Created
April 15, 2023 07:10
-
-
Save listingclown3/7d5ac6e42a0cdc5ce85f28ededa3106b to your computer and use it in GitHub Desktop.
How to log into Discord using your token through Puppeteer
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const puppeteer = require('puppeteer'); | |
(async () => { | |
// Launch puppeteer instance | |
console.log("Launching Puppeteer browser...") | |
const browser = await puppeteer.launch(); | |
const page = await browser.newPage(); | |
const discordToken = '' // Your Discord Token, How To Get Your Discord Token -> https://www.youtube.com/watch?v=YEgFvgg7ZPI&ab_channel=GaugingGadgets | |
// Local Storage error with Puppeteer, found bypass, CREDIT: https://gist.github.com/zelbov/58e9fbbe5157bf61067d2693118dd09a | |
const bypassLocalStorageOverride = (page) => page.evaluateOnNewDocument(() => { | |
// Preserve localStorage as separate var to keep it before any overrides | |
let __ls = localStorage | |
// Restrict closure overrides to break global context reference to localStorage | |
Object.defineProperty(window, 'localStorage', { writable: false, configurable: false, value: __ls }) | |
}) | |
console.log("Redirecting to https://discord.com/app ... (May take a few seconds)") | |
// Calling function before storing token into Discord so that errors don't occur | |
bypassLocalStorageOverride(page) | |
await page.goto('https://discord.com/app'); | |
// Setting token into Discord Local Storage (Don't worry it's not being sent/stored anywhere, this is how Discord does it) | |
await page.evaluate((token) => { | |
localStorage.setItem('token', `"${token}"`); | |
}, discordToken); | |
// Navigate to a page where you want to use the local storage value | |
await page.goto(''); // The discord channel / App Authorization that you want to log into Discord for... | |
console.log("Successfully logged in...") | |
await page.waitForTimeout(5000) // Wait for page to load | |
// Do whatever you want here afterwards, I just decided to take a screenshot for proof | |
await page.screenshot({ path: 'output.png' }) | |
// Ending proccess | |
await browser.close() | |
})(); |
Will look into
I fixed a sepperate issue, made my own gist, will continue to update for my own need, I credited you:
https://gist.github.com/DevGod100/6b1c93379071804b54995e190b99623c
Not logged in to discord though, so the issue persists.
What do you think is the cause??
Will look into
also guys use an env variable plz...
### const discordToken = process.env.DISCORD_TOKEN;
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
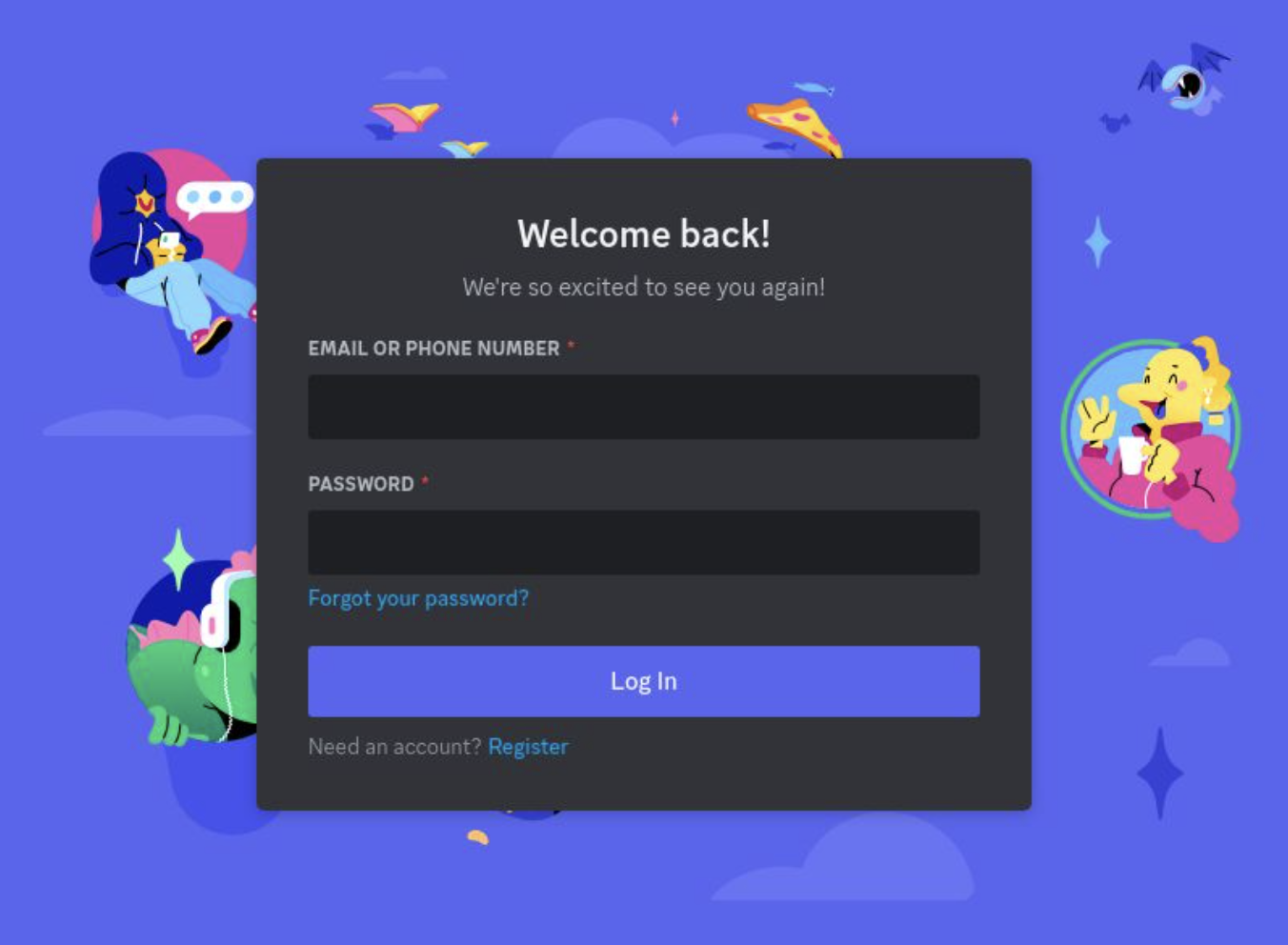
don't workObject.defineProperty(window, 'localStorage', { writable: false, configurable: false, value: __ls })