property will be the text of the checkbox. x and y are the optional location parameters for the position of the checkbox within the form | |
---@param formHandle integer | |
---@param caption string | number | |
---@param x? integer | |
---@param y? integer | |
---@return integer | |
function forms.checkbox(formHandle, caption, x, y) end | |
---Clears the canvas | |
---@param componentHandle integer | |
---@param color luacolor | |
function forms.clear(componentHandle, color) end | |
---Removes all click events from the given widget at the specified handle | |
---@param handle integer | |
function forms.clearclicks(handle) end | |
---clears the image cache that is built up by using gui.drawImage, also releases the file handle for cached images | |
---@param componentHandle integer | |
function forms.clearImageCache(componentHandle) end | |
---Creates a color object useful with setproperty | |
---@param r integer | |
---@param g integer | |
---@param b integer | |
---@param a integer | |
---@return color | |
function forms.createcolor(r, g, b, a) end | |
---Closes and removes a Lua created form with the specified handle. If a dialog was found and removed true is returned, else false | |
---@param handle integer | |
---@return boolean | |
function forms.destroy(handle) end | |
---Closes and removes all Lua created dialogs | |
function forms.destroyall() end | |
---draws a Arc shape at the given coordinates and the given width and height | |
---@param componentHandle integer | |
---@param x integer | |
---@param y integer | |
---@param width integer | |
---@param height integer | |
---@param startangle integer | |
---@param sweepangle integer | |
---@param line? luacolor | |
function forms.drawArc(componentHandle, x, y, width, height, startangle, sweepangle, line) end | |
---Draws an axis of the specified size at the coordinate pair.) | |
---@param componentHandle integer | |
---@param x integer | |
---@param y integer | |
---@param size integer | |
---@param color? luacolor | |
function forms.drawAxis(componentHandle, x, y, size, color) end | |
---Draws a Bezier curve using the table of coordinates provided in the given color | |
---@param componentHandle integer | |
---@param points table | |
---@param color luacolor | |
function forms.drawBezier(componentHandle, points, color) end | |
---Draws a rectangle on screen from x1/y1 to x2/y2. Same as drawRectangle except it receives two points intead of a point and width/height | |
---@param componentHandle integer | |
---@param x integer | |
---@param y integer | |
---@param x2 integer | |
---@param y2 integer | |
---@param line? luacolor | |
---@param background? luacolor | |
function forms.drawBox(componentHandle, x, y, x2, y2, line, background) end | |
---Draws an ellipse at the given coordinates and the given width and height. Line is the color of the ellipse. Background is the optional fill color | |
---@param componentHandle integer | |
---@param x integer | |
---@param y integer | |
---@param width integer | |
---@param height integer | |
---@param line? luacolor | |
---@param background? luacolor | |
function forms.drawEllipse(componentHandle, x, y, width, height, line, background) end | |
---draws an Icon (.ico) file from the given path at the given coordinate. width and height are optional. If specified, it will resize the image accordingly | |
---@param componentHandle integer | |
---@param path string | |
---@param x integer | |
---@param y integer | |
---@param width? integer | |
---@param height? integer | |
function forms.drawIcon(componentHandle, path, x, y, width, height) end | |
---draws an image file from the given path at the given coordinate. width and height are optional. If specified, it will resize the image accordingly | |
---@param componentHandle integer | |
---@param path string | |
---@param x integer | |
---@param y integer | |
---@param width? integer | |
---@param height? integer | |
---@param cache? boolean | |
function forms.drawImage(componentHandle, path, x, y, width, height, cache) end | |
---Draws a region of the given image file at the given location on the canvas, and optionally resizes it before drawing. Consult this diagram to see its usage (renders embedded on the TASVideos Wiki): 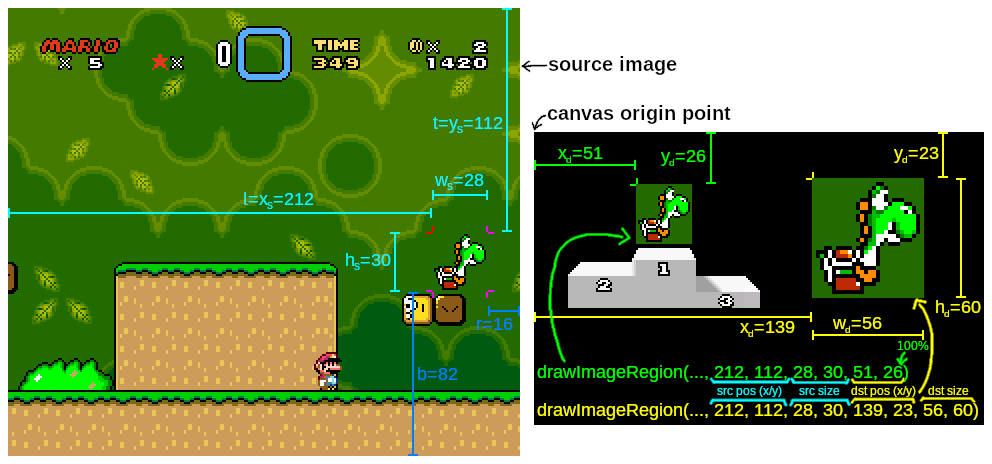 | |
---@param componentHandle integer | |
---@param path string | |
---@param source_x integer | |
---@param source_y integer | |
---@param source_width integer | |
---@param source_height integer | |
---@param dest_x integer | |
---@param dest_y integer | |
---@param dest_width? integer | |
---@param dest_height? integer | |
function forms.drawImageRegion(componentHandle, path, source_x, source_y, source_width, source_height, dest_x, dest_y, dest_width, dest_height) end | |
---Draws a line from the first coordinate pair to the 2nd. Color is optional (if not specified it will be drawn black) | |
---@param componentHandle integer | |
---@param x1 integer | |
---@param y1 integer | |
---@param x2 integer | |
---@param y2 integer | |
---@param color? luacolor | |
function forms.drawLine(componentHandle, x1, y1, x2, y2, color) end | |
---draws a Pie shape at the given coordinates and the given width and height | |
---@param componentHandle integer | |
---@param x integer | |
---@param y integer | |
---@param width integer | |
---@param height integer | |
---@param startangle integer | |
---@param sweepangle integer | |
---@param line? luacolor | |
---@param background? luacolor | |
function forms.drawPie(componentHandle, x, y, width, height, startangle, sweepangle, line, background) end | |
---Draws a single pixel at the given coordinates in the given color. Color is optional (if not specified it will be drawn black) | |
---@param componentHandle integer | |
---@param x integer | |
---@param y integer | |
---@param color? luacolor | |
function forms.drawPixel(componentHandle, x, y, color) end | |
---Draws a polygon using the table of coordinates specified in points. This should be a table of tables(each of size 2). If x or y is passed, the polygon will be translated by the passed coordinate pair. Line is the color of the polygon. Background is the optional fill color | |
---@param componentHandle integer | |
---@param points table | |
---@param x? integer | |
---@param y? integer | |
---@param line? luacolor | |
---@param background? luacolor | |
function forms.drawPolygon(componentHandle, points, x, y, line, background) end | |
---Draws a rectangle at the given coordinate and the given width and height. Line is the color of the box. Background is the optional fill color | |
---@param componentHandle integer | |
---@param x integer | |
---@param y integer | |
---@param width integer | |
---@param height integer | |
---@param line? luacolor | |
---@param background? luacolor | |
function forms.drawRectangle(componentHandle, x, y, width, height, line, background) end | |
---Alias of DrawText() | |
---@param componentHandle integer | |
---@param x integer | |
---@param y integer | |
---@param message string | number | |
---@param forecolor? luacolor | |
---@param backcolor? luacolor | |
---@param fontsize? integer | |
---@param fontfamily? string | |
---@param fontstyle? string | |
---@param horizalign? string | |
---@param vertalign? string | |
function forms.drawString(componentHandle, x, y, message, forecolor, backcolor, fontsize, fontfamily, fontstyle, horizalign, vertalign) end | |
---Draws the given message at the given x,y coordinates and the given color. The default color is white. A fontfamily can be specified and is monospace generic if none is specified (font family options are the same as the .NET FontFamily class). The fontsize default is 12. The default font style is regular. Font style options are regular, bold, italic, strikethrough, underline. Horizontal alignment options are left (default), center, or right. Vertical alignment options are bottom (default), middle, or top. Alignment options specify which ends of the text will be drawn at the x and y coordinates. | |
---@param componentHandle integer | |
---@param x integer | |
---@param y integer | |
---@param message string | number | |
---@param forecolor? luacolor | |
---@param backcolor? luacolor | |
---@param fontsize? integer | |
---@param fontfamily? string | |
---@param fontstyle? string | |
---@param horizalign? string | |
---@param vertalign? string | |
function forms.drawText(componentHandle, x, y, message, forecolor, backcolor, fontsize, fontfamily, fontstyle, horizalign, vertalign) end | |
---Creates a dropdown (with a ComboBoxStyle of DropDownList) control on the given form. Dropdown items are passed via a lua table. Only the values will be pulled for the dropdown items, the keys are irrelevant. Items will be sorted alphabetically. x and y are the optional location parameters, and width and height are the optional size parameters. | |
---@param formHandle integer | |
---@param items table | |
---@param x? integer | |
---@param y? integer | |
