Created
May 21, 2021 19:54
-
-
Save kellenff/5b9fab4690e60735650717f8ae34f7da to your computer and use it in GitHub Desktop.
React Layout component example
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import React from "react"; | |
import {Card, CardContent, CardHeader, Grid, Typography} from "@material-ui/core"; | |
type Props = { | |
title: string; | |
start?: React.ReactNode; | |
}; | |
const CustomCard: React.FC<Props> = ({title, start, children}) => { | |
return ( | |
<Card> | |
<CardHeader | |
title={ | |
start ? ( | |
<Grid container> | |
<Grid item>{start}</Grid> | |
<Grid item>{title}</Grid> | |
</Grid> | |
) : ( | |
title | |
) | |
} | |
/> | |
<CardContent>{children}</CardContent> | |
</Card> | |
); | |
}; | |
export default CustomCard; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import React from "react"; | |
import Layout from "../components/layout"; | |
import {Grid, Typography} from "@material-ui/core"; | |
import CustomCard from "../components/custom-card"; | |
import {Timer} from "@material-ui/icons"; | |
const Home: React.FC = () => { | |
return ( | |
<Layout header="Home"> | |
<Grid container spacing={2}> | |
<Grid item xs={12}> | |
<CustomCard title="Card 1"> | |
<Typography>Hello from card 1</Typography> | |
</CustomCard> | |
</Grid> | |
<Grid item xs={12}> | |
<CustomCard title="Card with icon" start={<Timer />}> | |
<Typography>I have an icon in the header</Typography> | |
</CustomCard> | |
</Grid> | |
</Grid> | |
</Layout> | |
); | |
}; | |
export default Home; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import React from "react"; | |
import Navigation from "./navigation"; | |
import {Container, Typography} from "@material-ui/core"; | |
type Props = { | |
header: string; | |
}; | |
const Layout: React.FC<Props> = ({header, children}) => { | |
return ( | |
<Container> | |
<Typography variant="h1">{header}</Typography> | |
{children} | |
<Navigation /> | |
</Container> | |
); | |
}; | |
export default Layout; |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Produces something like this:
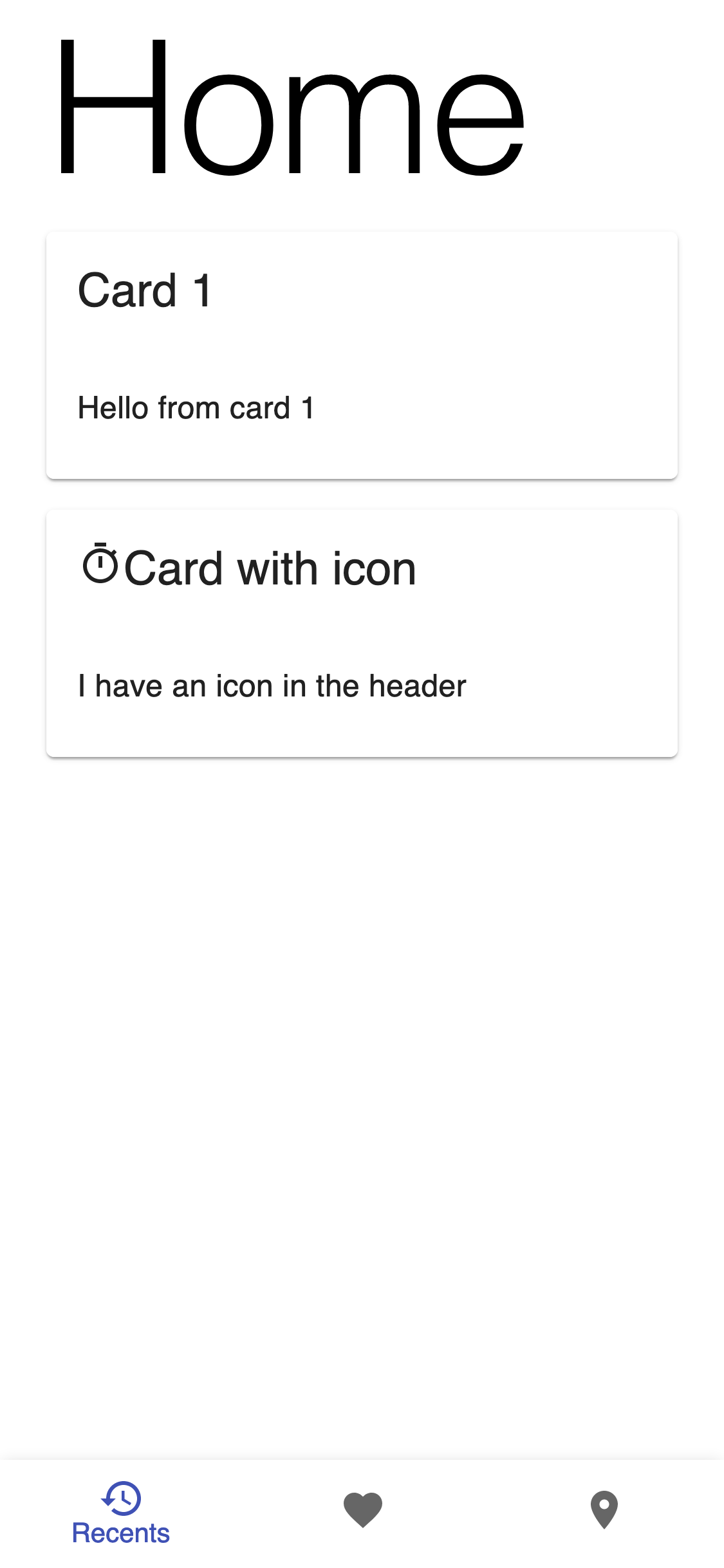