-
-
Save lloydzhou/e2fd09a1aab49d163562727f706d4160 to your computer and use it in GitHub Desktop.
钉钉(dingtalk)机器人客户端
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import json | |
import httpx | |
from typing import Dict, Any | |
class Base(Dict): | |
def __getattr__(self, name: str) -> Any: | |
try: | |
return self[name] | |
except KeyError: | |
raise AttributeError(name) | |
def __setattr__(self, name: str, value: Any) -> None: | |
self[name] = value | |
class DingDingAT(Base): | |
def __init__(self, *atMobile, atMobiles=list(), atUserIds=list(), isAtAll=False): | |
super().__init__(at=dict( | |
atMobiles=atMobiles + list(atMobile), | |
atUserIds=atUserIds, | |
isAtAll=isAtAll, | |
)) | |
class DingDingLinkMessage(Base): | |
def __init__(self, messageUrl='', picUrl='', text='', title='', **kwargs): | |
super().__init__( | |
msgtype='link', | |
link=dict(messageUrl=messageUrl, picUrl=picUrl, text=text, title=title) | |
) | |
class DingDingTextMessage(Base): | |
def __init__(self, content='', **kwargs): | |
super().__init__( | |
msgtype='text', | |
text=dict(content=content) | |
) | |
class DingDingFeedCardLink(Base): | |
def __init__(self, title='', messageURL='', picURL=''): | |
super().__init__(title=title, messageURL=messageURL, picURL=picURL) | |
class DingDingFeedCardMessage(Base): | |
def __init__(self, *link, links=list(), **kwargs): | |
super().__init__( | |
msgtype="feedCard", | |
feedCard=dict(links=links + list(link)), | |
**kwargs, | |
) | |
class DingDingMarkdownMessage(Base): | |
def __init__(self, text='', title='', **kwargs): | |
super().__init__( | |
msgtype='markdown', | |
markdown=dict(title=title, text=text), | |
**kwargs | |
) | |
class DingDingActionCardButton(Base): | |
def __init__(self, actionURL='', title=''): | |
super().__init__(actionURL=actionURL, title=title) | |
class DingDingActionCardMessage(Base): | |
def __init__(self, *btn, btns=list(), title='', text='', btnOrientation="0", **kwargs): | |
super().__init__( | |