---@param width? integer | |
---@param height? integer | |
---@return integer | |
function forms.dropdown(formHandle, items, x, y, width, height) end | |
---Returns an integer representation of the mouse X coordinate relative to the PictureBox. | |
---@param componentHandle integer | |
---@return integer | |
function forms.getMouseX(componentHandle) end | |
---Returns an integer representation of the mouse Y coordinate relative to the PictureBox. | |
---@param componentHandle integer | |
---@return integer | |
function forms.getMouseY(componentHandle) end | |
---returns a string representation of the value of a property of the widget at the given handle | |
---@param handle integer | |
---@param property string | |
---@return string | |
function forms.getproperty(handle, property) end | |
---Returns the text property of a given form or control | |
---@param handle integer | |
---@return string | |
function forms.gettext(handle) end | |
---Returns the given checkbox's checked property | |
---@param handle integer | |
---@return boolean | |
function forms.ischecked(handle) end | |
---Creates a label control on the given form. The caption property is the text of the label. x, and y are the optional location parameters for the position of the label within the given form. The function returns the handle of the created label. Width and Height are optional, if not specified they will be a default size. | |
---@param formHandle integer | |
---@param caption string | number | |
---@param x? integer | |
---@param y? integer | |
---@param width? integer | |
---@param height? integer | |
---@param fixedWidth? boolean | |
---@return integer | |
function forms.label(formHandle, caption, x, y, width, height, fixedWidth) end | |
---creates a new default dialog, if both width and height are specified it will create a dialog of the specified size. If title is specified it will be the caption of the dialog, else the dialog caption will be 'Lua Dialog'. The function will return an int representing the handle of the dialog created. | |
---@param width? integer | |
---@param height? integer | |
---@param title? string | |
---@param onClose? function | |
---@return integer | |
function forms.newform(width, height, title, onClose) end | |
---Creates a standard openfile dialog with optional parameters for the filename, directory, and filter. The return value is the directory that the user picked. If they chose to cancel, it will return an empty string | |
---@param fileName? string | |
---@param initialDirectory? string | |
---@param filter? string | |
---@return string | |
function forms.openfile(fileName, initialDirectory, filter) end | |
---Creates a new drawing area in the form. Optionally the location in the form as well as the size of the drawing area can be specified. Returns the handle the component can be refered to with. | |
---@param formHandle integer | |
---@param x? integer | |
---@param y? integer | |
---@param width? integer | |
---@param height? integer | |
---@return integer | |
function forms.pictureBox(formHandle, x, y, width, height) end | |
---Redraws the canvas | |
---@param componentHandle integer | |
function forms.refresh(componentHandle) end | |
---Sets the default background color to use in drawing methods, transparent by default | |
---@param componentHandle integer | |
---@param color luacolor | |
function forms.setDefaultBackgroundColor(componentHandle, color) end | |
---Sets the default foreground color to use in drawing methods, white by default | |
---@param componentHandle integer | |
---@param color luacolor | |
function forms.setDefaultForegroundColor(componentHandle, color) end | |
---Sets the default backgroiund color to use in text drawing methods, half-transparent black by default | |
---@param componentHandle integer | |
---@param color luacolor | |
function forms.setDefaultTextBackground(componentHandle, color) end | |
---Updates the item list of a dropdown menu. The optional third parameter toggles alphabetical sorting of items, pass false to skip sorting. | |
---@param handle integer | |
---@param items table | |
---@param alphabetize? boolean | |
function forms.setdropdownitems(handle, items, alphabetize) end | |
---Sets the location of a control or form by passing in the handle of the created object | |
---@param handle integer | |
---@param x integer | |
---@param y integer | |
function forms.setlocation(handle, x, y) end | |
---Attempts to set the given property of the widget with the given value. Note: not all properties will be able to be represented for the control to accept | |
---@param handle integer | |
---@param property string | |
---@param value any | |
function forms.setproperty(handle, property, value) end | |
---TODO | |
---@param handle integer | |
---@param width integer | |
---@param height integer | |
function forms.setsize(handle, width, height) end | |
---Sets the text property of a control or form by passing in the handle of the created object | |
---@param handle integer | |
---@param caption string | number | |
function forms.settext(handle, caption) end | |
---Creates a textbox control on the given form. The caption property will be the initial value of the textbox (default is empty). Width and Height are option, if not specified they will be a default size of 100, 20. Type is an optional property to restrict the textbox input. The available options are HEX, SIGNED, and UNSIGNED. Passing it null or any other value will set it to no restriction. x, and y are the optional location parameters for the position of the textbox within the given form. The function returns the handle of the created textbox. If true, the multiline will enable the standard winform multi-line property. If true, the fixedWidth options will create a fixed width font. Scrollbars is an optional property to specify which scrollbars to display. The available options are Vertical, Horizontal, Both, and None. Scrollbars are only shown on a multiline textbox | |
---@param formHandle integer | |
---@param caption? string | number | |
---@param width? integer | |
---@param height? integer | |
---@param boxtype? string | |
---@param x? integer | |
---@param y? integer | |
---@param multiline? boolean | |
---@param fixedWidth? boolean | |
---@param scrollbars? string | |
---@return integer | |
function forms.textbox(formHandle, caption, width, height, boxtype, x, y, multiline, fixedWidth, scrollbars) end | |
---@class gameinfo | |
gameinfo = {} | |
---returns identifying information about the 'mapper' or similar capability used for this game. empty if no such useful distinction can be drawn | |
---@return string | |
function gameinfo.getboardtype() end | |
---returns the game options for the currently loaded rom. Options vary per platform | |
---@return table | |
function gameinfo.getoptions() end | |
---returns the hash of the currently loaded rom, if a rom is loaded | |
---@return string | |
function gameinfo.getromhash() end | |
---returns the name of the currently loaded rom, if a rom is loaded | |
---@return string | |
function gameinfo.getromname() end | |
---returns the game database status of the currently loaded rom. Statuses are for example: GoodDump, BadDump, Hack, Unknown, NotInDatabase | |
---@return string | |
function gameinfo.getstatus() end | |
---returns whether or not the currently loaded rom is in the game database | |
---@return boolean | |
function gameinfo.indatabase() end | |
---returns the currently loaded rom's game database status is considered 'bad' | |
---@return boolean | |
function gameinfo.isstatusbad() end | |
---Functions specific to GenesisHawk (functions may not run when an Genesis game is not loaded) | |
---@class genesis | |
genesis = {} | |
---Returns whether the bg layer A is displayed | |
---@return boolean | |
function genesis.getlayer_bga() end | |
---Returns whether the bg layer B is displayed | |
---@return boolean | |
function genesis.getlayer_bgb() end | |
---Returns whether the bg layer W is displayed | |
---@return boolean | |
function genesis.getlayer_bgw() end | |
---Sets whether the bg layer A is displayed | |
---@param value boolean | |
function genesis.setlayer_bga(value) end | |
---Sets whether the bg layer B is displayed | |
---@param value boolean | |
function genesis.setlayer_bgb(value) end | |
---Sets whether the bg layer W is displayed | |
---@param value boolean | |
function genesis.setlayer_bgw(value) end | |
---@class gui | |
gui = {} | |
---Adds a message to the OSD's message area | |
---@param message string | number | |
function gui.addmessage(message) end | |
---clears all lua drawn graphics from the screen | |
---@param surfaceName? string | |
function gui.clearGraphics(surfaceName) end | |
---clears the image cache that is built up by using gui.drawImage, also releases the file handle for cached images | |
function gui.clearImageCache() end | |
---clears all text created by gui.text() | |
function gui.cleartext() end | |
