- Cannot change
- Examples:
1
,9.12
,True
, or"Foo"
- Have values which can change
- Assigned a value using the equal sign
- Examples:
x = 43
,y = "flarp"; x = y
- A combination of operators (i.e.
+
or*
), variables, constants (and more) that the programming language interprets and computes to return a value - Example:
x + 1
orraw_input("Gimme yo' name!")
- Can be nested, and evaluated from the inside-out:
x = 100
y = 200
z = (x + (y + 50))
input_as_int = int(raw_input("Enter a number, jerk: "))
-
In many (most?) languages, a statement is some code that expresses some action to be carried out but that does not produce a result
-
Example ("assignment statement"):
x = 1
-
Example ("if statement"):
if x < 0: message = 'Negative' else: message = 'Not negative'
-
How can you tell if something is a statement or an expression? Wrap it in parens and try to assign it to something:
>>> x = 2 >>> y = (x = 4) File "<stdin>", line 1 y = (x = 4) ^ SyntaxError: invalid syntax
Lots of types:
- string
"hello"
- floating-point number
1.12
- integer
1212
Why do they matter? The computer does a bad job at combining disparate types.
For example, +
with a string and integer:
>>> "foo" + 123
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: cannot concatenate 'str' and 'int' objects
...but what about multiplication?
>>> "foo" * 3
'foofoofoo'
Lots of useful functions for converting between types - but watch out: some information can be lost!
>>> int(1.1)
1
>>> float("1.1")
1.1
>>> str(1)
'1'
>>> bool("hotdog")
True
>>> bool("")
False
2.2 Write a program that uses raw_input to prompt a user for their name and then welcomes them. Note that raw_input will pop up a dialog box. Enter Sarah in the pop-up box when you are prompted so your output will match the desired output.
name = raw_input("Enter your name")
print "Howdy"
Write a program to prompt the user for hours and rate per hour using raw_input to compute gross pay. Use 35 hours and a rate of 2.75 per hour to test the program (the pay should be 96.25). You should use raw_input to read a string and float() to convert the string to a number. Do not worry about error checking or bad user data.
# This first line is provided for you
hrs = raw_input("Enter Hours:")
The simplest conditional statement!
if n < 0:
print "Negative!"
else:
print "Not negative!"
"Conditions" are evaluated top-to-bottom until one evaluates to True
. Then the code in the "statement block" will be evaluated.
Conditional statements like if/else can be nested, too:
if n < 0:
print "Negative!"
else:
if n == 0:
print "Zero"
else:
print "Positive"
This nested if/else can be flattened out by using elsif
, a contraction of "else if":
if n < 0:
print "Negative!"
elsif n == 0:
print "Zero"
else:
print "Positive"
A "condition," in this context, is any expression that evaluates to a Boolean
value (True
or False
). Some examples include:
x < 10
raw_input("Are you over 18 years of age?") == "yes"
Conditions can be combined using "logical operators," which we haven't gotten to yet (I think?).
Some examples of these are and
and or
and not
.
Question: What is the value of the expression:
(1 == 1)
Question 2: What is the value of the expression:
((1 == 1) and (2 == 2))
Question 3:
(1 == 1) or (1 == 2)
Question 4:
not (1 == 1)
A common error you will come across is a SyntaxError
, which means you wrote some malformed code:
>>> x = elsif "woot"
File "<stdin>", line 1
x = elsif "woot"
^
SyntaxError: invalid syntax
This error occurs when Python attempts to parse (read) your program - but before it really starts executing it.
Even if a statement or expression is syntactically correct, it may cause an error when an attempt is made to execute it.
>>> 'foo' + 2
Traceback (most recent call last):
File "<stdin>", line 1, in ?
TypeError: cannot concatenate 'str' and 'int' objects
try
and except
allow you to trap and handle errors that occur when running your program:
try:
user_input = raw_input("Please enter a number: ")
x = int(user_input)
print ("Your number was: " + str(x))
except:
print "Oops! That was no valid number. Try again..."
When an error occurs, the next line in the block will not be executed as normal. Instead, Python will look outwards from the place where the error occurred to see if you have added a try
. In the case above, the expression print ("Your number was: " + str(x))
will not be executed if the user of the program enters input that Python can't convert to an integer - like "derp".
