Created
February 1, 2021 15:33
-
-
Save karljj1/9e22dbae2aea5981baabb96a4c133700 to your computer and use it in GitHub Desktop.
Change the particle system update so that LateUpdate is taken into account
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System.Linq; | |
using UnityEngine; | |
using UnityEngine.LowLevel; | |
using UnityEngine.PlayerLoop; | |
using static UnityEngine.PlayerLoop.PreLateUpdate; | |
public class ChangePlayerLoop : MonoBehaviour | |
{ | |
public bool defaultLoop = true; | |
// Start is called before the first frame update | |
void Start() | |
{ | |
if (defaultLoop) | |
{ | |
PlayerLoop.SetPlayerLoop(PlayerLoop.GetDefaultPlayerLoop()); | |
return; | |
} | |
var loop = PlayerLoop.GetCurrentPlayerLoop(); | |
PlayerLoopSystem? particleUpdate = null; | |
// Find the particle system update | |
for (int i = 0; i < loop.subSystemList.Length; ++i) | |
{ | |
if (loop.subSystemList[i].type == typeof(PreLateUpdate)) | |
{ | |
var preLateUpdate = loop.subSystemList[i]; | |
for (int j = 0; j < preLateUpdate.subSystemList.Length; ++j) | |
{ | |
if (preLateUpdate.subSystemList[j].type == typeof(ParticleSystemBeginUpdateAll)) | |
{ | |
// Remove particle system update | |
particleUpdate = preLateUpdate.subSystemList[j]; | |
var list = preLateUpdate.subSystemList.ToList(); | |
list.RemoveAt(j); | |
preLateUpdate.subSystemList = list.ToArray(); | |
} | |
} | |
} | |
} | |
if (!particleUpdate.HasValue) | |
return; | |
// Move it so it is updated after LateUpdate | |
for (int i = 0; i < loop.subSystemList.Length; ++i) | |
{ | |
if (loop.subSystemList[i].type == typeof(PostLateUpdate)) | |
{ | |
var system = loop.subSystemList[i]; | |
var list = system.subSystemList.ToList(); | |
list.Add(particleUpdate.Value); | |
system.subSystemList = list.ToArray(); | |
} | |
} | |
PlayerLoop.SetPlayerLoop(loop); | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
This moves the update to come after LateUpdate
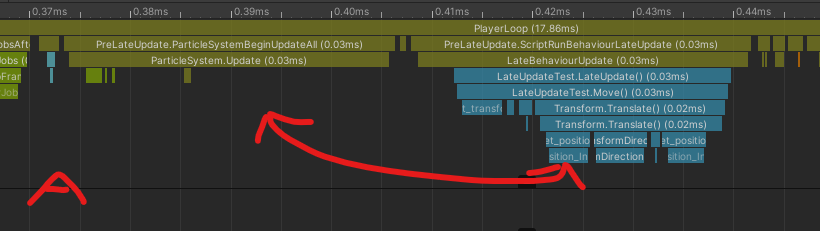
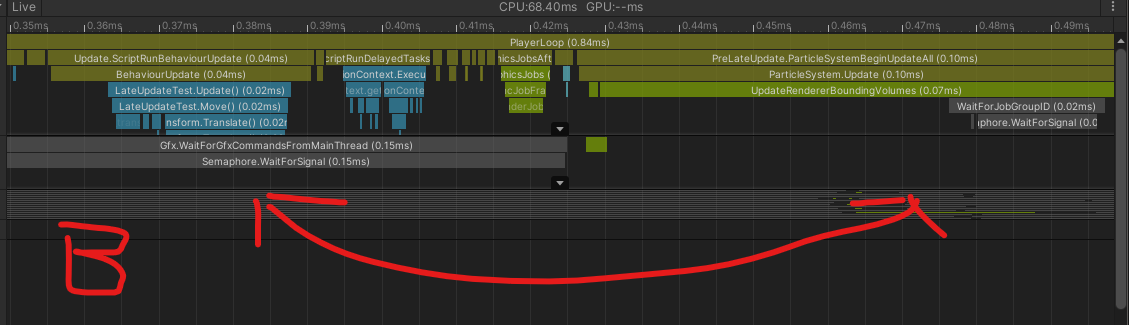
A is before with an example script and B is after changing the loop