---Creates a canvas of the given size and, if specified, the given coordinates. | |
---@param width integer | |
---@param height integer | |
---@param x? integer | |
---@param y? integer | |
---@return table | |
function gui.createcanvas(width, height, x, y) end | |
---Sets the default background color to use in drawing methods, transparent by default | |
---@param color luacolor | |
function gui.defaultBackground(color) end | |
---Sets the default foreground color to use in drawing methods, white by default | |
---@param color luacolor | |
function gui.defaultForeground(color) end | |
---Sets the default font to use in gui.pixelText(). Two font families are available, "fceux" and "gens" (or "0" and "1" respectively), "gens" is used by default | |
---@param fontfamily string | |
function gui.defaultPixelFont(fontfamily) end | |
---Sets the default background color to use in text drawing methods, half-transparent black by default | |
---@param color luacolor | |
function gui.defaultTextBackground(color) end | |
---Draws an axis of the specified size at the coordinate pair.) | |
---@param x integer | |
---@param y integer | |
---@param size integer | |
---@param color? luacolor | |
---@param surfaceName? string | |
function gui.drawAxis(x, y, size, color, surfaceName) end | |
---Draws a Bezier curve using the table of coordinates provided in the given color | |
---@param points table | |
---@param color luacolor | |
---@param surfaceName? string | |
function gui.drawBezier(points, color, surfaceName) end | |
---Draws a rectangle on screen from x1/y1 to x2/y2. Same as drawRectangle except it receives two points intead of a point and width/height | |
---@param x integer | |
---@param y integer | |
---@param x2 integer | |
---@param y2 integer | |
---@param line? luacolor | |
---@param background? luacolor | |
---@param surfaceName? string | |
function gui.drawBox(x, y, x2, y2, line, background, surfaceName) end | |
---Draws an ellipse at the given coordinates and the given width and height. Line is the color of the ellipse. Background is the optional fill color | |
---@param x integer | |
---@param y integer | |
---@param width integer | |
---@param height integer | |
---@param line? luacolor | |
---@param background? luacolor | |
---@param surfaceName? string | |
function gui.drawEllipse(x, y, width, height, line, background, surfaceName) end | |
---Finishes drawing to the current lua surface and causes it to get displayed. | |
---@deprecated | |
function gui.DrawFinish() end | |
---draws an Icon (.ico) file from the given path at the given coordinate. width and height are optional. If specified, it will resize the image accordingly | |
---@param path string | |
---@param x integer | |
---@param y integer | |
---@param width? integer | |
---@param height? integer | |
---@param surfaceName? string | |
function gui.drawIcon(path, x, y, width, height, surfaceName) end | |
---draws an image file from the given path at the given coordinate. width and height are optional. If specified, it will resize the image accordingly | |
---@param path string | |
---@param x integer | |
---@param y integer | |
---@param width? integer | |
---@param height? integer | |
---@param cache? boolean | |
---@param surfaceName? string | |
function gui.drawImage(path, x, y, width, height, cache, surfaceName) end | |
---draws a given region of an image file from the given path at the given coordinate, and optionally with the given size | |
---@param path string | |
---@param source_x integer | |
---@param source_y integer | |
---@param source_width integer | |
---@param source_height integer | |
---@param dest_x integer | |
---@param dest_y integer | |
---@param dest_width? integer | |
---@param dest_height? integer | |
---@param surfaceName? string | |
function gui.drawImageRegion(path, source_x, source_y, source_width, source_height, dest_x, dest_y, dest_width, dest_height, surfaceName) end | |
---Draws a line from the first coordinate pair to the 2nd. Color is optional (if not specified it will be drawn black) | |
---@param x1 integer | |
---@param y1 integer | |
---@param x2 integer | |
---@param y2 integer | |
---@param color? luacolor | |
---@param surfaceName? string | |
function gui.drawLine(x1, y1, x2, y2, color, surfaceName) end | |
---Changes drawing target to the specified lua surface name. This may clobber any previous drawing to this surface (pass false if you don't want it to) | |
---@deprecated | |
---@param name string | |
---@param clear? boolean | |
function gui.DrawNew(name, clear) end | |
---draws a Pie shape at the given coordinates and the given width and height | |
---@param x integer | |
---@param y integer | |
---@param width integer | |
---@param height integer | |
---@param startangle integer | |
---@param sweepangle integer | |
---@param line? luacolor | |
---@param background? luacolor | |
---@param surfaceName? string | |
function gui.drawPie(x, y, width, height, startangle, sweepangle, line, background, surfaceName) end | |
---Draws a single pixel at the given coordinates in the given color. Color is optional (if not specified it will be drawn black) | |
---@param x integer | |
---@param y integer | |
---@param color? luacolor | |
---@param surfaceName? string | |
function gui.drawPixel(x, y, color, surfaceName) end | |
---Draws a polygon using the table of coordinates specified in points. This should be a table of tables(each of size 2). If x or y is passed, the polygon will be translated by the passed coordinate pair. Line is the color of the polygon. Background is the optional fill color | |
---@param points table | |
---@param offsetX? integer | |
---@param offsetY? integer | |
---@param line? luacolor | |
---@param background? luacolor | |
---@param surfaceName? string | |
function gui.drawPolygon(points, offsetX, offsetY, line, background, surfaceName) end | |
---Draws a rectangle at the given coordinate and the given width and height. Line is the color of the box. Background is the optional fill color | |
---@param x integer | |
---@param y integer | |
---@param width integer | |
---@param height integer | |
---@param line? luacolor | |
---@param background? luacolor | |
---@param surfaceName? string | |
function gui.drawRectangle(x, y, width, height, line, background, surfaceName) end | |
---Draws the given message in the emulator screen space (like all draw functions) at the given x,y coordinates and the given color. The default color is white. A fontfamily can be specified and is monospace generic if none is specified (font family options are the same as the .NET FontFamily class). The fontsize default is 12. The default font style is regular. Font style options are regular, bold, italic, strikethrough, underline. Horizontal alignment options are left (default), center, or right. Vertical alignment options are bottom (default), middle, or top. Alignment options specify which ends of the text will be drawn at the x and y coordinates. For pixel-perfect font look, make sure to disable aspect ratio correction. | |
---@param x integer | |
---@param y integer | |
---@param message string | number | |
---@param forecolor? luacolor | |
---@param backcolor? luacolor | |
---@param fontsize? integer | |
---@param fontfamily? string | |
---@param fontstyle? string | |
---@param horizalign? string | |
---@param vertalign? string | |
---@param surfaceName? string | |
function gui.drawString(x, y, message, forecolor, backcolor, fontsize, fontfamily, fontstyle, horizalign, vertalign, surfaceName) end | |
---alias for gui.drawString | |
---@param x integer | |
---@param y integer | |
---@param message string | number | |
---@param forecolor? luacolor | |
---@param backcolor? luacolor | |
---@param fontsize? integer | |
---@param fontfamily? string | |
---@param fontstyle? string | |
---@param horizalign? string | |
---@param vertalign? string | |
---@param surfaceName? string | |
function gui.drawText(x, y, message, forecolor, backcolor, fontsize, fontfamily, fontstyle, horizalign, vertalign, surfaceName) end | |
---Draws the given message in the emulator screen space (like all draw functions) at the given x,y coordinates and the given color. The default color is white. Two font families are available, "fceux" and "gens" (or "0" and "1" respectively), both are monospace and have the same size as in the emulators they've been taken from. If no font family is specified, it uses "gens" font, unless that's overridden via gui.defaultPixelFont() | |
---@param x integer | |
---@param y integer | |
---@param message string | number | |
---@param forecolor? luacolor | |
---@param backcolor? luacolor | |
---@param fontfamily? string | |
---@param surfaceName? string | |
function gui.pixelText(x, y, message, forecolor, backcolor, fontfamily, surfaceName) end | |
---Displays the given text on the screen at the given coordinates. Optional Foreground color. The optional anchor flag anchors the text to one of the four corners. Anchor flag parameters: topleft, topright, bottomleft, bottomright | |
