Last active
January 6, 2021 15:30
-
-
Save kieranmv95/8e64af1fb47583e80745d36f3f3c734c to your computer and use it in GitHub Desktop.
React hook for getting the users preffered themeName from OS and then adding a manual toggle option
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import { useEffect, useState } from 'react'; | |
// This hook checks if the prefers-color-scheme is supported in the users and if | |
// so grabs the theme from the OS settings. | |
// | |
// If the theme is not supported it defaults to return the string light | |
// | |
// If the user manually toggles the theme to light or dark that is preferred over | |
// the OS settings | |
// | |
// Usage: const [theme, toggleTheme] = useTheme(); | |
// theme: String [light || dark] | |
// toggleTheme: switches theme to alternative option | |
// | |
export default function useTheme() { | |
const [theme, setTheme] = useState(null); | |
const toggleTheme = () => { | |
if (theme === 'light') { | |
localStorage.setItem('theme', 'dark'); | |
setTheme('dark'); | |
} else { | |
localStorage.setItem('theme', 'light'); | |
setTheme('light'); | |
} | |
}; | |
const selectTheme = () => { | |
if (window.matchMedia('(prefers-color-scheme: light)').matches) { | |
setTheme('light'); | |
} else { | |
setTheme('dark'); | |
} | |
}; | |
useEffect(() => { | |
const preferredTheme = localStorage.getItem('theme'); | |
const pcs = window.matchMedia('(prefers-color-scheme)'); | |
if (preferredTheme) { | |
setTheme(preferredTheme); | |
} else if (pcs.media !== 'not all') { | |
selectTheme(); | |
window | |
.matchMedia('(prefers-color-scheme: dark)') | |
.addEventListener('change', selectTheme); | |
} else { | |
setTheme('light'); | |
} | |
}, []); | |
return [theme, toggleTheme]; | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Usage example. In my project i used the useTheme hook to get the theme name the user prefers and then pull that from my theme object and pass it into my styled components theme context to use throughout the application.
Layout.js
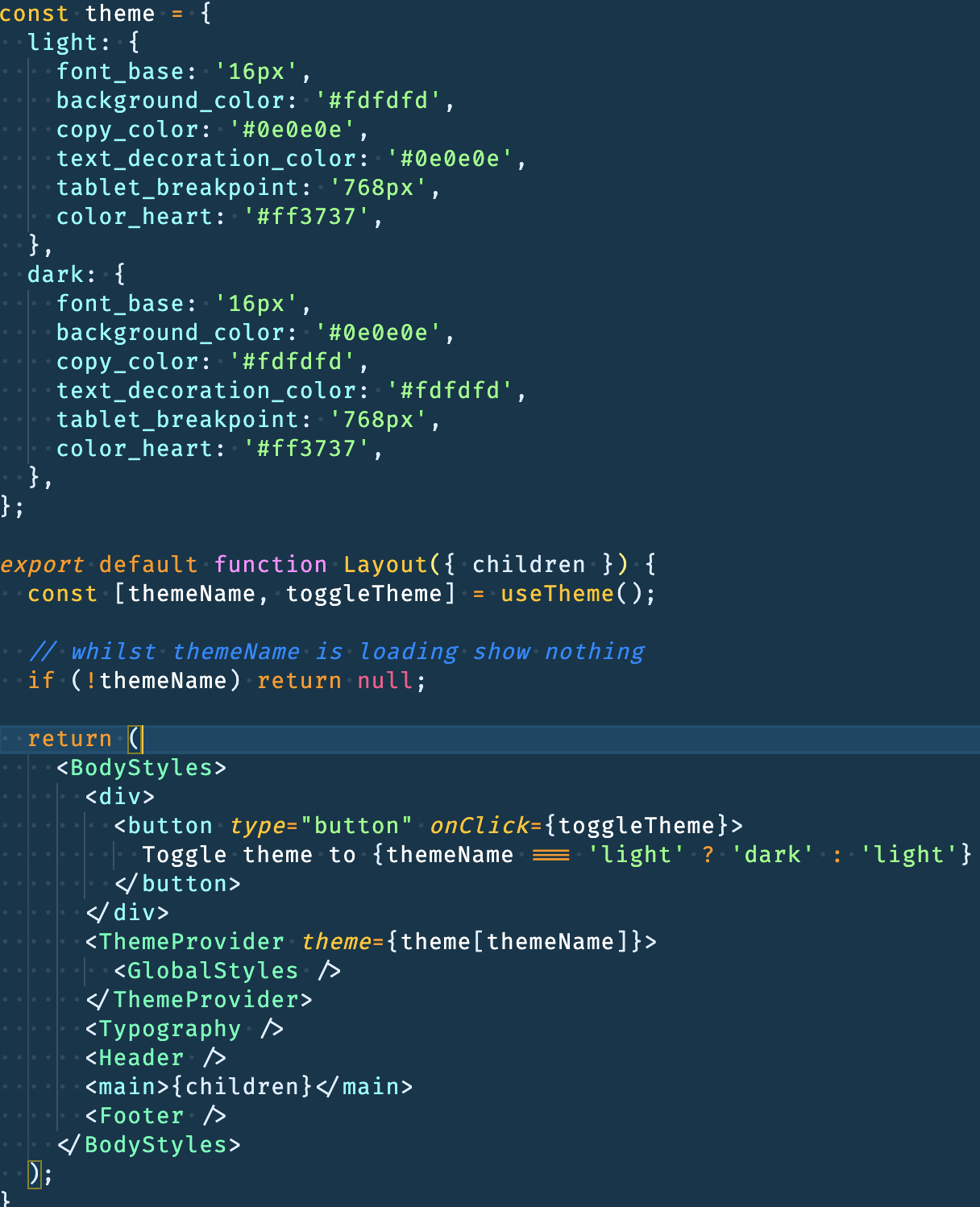
GlobalStyles.js
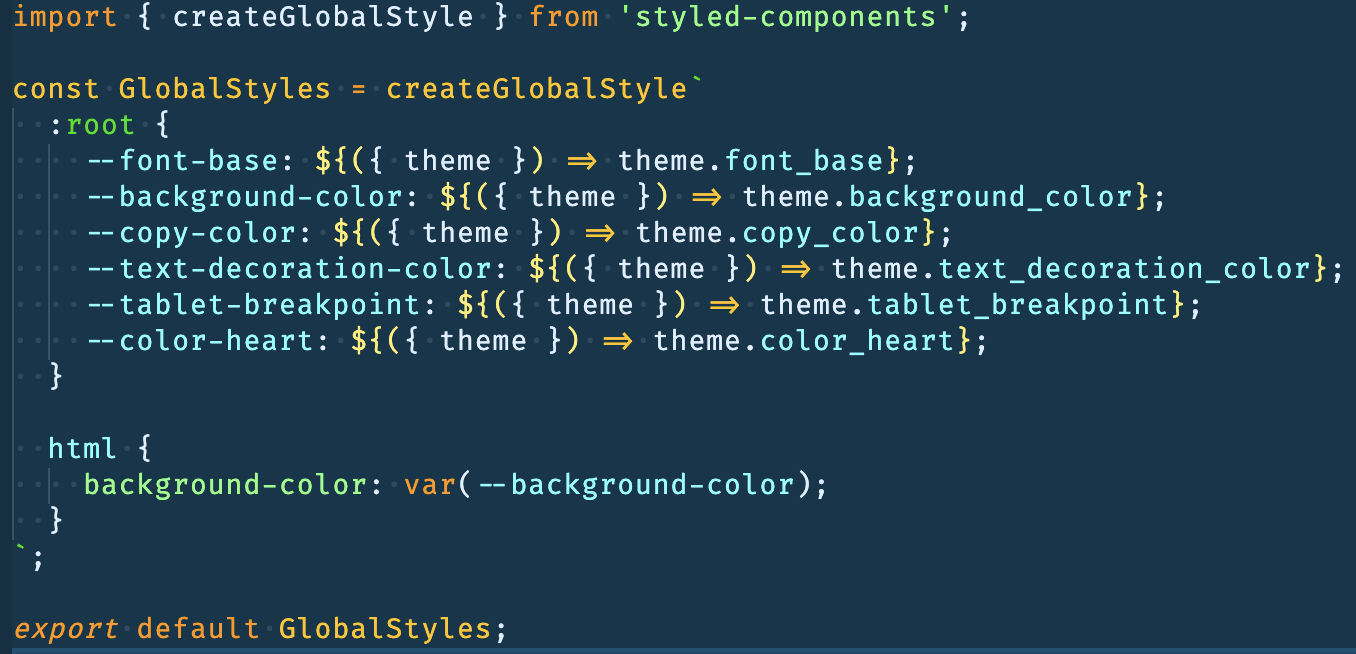