Write a program to prompt the user for hours and rate per hour using raw_input to compute gross pay. Pay the hourly rate for the hours up to 40 and 1.5 times the hourly rate for all hours worked above 40 hours. Use 45 hours and a rate of 10.50 per hour to test the program (the pay should be 498.75). You should use raw_input to read a string and float() to convert the string to a number. Do not worry about error checking the user input - assume the user types numbers properly.
hrs = raw_input("Enter Hours:")
h = float(hrs)
Write a program to prompt for a score between 0.0 and 1.0. If the score is out of range, print an error. If the score is between 0.0 and 1.0, print a grade using the following table:
Score Grade
>= 0.9 A
>= 0.8 B
>= 0.7 C
>= 0.6 D
< 0.6 F
If the user enters a value out of range, print a suitable error message and exit. For the test, enter a score of 0.85.
try:
score = float(raw_input("Enter your score: "))
grade = "A"
if (score < 0.6):
grade = "F"
elif (score < 0.7):
grade = "D"
elif (score < 0.8):
grade = "C"
elif (score < 0.9):
grade = "B"
print grade
except:
print "Input out of range."
@
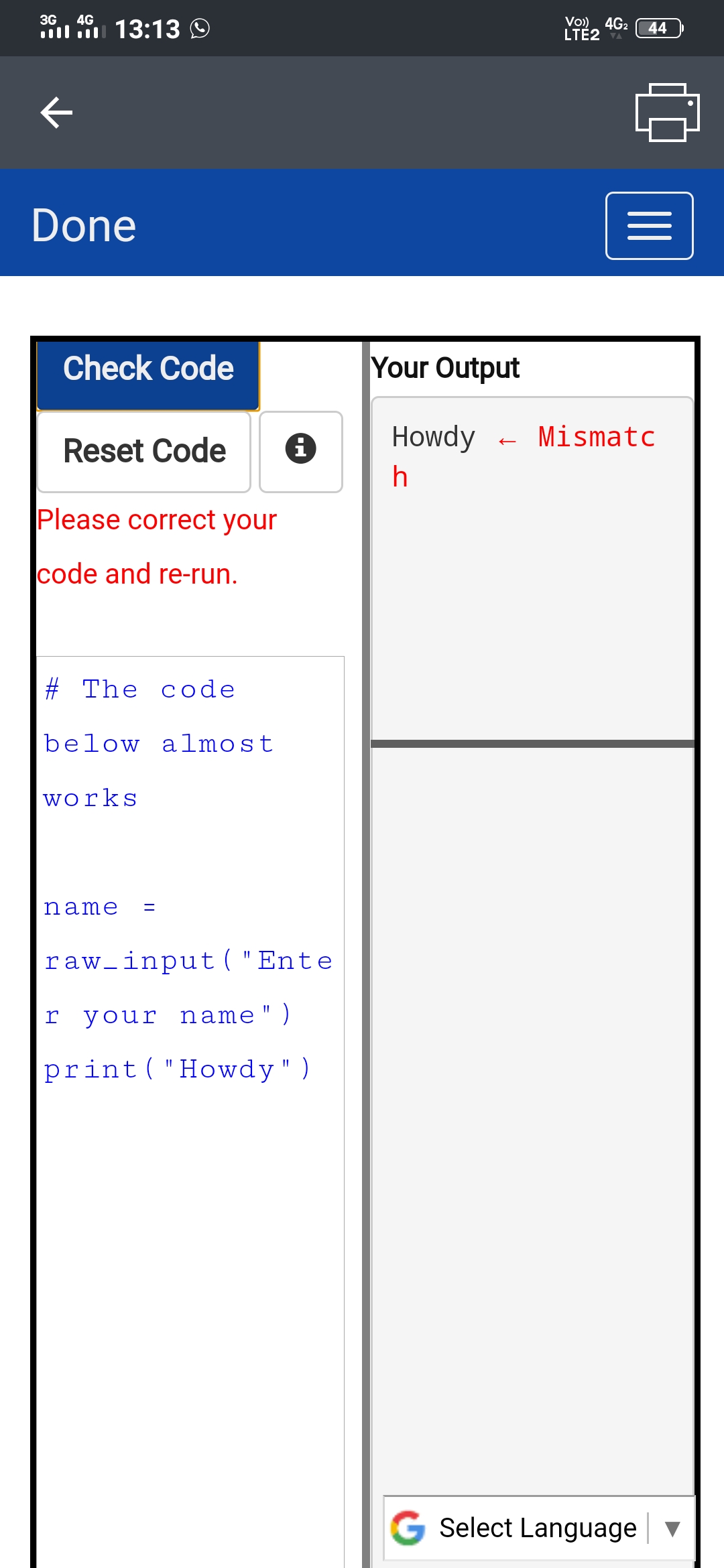