---@param x integer | |
---@param y integer | |
---@param message string | number | |
---@param forecolor? luacolor | |
---@param anchor? string | |
function gui.text(x, y, message, forecolor, anchor) end | |
---Stores the name of a surface to draw on, so you don't need to pass it to every draw function. The default is "emucore", and the other valid value is "client". | |
---@param surfaceName string | |
function gui.use_surface(surfaceName) end | |
---@class input | |
input = {} | |
---Returns a lua table of all the buttons the user is currently pressing on their keyboard and gamepads | |
---All buttons that are pressed have their key values set to true; all others remain nil. | |
---@return table | |
function input.get() end | |
---Returns a lua table of the mouse X/Y coordinates and button states. Table keys are X, Y, Left, Middle, Right, XButton1, XButton2, Wheel. | |
---@return table | |
function input.getmouse() end | |
---@class joypad | |
joypad = {} | |
---returns a lua table of the controller buttons pressed. If supplied, it will only return a table of buttons for the given controller | |
---@param controller? integer | |
---@return table | |
function joypad.get(controller) end | |
---returns a lua table of any controller buttons currently pressed by the user | |
---@param controller? integer | |
---@return table | |
function joypad.getimmediate(controller) end | |
---returns a lua table of the controller buttons pressed, including ones pressed by the current movie. If supplied, it will only return a table of buttons for the given controller | |
---@param controller? integer | |
---@return table | |
function joypad.getwithmovie(controller) end | |
---sets the given buttons to their provided values for the current frame | |
---@param buttons table | |
---@param controller? integer | |
function joypad.set(buttons, controller) end | |
---sets the given analog controls to their provided values for the current frame. Note that unlike set() there is only the logic of overriding with the given value. | |
---@param controls table | |
---@param controller? integer | |
function joypad.setanalog(controls, controller) end | |
---sets the given buttons to their provided values for the current frame, string will be interpreted the same way an entry from a movie input log would be | |
---@param inputLogEntry string | |
function joypad.setfrommnemonicstr(inputLogEntry) end | |
---Represents a canvas object returned by the gui.createcanvas() method | |
---@class LuaCanvas | |
LuaCanvas = {} | |
---Clears the canvas | |
---@param color luacolor | |
function LuaCanvas.Clear(color) end | |
---clears the image cache that is built up by using gui.drawImage, also releases the file handle for cached images | |
function LuaCanvas.ClearImageCache() end | |
---draws a Arc shape at the given coordinates and the given width and height | |
---@param x integer | |
---@param y integer | |
---@param width integer | |
---@param height integer | |
---@param startAngle integer | |
---@param sweepAngle integer | |
---@param line? luacolor | |
function LuaCanvas.DrawArc(x, y, width, height, startAngle, sweepAngle, line) end | |
---Draws an axis of the specified size at the coordinate pair.) | |
---@param x integer | |
---@param y integer | |
---@param size integer | |
---@param color? luacolor | |
function LuaCanvas.DrawAxis(x, y, size, color) end | |
---Draws a Bezier curve using the table of coordinates provided in the given color | |
---@param points table | |
---@param color luacolor | |
function LuaCanvas.DrawBezier(points, color) end | |
---Draws a rectangle on screen from x1/y1 to x2/y2. Same as drawRectangle except it receives two points intead of a point and width/height | |
---@param x integer | |
---@param y integer | |
---@param x2 integer | |
---@param y2 integer | |
---@param line? luacolor | |
---@param background? luacolor | |
function LuaCanvas.DrawBox(x, y, x2, y2, line, background) end | |
---Draws an ellipse at the given coordinates and the given width and height. Line is the color of the ellipse. Background is the optional fill color | |
---@param x integer | |
---@param y integer | |
---@param width integer | |
---@param height integer | |
---@param line? luacolor | |
---@param background? luacolor | |
function LuaCanvas.DrawEllipse(x, y, width, height, line, background) end | |
---draws an Icon (.ico) file from the given path at the given coordinate. width and height are optional. If specified, it will resize the image accordingly | |
---@param path string | |
---@param x integer | |
---@param y integer | |
---@param width? integer | |
---@param height? integer | |
function LuaCanvas.DrawIcon(path, x, y, width, height) end | |
---draws an image file from the given path at the given coordinate. width and height are optional. If specified, it will resize the image accordingly | |
---@param path string | |
---@param x integer | |
---@param y integer | |
---@param width? integer | |
---@param height? integer | |
---@param cache? boolean | |
function LuaCanvas.DrawImage(path, x, y, width, height, cache) end | |
---draws a given region of an image file from the given path at the given coordinate, and optionally with the given size | |
---@param path string | |
---@param sourceX integer | |
---@param sourceY integer | |
---@param sourceWidth integer | |
---@param sourceHeight integer | |
---@param destX integer | |
---@param destY integer | |
---@param destWidth? integer | |
---@param destHeight? integer | |
function LuaCanvas.DrawImageRegion(path, sourceX, sourceY, sourceWidth, sourceHeight, destX, destY, destWidth, destHeight) end | |
---Draws a line from the first coordinate pair to the 2nd. Color is optional (if not specified it will be drawn black) | |
---@param x1 integer | |
---@param y1 integer | |
---@param x2 integer | |
---@param y2 integer | |
---@param color? luacolor | |
function LuaCanvas.DrawLine(x1, y1, x2, y2, color) end | |
---draws a Pie shape at the given coordinates and the given width and height | |
---@param x integer | |
---@param y integer | |
---@param width integer | |
---@param height integer | |
---@param startAngle integer | |
---@param sweepAngle integer | |
---@param line? luacolor | |
---@param background? luacolor | |
function LuaCanvas.DrawPie(x, y, width, height, startAngle, sweepAngle, line, background) end | |
---Draws a single pixel at the given coordinates in the given color. Color is optional (if not specified it will be drawn black) | |
---@param x integer | |
---@param y integer | |
---@param color? luacolor | |
function LuaCanvas.DrawPixel(x, y, color) end | |
---Draws a polygon using the table of coordinates specified in points. This should be a table of tables(each of size 2). Line is the color of the polygon. Background is the optional fill color | |
---@param points table | |
---@param x? integer | |
---@param y? integer | |
---@param line? luacolor | |
---@param background? luacolor | |
function LuaCanvas.DrawPolygon(points, x, y, line, background) end | |
---Draws a rectangle at the given coordinate and the given width and height. Line is the color of the box. Background is the optional fill color | |
---@param x integer | |
---@param y integer | |
---@param width integer | |
---@param height integer | |
---@param line? luacolor | |
---@param background? luacolor | |
function LuaCanvas.DrawRectangle(x, y, width, height, line, background) end | |
---Alias of DrawText() | |
---@param x integer | |
---@param y integer | |
---@param message string | number | |
---@param foreColor? luacolor | |
---@param backColor? luacolor | |
---@param fontSize? integer | |
---@param fontFamily? string | |
---@param fontStyle? string | |
---@param horizontalAlign? string | |
---@param verticalAlign? string | |
function LuaCanvas.DrawString(x, y, message, foreColor, backColor, fontSize, fontFamily, fontStyle, horizontalAlign, verticalAlign) end | |
---Draws the given message at the given x,y coordinates and the given color. The default color is white. A fontfamily can be specified and is monospace generic if none is specified (font family options are the same as the .NET FontFamily class). The fontsize default is 12. The default font style is regular. Font style options are regular, bold, italic, strikethrough, underline. Horizontal alignment options are left (default), center, or right. Vertical alignment options are bottom (default), middle, or top. Alignment options specify which ends of the text will be drawn at the x and y coordinates. | |
---@param x integer | |
---@param y integer | |
---@param message string | number | |
---@param foreColor? luacolor | |
---@param backColor? luacolor | |
---@param fontSize? integer | |
---@param fontFamily? string | |
---@param fontStyle? string | |
---@param horizontalAlign? string | |
---@param verticalAlign? string | |
function LuaCanvas.DrawText(x, y, message, foreColor, backColor, fontSize, fontFamily, fontStyle, horizontalAlign, verticalAlign) end | |
