Last active
October 17, 2022 22:29
-
-
Save kieronlawson/53d763aa04c3b4e1785dc22dfbbb402f to your computer and use it in GitHub Desktop.
Example Spoke Insights Cards using Twilio Serverless Runtime
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const insights = [ | |
{ | |
"title":"Line Called", | |
"body":"\n**Mercedes-Benz London**\n35 Southdale Road East, London, ON N6c4X5\n\n**Office Hours**\nSales: 9am - 7pm\nService:7:30am - 5pm\nParts: 7:30am - 5pm\n\n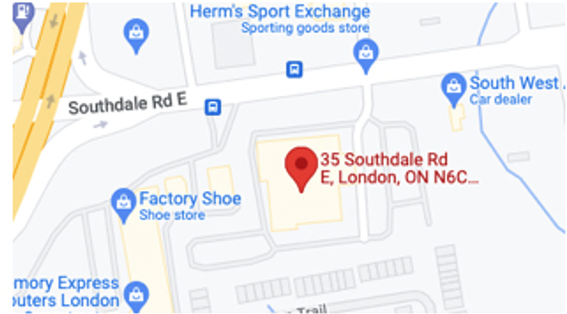", | |
"state":"expanded", | |
"thumbnail":{ | |
"icon_name":"phone" | |
} | |
}, | |
{ | |
"title":"Assigned Salesperson", | |
"body":"\nJames McKinney", | |
"state":"expanded", | |
"thumbnail":{ | |
"icon_name":"badge" | |
} | |
} | |
]; | |
function isValidUser(userEmail) { | |
// add list of valid users to this array | |
const validUsers = [ | |
"test@test.com", | |
"email@address.com" | |
]; | |
return validUsers.includes(userEmail.toLowerCase()); | |
} | |
async function getInsights(context, event, callback) { | |
try { | |
/** | |
* Event is an object that will contain query string parameters from | |
* Spoke Insights Data Action | |
* | |
* The syntax { varname = "" } = event will extract the value into varname, if the value is not | |
* provided then varname will default to empty string | |
*/ | |
const { userEmail = "" } = event; | |
// exit with empty payload if user is not in valid user list | |
if (!isValidUser(userEmail)) { | |
callback(null, []); | |
return; | |
} | |
// return insights payload | |
callback(null, insights); | |
} catch (error) { | |
console.error("Insights error", error); | |
callback(error.toString(), null); | |
} | |
} | |
exports.handler = getInsights; |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment