Created
August 13, 2018 08:10
-
-
Save ktajpuri/461359e35f730f8372e7e62dcad25c63 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
class Person { | |
constructor(name, age) { | |
this.name = name; | |
this.age = age; | |
} | |
printIdentity() { | |
return "Mr. " + this.name; | |
} | |
testFunc() { | |
console.log("I am test from person"); | |
} | |
} | |
class Student extends Person { | |
constructor(std, name, age) { | |
super(name, age); | |
this.std = std; | |
} | |
printGreeting() { | |
return super.printIdentity() + " is in standard " + this.std; | |
} | |
testFunc() { | |
console.log("I am test from student"); | |
} | |
} | |
class HeadBoy extends Student { | |
constructor(house, std, name, age) { | |
super(std, name, age); | |
this.house = house; | |
} | |
print() { | |
return ( | |
super.printGreeting() + | |
" and is in house " + | |
this.house + | |
" he is " + | |
this.age + | |
" years old" | |
); | |
} | |
/*testFunc() {//uncommenting this function will invoke test func from headboy | |
console.log('I am test from head boy') | |
}*/ | |
} | |
let headboy = new HeadBoy("gandhi", "10th", "kamlesh", 20); | |
headboy.testFunc(); |
Interesting this to note here is that ES6 class keyword is just syntactical sugar over the JavaScript prototypical inheritance.
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Prototype chain for this -
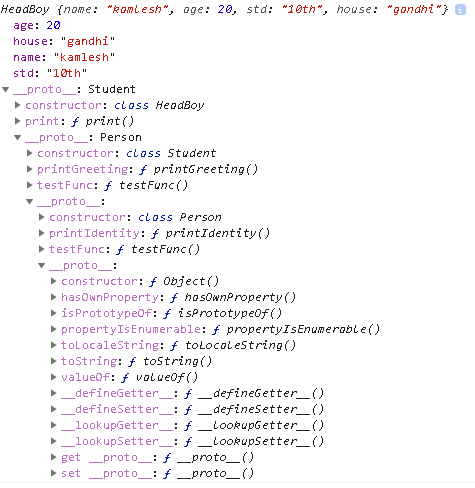