---Returns an integer representation of the mouse X coordinate relative to the canvas window. | |
---@return integer | |
function LuaCanvas.GetMouseX() end | |
---Returns an integer representation of the mouse Y coordinate relative to the canvas window. | |
---@return integer | |
function LuaCanvas.GetMouseY() end | |
---Redraws the canvas | |
function LuaCanvas.Refresh() end | |
---Saves everything that's been drawn to a .png file at the given path. Relative paths are relative to the path set for "Screenshots" for the current system. | |
---@param path string | |
function LuaCanvas.save_image_to_disk(path) end | |
---Sets the default background color to use in drawing methods, transparent by default | |
---@param color luacolor | |
function LuaCanvas.SetDefaultBackgroundColor(color) end | |
---Sets the default foreground color to use in drawing methods, white by default | |
---@param color luacolor | |
function LuaCanvas.SetDefaultForegroundColor(color) end | |
---Sets the default background color to use in text drawing methods, half-transparent black by default | |
---@param color luacolor | |
function LuaCanvas.SetDefaultTextBackground(color) end | |
---Sets the location of the canvas window | |
---@param x integer | |
---@param y integer | |
function LuaCanvas.SetLocation(x, y) end | |
---Sets the canvas window title | |
---@param title string | |
function LuaCanvas.SetTitle(title) end | |
---Main memory library reads and writes from the Main memory domain (the default memory domain set by any given core) | |
---@class mainmemory | |
mainmemory = {} | |
---Returns the number of bytes of the domain defined as main memory | |
---@return integer | |
function mainmemory.getcurrentmemorydomainsize() end | |
---returns the name of the domain defined as main memory for the given core | |
---@return string | |
function mainmemory.getname() end | |
---Reads length bytes starting at addr into an array-like table (1-indexed). | |
---@param addr integer | |
---@param length integer | |
---@return table | |
function mainmemory.read_bytes_as_array(addr, length) end | |
---Reads length bytes starting at addr into a dict-like table (where the keys are the addresses, relative to the start of the main memory). | |
---@param addr integer | |
---@param length integer | |
---@return table | |
function mainmemory.read_bytes_as_dict(addr, length) end | |
---read signed 2 byte value, big endian | |
---@param addr integer | |
---@return integer | |
function mainmemory.read_s16_be(addr) end | |
---read signed 2 byte value, little endian | |
---@param addr integer | |
---@return integer | |
function mainmemory.read_s16_le(addr) end | |
---read signed 24 bit value, big endian | |
---@param addr integer | |
---@return integer | |
function mainmemory.read_s24_be(addr) end | |
---read signed 24 bit value, little endian | |
---@param addr integer | |
---@return integer | |
function mainmemory.read_s24_le(addr) end | |
---read signed 4 byte value, big endian | |
---@param addr integer | |
---@return integer | |
function mainmemory.read_s32_be(addr) end | |
---read signed 4 byte value, little endian | |
---@param addr integer | |
---@return integer | |
function mainmemory.read_s32_le(addr) end | |
---read signed byte | |
---@param addr integer | |
---@return integer | |
function mainmemory.read_s8(addr) end | |
---read unsigned 2 byte value, big endian | |
---@param addr integer | |
---@return integer | |
function mainmemory.read_u16_be(addr) end | |
---read unsigned 2 byte value, little endian | |
---@param addr integer | |
---@return integer | |
function mainmemory.read_u16_le(addr) end | |
---read unsigned 24 bit value, big endian | |
---@param addr integer | |
---@return integer | |
function mainmemory.read_u24_be(addr) end | |
---read unsigned 24 bit value, little endian | |
---@param addr integer | |
---@return integer | |
function mainmemory.read_u24_le(addr) end | |
---read unsigned 4 byte value, big endian | |
---@param addr integer | |
---@return integer | |
function mainmemory.read_u32_be(addr) end | |
---read unsigned 4 byte value, little endian | |
---@param addr integer | |
---@return integer | |
function mainmemory.read_u32_le(addr) end | |
---read unsigned byte | |
---@param addr integer | |
---@return integer | |
function mainmemory.read_u8(addr) end | |
---gets the value from the given address as an unsigned byte | |
---@param addr integer | |
---@return integer | |
function mainmemory.readbyte(addr) end | |
---Reads the address range that starts from address, and is length long. Returns a zero-indexed table containing the read values (an array of bytes.) | |
---@deprecated | |
---@param addr integer | |
---@param length integer | |
---@return table | |
function mainmemory.readbyterange(addr, length) end | |
---Reads the given address as a 32-bit float value from the main memory domain with th e given endian | |
---@param addr integer | |
---@param bigendian boolean | |
---@return number | |
function mainmemory.readfloat(addr, bigendian) end | |
---Writes sequential bytes starting at addr. | |
---@param addr integer | |
---@param bytes table | |
function mainmemory.write_bytes_as_array(addr, bytes) end | |
---Writes bytes at arbitrary addresses (the keys of the given table are the addresses, relative to the start of the main memory). | |
---@param addrMap table | |
function mainmemory.write_bytes_as_dict(addrMap) end | |
---write signed 2 byte value, big endian | |
---@param addr integer | |
---@param value integer | |
function mainmemory.write_s16_be(addr, value) end | |
---write signed 2 byte value, little endian | |
---@param addr integer | |
---@param value integer | |
function mainmemory.write_s16_le(addr, value) end | |
---write signed 24 bit value, big endian | |
---@param addr integer | |
---@param value integer | |
function mainmemory.write_s24_be(addr, value) end | |
---write signed 24 bit value, little endian | |
---@param addr integer | |
---@param value integer | |
function mainmemory.write_s24_le(addr, value) end | |
---write signed 4 byte value, big endian | |
---@param addr integer | |
---@param value integer | |
function mainmemory.write_s32_be(addr, value) end | |
---write signed 4 byte value, little endian | |
---@param addr integer | |
---@param value integer | |
function mainmemory.write_s32_le(addr, value) end | |
---write signed byte | |
---@param addr integer | |
---@param value integer | |
function mainmemory.write_s8(addr, value) end | |
---write unsigned 2 byte value, big endian | |
---@param addr integer | |
---@param value integer | |
function mainmemory.write_u16_be(addr, value) end | |
---write unsigned 2 byte value, little endian | |
---@param addr integer | |
---@param value integer | |
function mainmemory.write_u16_le(addr, value) end | |
---write unsigned 24 bit value, big endian | |
---@param addr integer | |
---@param value integer | |
function mainmemory.write_u24_be(addr, value) end | |
---write unsigned 24 bit value, little endian | |
---@param addr integer | |
---@param value integer | |
function mainmemory.write_u24_le(addr, value) end | |
---write unsigned 4 byte value, big endian | |
---@param addr integer | |
---@param value integer | |
function mainmemory.write_u32_be(addr, value) end | |
---write unsigned 4 byte value, little endian | |
---@param addr integer | |
---@param value integer | |
function mainmemory.write_u32_le(addr, value) end | |
---write unsigned byte | |
---@param addr integer | |
---@param value integer | |
function mainmemory.write_u8(addr, value) end | |
---Writes the given value to the given address as an unsigned byte | |
---@param addr integer | |
---@param value integer | |
function mainmemory.writebyte(addr, value) end | |
---Writes the given values to the given addresses as unsigned bytes | |
---@deprecated | |
---@param memoryblock table | |
function mainmemory.writebyterange(memoryblock) end | |
---Writes the given 32-bit float value to the given address and endian | |
---@param addr integer | |
---@param value number | |
---@param bigendian boolean | |
function mainmemory.writefloat(addr, value, bigendian) end | |
---These functions behavior identically to the mainmemory functions but the user can set the memory domain to read and write from. The default domain is the system bus. Use getcurrentmemorydomain(), and usememorydomain() to control which domain is used. Each core has its own set of valid memory domains. Use getmemorydomainlist() to get a list of memory domains for the current core loaded. | |
---@class memory | |
memory = {} | |
---Returns a string name of the current memory domain selected by Lua. The default is Main memory | |
---@return string | |
function memory.getcurrentmemorydomain() end | |
---Returns the number of bytes of the current memory domain selected by Lua. The default is Main memory | |
---@return integer | |
function memory.getcurrentmemorydomainsize() end | |
---Returns a string of the memory domains for the loaded platform core. List will be a single string delimited by line feeds | |
---@return table | |
function memory.getmemorydomainlist() end | |
---Returns the number of bytes of the specified memory domain. If no domain is specified, or the specified domain doesn't exist, returns the current domain size | |
---@param name? string | |
---@return integer | |
function memory.getmemorydomainsize(name) end | |
---Returns a hash as a string of a region of memory, starting from addr, through count bytes. If the domain is unspecified, it uses the current region. | |
---@param addr integer | |
---@param count integer | |
---@param domain? string | |
---@return string | |
function memory.hash_region(addr, count, domain) end | |
---Reads length bytes starting at addr into an array-like table (1-indexed). | |
---@param addr integer | |
---@param length integer | |
---@param domain? string | |
---@return table | |
function memory.read_bytes_as_array(addr, length, domain) end | |
---Reads length bytes starting at addr into a dict-like table (where the keys are the addresses, relative to the start of the domain). | |
---@param addr integer | |
---@param length integer | |
---@param domain? string | |
---@return table | |
function memory.read_bytes_as_dict(addr, length, domain) end | |
---read signed 2 byte value, big endian | |
---@param addr integer | |
---@param domain? string | |
---@return integer | |
function memory.read_s16_be(addr, domain) end | |
---read signed 2 byte value, little endian | |
---@param addr integer | |
---@param domain? string | |
---@return integer | |
function memory.read_s16_le(addr, domain) end | |
---read signed 24 bit value, big endian | |
---@param addr integer | |
---@param domain? string | |
---@return integer | |
function memory.read_s24_be(addr, domain) end | |
---read signed 24 bit value, little endian | |
---@param addr integer | |
---@param domain? string | |
---@return integer | |
function memory.read_s24_le(addr, domain) end | |
---read signed 4 byte value, big endian | |
---@param addr integer | |
---@param domain? string | |
---@return integer | |
function memory.read_s32_be(addr, domain) end | |
---read signed 4 byte value, little endian | |
---@param addr integer | |
---@param domain? string | |
---@return integer | |
function memory.read_s32_le(addr, domain) end | |
---read signed byte | |
---@param addr integer | |
---@param domain? string | |
---@return integer | |
function memory.read_s8(addr, domain) end | |
---read unsigned 2 byte value, big endian | |
---@param addr integer | |
---@param domain? string | |
---@return integer | |
function memory.read_u16_be(addr, domain) end | |
---read unsigned 2 byte value, little endian | |
---@param addr integer | |
---@param domain? string | |
---@return integer | |
function memory.read_u16_le(addr, domain) end | |
---read unsigned 24 bit value, big endian | |
---@param addr integer | |
---@param domain? string | |
---@return integer | |
function memory.read_u24_be(addr, domain) end | |
---read unsigned 24 bit value, little endian | |
---@param addr integer | |
---@param domain? string | |
---@return integer | |
function memory.read_u24_le(addr, domain) end | |
---read unsigned 4 byte value, big endian | |
---@param addr integer | |
---@param domain? string | |
---@return integer | |
function memory.read_u32_be(addr, domain) end | |
---read unsigned 4 byte value, little endian | |
---@param addr integer | |
---@param domain? string | |
---@return integer | |
function memory.read_u32_le(addr, domain) end | |
---read unsigned byte | |
---@param addr integer | |
---@param domain? string | |
---@return integer | |
function memory.read_u8(addr, domain) end | |
---gets the value from the given address as an unsigned byte | |
---@param addr integer | |
---@param domain? string | |
---@return integer | |
function memory.readbyte(addr, domain) end | |
---Reads the address range that starts from address, and is length long. Returns a zero-indexed table containing the read values (an array of bytes.) | |
---@deprecated | |
---@param addr integer | |
---@param length integer | |
---@param domain? string | |
---@return table | |
function memory.readbyterange(addr, length, domain) end | |
---Reads the given address as a 32-bit float value from the main memory domain with th e given endian | |
---@param addr integer | |
---@param bigendian boolean | |
---@param domain? string | |
---@return number | |
function memory.readfloat(addr, bigendian, domain) end | |
---Attempts to set the current memory domain to the given domain. If the name does not match a valid memory domain, the function returns false, else it returns true | |
---@param domain string | |
---@return boolean | |
function memory.usememorydomain(domain) end | |
---Writes sequential bytes starting at addr. | |
---@param addr integer | |
---@param bytes table | |
---@param domain? string | |
function memory.write_bytes_as_array(addr, bytes, domain) end | |
---Writes bytes at arbitrary addresses (the keys of the given table are the addresses, relative to the start of the domain). | |
---@param addrMap table | |
---@param domain? string | |
function memory.write_bytes_as_dict(addrMap, domain) end | |
---write signed 2 byte value, big endian | |
---@param addr integer | |
---@param value integer | |
---@param domain? string | |
function memory.write_s16_be(addr, value, domain) end | |
---write signed 2 byte value, little endian | |
---@param addr integer | |
---@param value integer | |
---@param domain? string | |
function memory.write_s16_le(addr, value, domain) end | |
---write signed 24 bit value, big endian | |
---@param addr integer | |
---@param value integer | |
---@param domain? string | |
function memory.write_s24_be(addr, value, domain) end | |
---write signed 24 bit value, little endian | |
---@param addr integer | |
---@param value integer | |
---@param domain? string | |
function memory.write_s24_le(addr, value, domain) end | |
---write signed 4 byte value, big endian | |
---@param addr integer | |
---@param value integer | |
---@param domain? string | |
function memory.write_s32_be(addr, value, domain) end | |
---write signed 4 byte value, little endian | |
---@param addr integer | |
---@param value integer | |
---@param domain? string | |
function memory.write_s32_le(addr, value, domain) end | |
---write signed byte | |
---@param addr integer | |
---@param value integer | |
---@param domain? string | |
function memory.write_s8(addr, value, domain) end | |
---write unsigned 2 byte value, big endian | |
---@param addr integer | |
---@param value integer | |
---@param domain? string | |
function memory.write_u16_be(addr, value, domain) end | |
---write unsigned 2 byte value, little endian | |
---@param addr integer | |
---@param value integer | |
---@param domain? string | |
function memory.write_u16_le(addr, value, domain) end | |
---write unsigned 24 bit value, big endian | |
---@param addr integer | |
---@param value integer | |
---@param domain? string | |
function memory.write_u24_be(addr, value, domain) end | |
---write unsigned 24 bit value, little endian | |
---@param addr integer | |
---@param value integer | |
---@param domain? string | |
function memory.write_u24_le(addr, value, domain) end | |
---write unsigned 4 byte value, big endian | |
---@param addr integer | |
---@param value integer | |
---@param domain? string | |
function memory.write_u32_be(addr, value, domain) end | |
---write unsigned 4 byte value, little endian | |
---@param addr integer | |
---@param value integer | |
---@param domain? string | |
function memory.write_u32_le(addr, value, domain) end | |
---write unsigned byte | |
---@param addr integer | |
---@param value integer | |
---@param domain? string | |
function memory.write_u8(addr, value, domain) end | |
---Writes the given value to the given address as an unsigned byte | |
---@param addr integer | |
---@param value integer | |
---@param domain? string | |
function memory.writebyte(addr, value, domain) end | |
---Writes the given values to the given addresses as unsigned bytes | |
---@deprecated | |
---@param memoryblock table | |
---@param domain? string | |
function memory.writebyterange(memoryblock, domain) end | |
---Writes the given 32-bit float value to the given address and endian | |
---@param addr integer | |
---@param value number | |
---@param bigendian boolean | |
---@param domain? string | |
function memory.writefloat(addr, value, bigendian, domain) end | |
---@class memorysavestate | |
memorysavestate = {} | |
---clears all savestates stored in memory | |
function memorysavestate.clearstatesfrommemory() end | |
---loads an in memory state with the given identifier | |
---@param identifier string | |
function memorysavestate.loadcorestate(identifier) end | |
---removes the savestate with the given identifier from memory | |
---@param identifier string | |
function memorysavestate.removestate(identifier) end | |