msgtype='actionCard', | |
actionCard=dict( | |
title=title, | |
text=text, | |
btnOrientation=btnOrientation, | |
btns=btns + list(btn), | |
) | |
) | |
class DingDingBot(Base): | |
def __init__(self, webhook='', **kwargs): | |
super().__init__(webhook=webhook, **kwargs) | |
async def asend(self, msg): | |
return httpx.AsyncClient().post(self.webhook, data=json.dumps(msg), headers={'Content-Type': 'application/json'}).json() | |
def send(self, msg): | |
return httpx.post(self.webhook, data=json.dumps(msg), headers={'Content-Type': 'application/json'}).json() | |
if __name__ == "__main__": | |
client = DingDingBot('https://oapi.dingtalk.com/robot/sendBySession?session=xxxx') | |
# markdown中直接添加dtmd链接。可正常点击。但是不好布局 | |
response = client.send(DingDingMarkdownMessage(""" | |
# Midjourney Bot🎉 | |
 | |
[U1](dtmd://dingtalkclient/sendMessage?content=U1) | |
[U2](dtmd://dingtalkclient/sendMessage?content=U2) | |
[U3](dtmd://dingtalkclient/sendMessage?content=U3) | |
[U4](dtmd://dingtalkclient/sendMessage?content=U4) | |
[V1](dtmd://dingtalkclient/sendMessage?content=V1) | |
[V2](dtmd://dingtalkclient/sendMessage?content=V2) | |
[V3](dtmd://dingtalkclient/sendMessage?content=V3) | |
[V4](dtmd://dingtalkclient/sendMessage?content=V4) | |
""", title='测试dtmd链接')) | |
print('response', response) | |
# ActionCard消息底部按钮使用dtmd链接,消息的text也支持markdown格式 | |
message = DingDingActionCardMessage( | |
DingDingActionCardButton('dtmd://dingtalkclient/sendMessage?content=内容不错', '内容不错'), | |
DingDingActionCardButton('dtmd://dingtalkclient/sendMessage?content=不感兴趣', '不感兴趣'), | |
title='我 20 年前想打造一间苹果咖啡厅,而它正是 Apple Store 的前身', | |
text="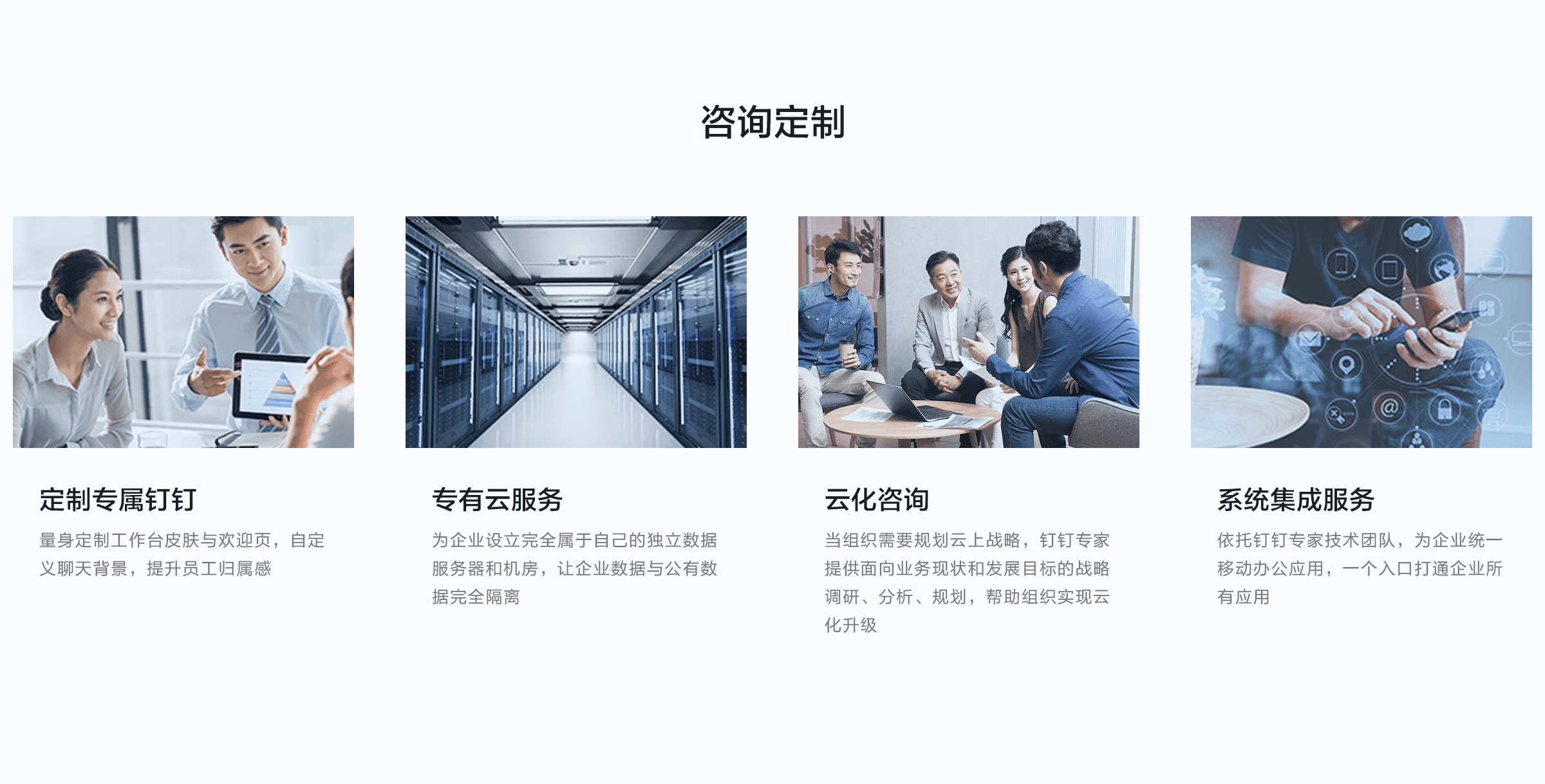 \n\n #### 乔布斯 20 年前想打造的苹果咖啡厅 \n\n Apple Store 的设计正从原来满满的科技感走向生活化,而其生活化的走向其实可以追溯到 20 年前苹果一个建立咖啡馆的计划" | |
) | |
print('message', message) | |
response = client.send(message) | |
print('response', response) | |
Author
lloydzhou
commented
Jul 13, 2023
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment