This is a companion gist to the article Sampling random variables and plotting histograms in Crystal
Your system has
Clone the files in this gist, then run the following once, to install the code dependencies.
shards install
You can now plot some histograms with
crystal normal_distribution.cr
and
crystal normal_vs_exp.cr
Output of
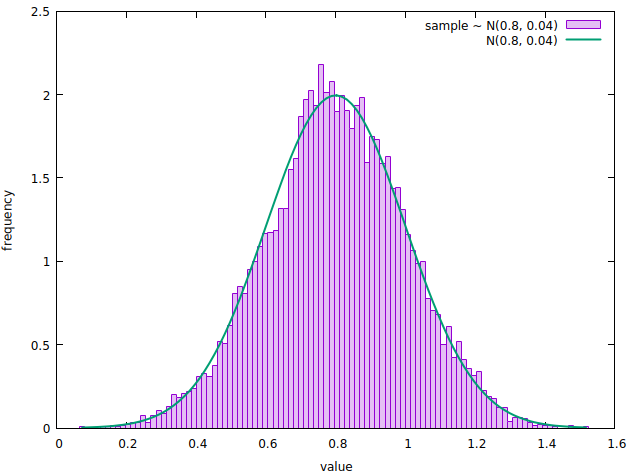
normal_distribution.cr