---creates a core savestate and stores it in memory. Note: a core savestate is only the raw data from the core, and not extras such as movie input logs, or framebuffers. Returns a unique identifer for the savestate | |
---@return string | |
function memorysavestate.savecorestate() end | |
---@class movie | |
movie = {} | |
---Returns the file name including path of the currently loaded movie | |
---@return string | |
function movie.filename() end | |
---If a movie is active, will return the movie comments as a lua table | |
---@return table | |
function movie.getcomments() end | |
---If a movie is loaded, gets the frames per second used by the movie to determine the movie length time | |
---@return number | |
function movie.getfps() end | |
---If a movie is active, will return the movie header as a lua table | |
---@return table | |
function movie.getheader() end | |
---Returns a table of buttons pressed on a given frame of the loaded movie | |
---@param frame integer | |
---@param controller? integer | |
---@return table | |
function movie.getinput(frame, controller) end | |
---Returns the input of a given frame of the loaded movie in a raw inputlog string | |
---@param frame integer | |
---@return string | |
function movie.getinputasmnemonic(frame) end | |
---Returns true if the movie is in read-only mode, false if in read+write | |
---@return boolean | |
function movie.getreadonly() end | |
---Gets the rerecord count of the current movie. | |
---@return integer | |
function movie.getrerecordcount() end | |
---Returns whether or not the current movie is incrementing rerecords on loadstate | |
---@return boolean | |
function movie.getrerecordcounting() end | |
---If a movie is active, will return the movie subtitles as a lua table | |
---@return table | |
function movie.getsubtitles() end | |
---Returns true if a movie is loaded in memory (play, record, or finished modes), false if not (inactive mode) | |
---@return boolean | |
function movie.isloaded() end | |
---Returns the total number of frames of the loaded movie | |
---@return integer | |
function movie.length() end | |
---Returns the mode of the current movie. Possible modes: "PLAY", "RECORD", "FINISHED", "INACTIVE" | |
---@return string | |
function movie.mode() end | |
---Resets the core to frame 0 with the currently loaded movie in playback mode. If a path to a movie is specified, attempts to load it, then continues with playback if it was successful. Returns true iff successful. | |
---@param path? string | |
---@return boolean | |
function movie.play_from_start(path) end | |
---Saves the current movie to the disc. If the filename is provided (no extension or path needed), the movie is saved under the specified name to the current movie directory. The filename may contain a subdirectory, it will be created if it doesn't exist. Existing files won't get overwritten. | |
---@param filename? string | |
function movie.save(filename) end | |
---Sets the read-only state to the given value. true for read only, false for read+write | |
---@param readOnly boolean | |
function movie.setreadonly(readOnly) end | |
---Sets the rerecord count of the current movie. | |
---@param count integer | |
function movie.setrerecordcount(count) end | |
---Sets whether or not the current movie will increment the rerecord counter on loadstate | |
---@param counting boolean | |
function movie.setrerecordcounting(counting) end | |
---Returns whether or not the movie is a saveram-anchored movie | |
---@return boolean | |
function movie.startsfromsaveram() end | |
---Returns whether or not the movie is a savestate-anchored movie | |
---@return boolean | |
function movie.startsfromsavestate() end | |
---Stops the current movie. Pass false to discard changes. | |
---@param saveChanges? boolean | |
function movie.stop(saveChanges) end | |
---Functions specific to NDSHawk (functions may not run when an NDS game is not loaded) | |
---@class nds | |
nds = {} | |
---Returns the audio bitrate setting | |
---@return string | |
function nds.getaudiobitrate() end | |
---Returns the gap between the screens | |
---@return integer | |
function nds.getscreengap() end | |
---Returns whether screens are inverted | |
---@return boolean | |
function nds.getscreeninvert() end | |
---Returns which screen layout is active | |
---@return string | |
function nds.getscreenlayout() end | |
---Returns how screens are rotated | |
---@return string | |
function nds.getscreenrotation() end | |
---Sets the audio bitrate setting | |
---@param value string | |
function nds.setaudiobitrate(value) end | |
---Sets the gap between the screens | |
---@param value integer | |
function nds.setscreengap(value) end | |
---Sets whether screens are inverted | |
---@param value boolean | |
function nds.setscreeninvert(value) end | |
---Sets which screen layout is active | |
---@param value string | |
function nds.setscreenlayout(value) end | |
---Sets how screens are rotated | |
---@param value string | |
function nds.setscreenrotation(value) end | |
---Functions related specifically to Nes Cores | |
---@class nes | |
nes = {} | |
---Gets the NES setting 'Allow more than 8 sprites per scanline' value | |
---@return boolean | |
function nes.getallowmorethaneightsprites() end | |
---Gets the current value for the bottom scanline value | |
---@param pal? boolean | |
---@return integer | |
function nes.getbottomscanline(pal) end | |
---Gets the current value for the Clip Left and Right sides option | |
---@return boolean | |
function nes.getclipleftandright() end | |
---Indicates whether or not the bg layer is being displayed | |
---@return boolean | |
function nes.getdispbackground() end | |
---Indicates whether or not sprites are being displayed | |
---@return boolean | |
function nes.getdispsprites() end | |
---Gets the current value for the top scanline value | |
---@param pal? boolean | |
---@return integer | |
function nes.gettopscanline(pal) end | |
---Sets the NES setting 'Allow more than 8 sprites per scanline' | |
---@param allow boolean | |
function nes.setallowmorethaneightsprites(allow) end | |
---Sets the Clip Left and Right sides option | |
---@param leftandright boolean | |
function nes.setclipleftandright(leftandright) end | |
---Sets whether or not the background layer will be displayed | |
---@param show boolean | |
function nes.setdispbackground(show) end | |
---Sets whether or not sprites will be displayed | |
---@param show boolean | |
function nes.setdispsprites(show) end | |
---sets the top and bottom scanlines to be drawn (same values as in the graphics options dialog). Top must be in the range of 0 to 127, bottom must be between 128 and 239. Not supported in the Quick Nes core | |
---@param top integer | |
---@param bottom integer | |
---@param pal? boolean | |
function nes.setscanlines(top, bottom, pal) end | |
---@class savestate | |
savestate = {} | |
---Loads a savestate with the given path. Returns true iff succeeded. If EmuHawk is deferring quicksaves, to TAStudio for example, that form will do what it likes (and the path is ignored). | |
---@param path string | |
---@param suppressOSD? boolean | |
---@return boolean | |
function savestate.load(path, suppressOSD) end | |
---Loads the savestate at the given slot number (must be an integer between 1 and 10). Returns true iff succeeded. If EmuHawk is deferring quicksaves, to TAStudio for example, that form will do what it likes with the slot number. | |
---@param slotNum integer | |
---@param suppressOSD? boolean | |
---@return boolean | |
function savestate.loadslot(slotNum, suppressOSD) end | |
---Saves a state at the given path. If EmuHawk is deferring quicksaves, to TAStudio for example, that form will do what it likes (and the path is ignored). | |
---@param path string | |
---@param suppressOSD? boolean | |
function savestate.save(path, suppressOSD) end | |
---Saves a state at the given save slot (must be an integer between 1 and 10). If EmuHawk is deferring quicksaves, to TAStudio for example, that form will do what it likes with the slot number. | |
---@param slotNum integer | |
---@param suppressOSD? boolean | |
function savestate.saveslot(slotNum, suppressOSD) end | |
---Functions specific to SNESHawk (functions may not run when an SNES game is not loaded) | |
---@class snes | |
snes = {} | |
---Returns whether the bg 1 layer is displayed | |
---@return boolean | |
function snes.getlayer_bg_1() end | |
---Returns whether the bg 2 layer is displayed | |
---@return boolean | |
function snes.getlayer_bg_2() end | |
---Returns whether the bg 3 layer is displayed | |
---@return boolean | |
function snes.getlayer_bg_3() end | |
---Returns whether the bg 4 layer is displayed | |
---@return boolean | |
function snes.getlayer_bg_4() end | |
---Returns whether the obj 1 layer is displayed | |
---@return boolean | |
function snes.getlayer_obj_1() end | |
---Returns whether the obj 2 layer is displayed | |
---@return boolean | |
function snes.getlayer_obj_2() end | |
