Okay! Here are the environment keys and flows that authentication middleware follow.
Any API you see on IAuthenticationManager, IOwinContext, IOwinRequest, IOwinResponse are only syntax sugar and utility code around these env keys and values.
Simplest cases first: the application wants to sign in or sign out the user.
For SignIn it means that it has calculated the claims the user should possess, and it passes those claims to the appropriate authentication middleware by creating a ClaimsIdentity where the identity.AuthenticationType exactly equals the middleware's options.AuthenticationType.
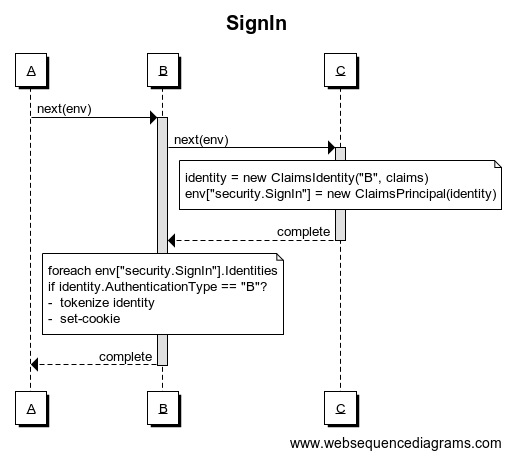For SignOut there are no claims involved, so the value for the key is just the array of AuthenticationType strings matching the middleware's option.AuthenticationType.
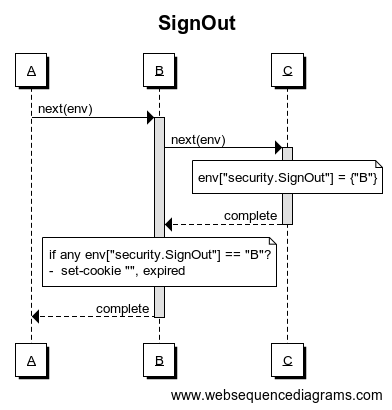Multiple middleware can SignIn by having several ClaimsIdentity onto the ClaimsPrincipal.Identities. Multiple middleware can SignOut by having more than one AuthenticationType in the string array.
These two flows are the most similar to the traditional forms http module. This is assuming the options.AuthenticationMode is active.
If a request arrives for a protected resource, and the user is anonymous or has not presented sufficient credentials, a challenge is produced by the convention of a 401 response status code. In the case of cookie auth middleware this is converted to a redirect that is expected to SignIn and return. Other mechanisms, like social logins, are more complex but boil down to redirecting away and eventually returning.
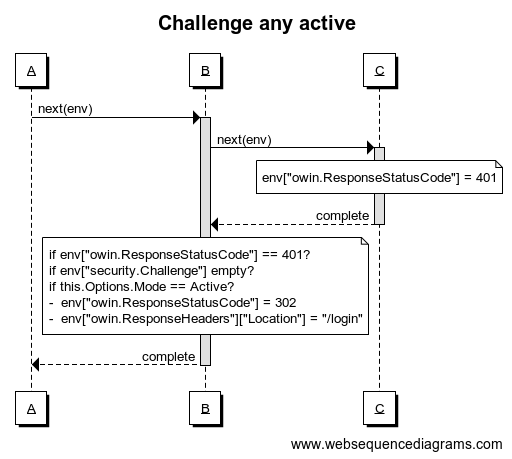After the user has successfully signed in the IPrincipal/ClaimsPrincipal may be examined.
The user principal generated by active middleware will be present on the OWIN environment dictionary and will also be assigned to the ASPNET request.User value.
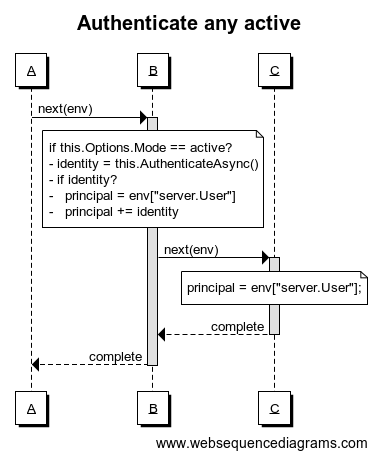For the cases where you want a 401 to address a specific middleware by it's AuthenticationType name, you can direct the challenge with an additional env key. This makes the active/passive mode irrelevant, really, because the middleware will use only the AuthenticationType equality to determine if any action should be performed.
Common examples of this are social authentication types, which typically ignore outgoing 401's unless they are explicitly named. Another common example of this is when you have an active middleware, like cookie which will redirect, you may set the challenge authentication type to any other value to prevent the redirect from occuring.
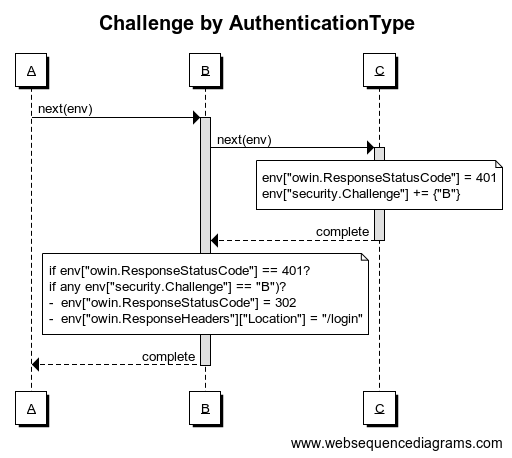The case where you need to authenticate by authentication type is one where you invoke an api function out of the enviroment, instead of looking at the request user. The active/passive mode is irrelevant for this calling pattern as well, because only the authenticationTypes parameter determines which middleware should perform their authentication and callback with an identity.
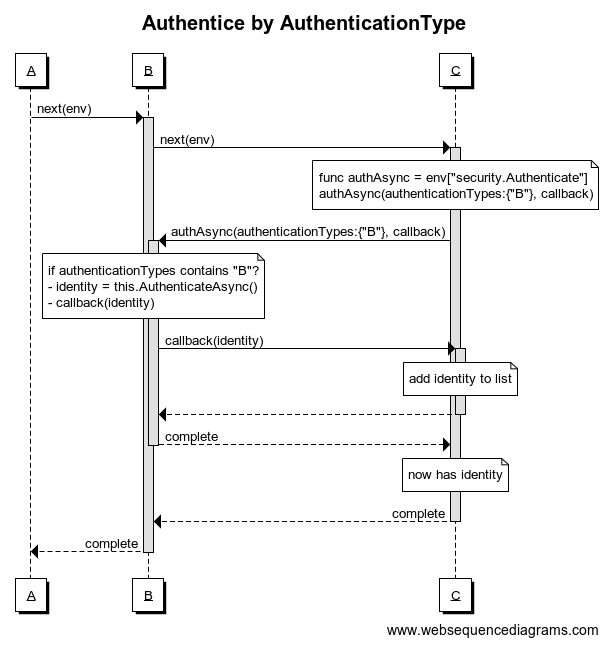