---Returns whether the obj 3 layer is displayed | |
---@return boolean | |
function snes.getlayer_obj_3() end | |
---Returns whether the obj 4 layer is displayed | |
---@return boolean | |
function snes.getlayer_obj_4() end | |
---Sets whether the bg 1 layer is displayed | |
---@param value boolean | |
function snes.setlayer_bg_1(value) end | |
---Sets whether the bg 2 layer is displayed | |
---@param value boolean | |
function snes.setlayer_bg_2(value) end | |
---Sets whether the bg 3 layer is displayed | |
---@param value boolean | |
function snes.setlayer_bg_3(value) end | |
---Sets whether the bg 4 layer is displayed | |
---@param value boolean | |
function snes.setlayer_bg_4(value) end | |
---Sets whether the obj 1 layer is displayed | |
---@param value boolean | |
function snes.setlayer_obj_1(value) end | |
---Sets whether the obj 2 layer is displayed | |
---@param value boolean | |
function snes.setlayer_obj_2(value) end | |
---Sets whether the obj 3 layer is displayed | |
---@param value boolean | |
function snes.setlayer_obj_3(value) end | |
---Sets whether the obj 4 layer is displayed | |
---@param value boolean | |
function snes.setlayer_obj_4(value) end | |
---A library for performing SQLite operations. | |
---@class SQL | |
SQL = {} | |
---Creates a SQLite Database. Name should end with .db | |
---@param name string | |
---@return string | |
function SQL.createdatabase(name) end | |
---Opens a SQLite database. Name should end with .db | |
---@param name string | |
---@return string | |
function SQL.opendatabase(name) end | |
---Run a SQLite read command which includes Select. Returns all rows into a LuaTable.Ex: select * from rewards | |
---@param query? string | |
---@return any | |
function SQL.readcommand(query) end | |
---Runs a SQLite write command which includes CREATE,INSERT, UPDATE. Ex: create TABLE rewards (ID integer PRIMARY KEY, action VARCHAR(20)) | |
---@param query? string | |
---@return string | |
function SQL.writecommand(query) end | |
---A library for manipulating the Tastudio dialog of the EmuHawk client | |
---@class tastudio | |
tastudio = {} | |
---@param name string | |
---@param text string | |
---@param width integer | |
function tastudio.addcolumn(name, text, width) end | |
function tastudio.applyinputchanges() end | |
---Clears the cache that is built up by using `tastudio.onqueryitemicon`, so that changes to the icons on disk can be picked up. | |
function tastudio.clearIconCache() end | |
function tastudio.clearinputchanges() end | |
---returns whether or not tastudio is currently engaged (active) | |
---@return boolean | |
function tastudio.engaged() end | |
---Returns a list of the current tastudio branches. Each entry will have the Id, Frame, and Text properties of the branch | |
---@return table | |
function tastudio.getbranches() end | |
---Gets the controller state of the given frame with the given branch identifier | |
---@param branchId string | |
---@param frame integer | |
---@return table | |
function tastudio.getbranchinput(branchId, frame) end | |
---returns the marker text at the given frame, or an empty string if there is no marker for the given frame | |
---@param frame integer | |
---@return string | |
function tastudio.getmarker(frame) end | |
---returns whether or not TAStudio is in recording mode | |
---@return boolean | |
function tastudio.getrecording() end | |
---gets the currently selected frames | |
---@return table | |
function tastudio.getselection() end | |
---Returns whether or not the given frame has a savestate associated with it | |
---@param frame integer | |
---@return boolean | |
function tastudio.hasstate(frame) end | |
---Returns whether or not the given frame was a lag frame, null if unknown | |
---@param frame integer | |
---@return boolean | |
function tastudio.islag(frame) end | |
---Loads a branch at the given index, if a branch at that index exists. | |
---@param index integer | |
function tastudio.loadbranch(index) end | |
---called whenever a branch is loaded. luaf must be a function that takes the integer branch index as a parameter | |
---@param luaf function | |
function tastudio.onbranchload(luaf) end | |
---called whenever a branch is removed. luaf must be a function that takes the integer branch index as a parameter | |
---@param luaf function | |
function tastudio.onbranchremove(luaf) end | |
---called whenever a branch is created or updated. luaf must be a function that takes the integer branch index as a parameter | |
---@param luaf function | |
function tastudio.onbranchsave(luaf) end | |
---Called whenever the greenzone is invalidated. Your callback can have 1 parameter, which will be the index of the first row that was invalidated. | |
---@param luaf function | |
function tastudio.ongreenzoneinvalidated(luaf) end | |
---called during the background draw event of the tastudio listview. luaf must be a function that takes 2 params: index, column. The first is the integer row index of the listview, and the 2nd is the string column name. luaf should return a value that can be parsed into a .NET Color object (string color name, or integer value) | |
---@param luaf function | |
function tastudio.onqueryitembg(luaf) end | |
---Called during the icon draw event of the tastudio listview. `luaf` must be a function that takes 2 params: `(index, column)`. The first is the integer row index of the listview, and the 2nd is the string column name. The callback should return a string, the path to the `.ico` file to be displayed. The file will be cached, so if you change the file on disk, call `tastudio.clearIconCache()`. | |
---@param luaf function | |
function tastudio.onqueryitemicon(luaf) end | |
---Called during the text draw event of the tastudio listview. `luaf` must be a function that takes 2 params: `(index, column)`. The first is the integer row index of the listview, and the 2nd is the string column name. The callback should return a string to be displayed. | |
---@param luaf function | |
function tastudio.onqueryitemtext(luaf) end | |
---if there is a marker for the given frame, it will be removed | |
---@param frame integer | |
function tastudio.removemarker(frame) end | |
---adds the given message to the existing branch, or to the branch that will be created next if branch index is not specified | |
---@param text string | |
---@param index? integer | |
function tastudio.setbranchtext(text, index) end | |
---Sets the lag information for the given frame, if the frame does not exist in the lag log, it will be added. If the value is null, the lag information for that frame will be removed | |
---@param frame integer | |
---@param value? boolean | |
function tastudio.setlag(frame, value) end | |
---Adds or sets a marker at the given frame, with an optional message | |
---@param frame integer | |
---@param message? string | number | |
function tastudio.setmarker(frame, message) end | |
---Seeks the given frame (a number) or marker (a string) | |
---@param frame any | |
function tastudio.setplayback(frame) end | |
---sets the recording mode on/off depending on the parameter | |
---@param val boolean | |
function tastudio.setrecording(val) end | |
---@param frame integer | |
---@param button string | |
---@param value number | |
function tastudio.submitanalogchange(frame, button, value) end | |
---@param frame integer | |
---@param number integer | |
function tastudio.submitclearframes(frame, number) end | |
---@param frame integer | |
---@param number integer | |
function tastudio.submitdeleteframes(frame, number) end | |
---@param frame integer | |
---@param button string | |
---@param value boolean | |
function tastudio.submitinputchange(frame, button, value) end | |
---@param frame integer | |
---@param number integer | |
function tastudio.submitinsertframes(frame, number) end | |
---toggles tastudio recording mode on/off depending on its current state | |
function tastudio.togglerecording() end | |
---A library for setting and retrieving dynamic data that will be saved and loaded with savestates | |
---@class userdata | |
userdata = {} | |
---clears all user data | |
function userdata.clear() end | |
---returns whether or not there is an entry for the given key | |
---@param key string | |
---@return boolean | |
function userdata.containskey(key) end | |
---gets the data with the given key, if the key does not exist it will return nil | |
---@param key string | |
---@return any | |
function userdata.get(key) end | |
---returns a list-like table of valid keys | |
---@return table | |
function userdata.get_keys() end | |
---remove the data with the given key. Returns true if the element is successfully found and removed; otherwise, false. | |
---@param key string | |
---@return boolean | |
function userdata.remove(key) end | |
---adds or updates the data with the given key with the given value | |
---@param name string | |
---@param value any | |
function userdata.set(name, value) end |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment