Last active
July 12, 2022 03:35
-
-
Save lucaswkuipers/4f938e6233d17049c6aa06f6671230a8 to your computer and use it in GitHub Desktop.
Simple app view with Einstein's photo and a made up quote.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import UIKit | |
final class QuoteView: UIView { | |
private let portraitImageView: UIImageView = { | |
let imageView = UIImageView() | |
imageView.layer.cornerRadius = 64 | |
imageView.clipsToBounds = true | |
return imageView | |
}() | |
private let quoteLabel: UILabel = { | |
let label = UILabel() | |
label.font = .preferredFont(forTextStyle: .body) | |
label.numberOfLines = 0 | |
label.textAlignment = .center | |
return label | |
}() | |
private let authorLabel: UILabel = { | |
let label = UILabel() | |
label.font = .preferredFont(forTextStyle: .caption1) | |
label.numberOfLines = 1 | |
return label | |
}() | |
init() { | |
super.init(frame: .zero) | |
setupViewStyle() | |
setupViewHierarchy() | |
setupViewConstraints() | |
} | |
required init?(coder: NSCoder) { | |
super.init(coder: coder) | |
} | |
func setupData(imageName: String, quote: String, author: String) { | |
portraitImageView.image = UIImage(named: imageName) | |
quoteLabel.text = quote | |
authorLabel.text = "- \(author)." | |
} | |
private func setupViewStyle() { | |
backgroundColor = .systemBackground | |
} | |
private func setupViewHierarchy() { | |
addSubview(portraitImageView) | |
addSubview(quoteLabel) | |
addSubview(authorLabel) | |
} | |
private func setupViewConstraints() { | |
subviews.forEach { $0.translatesAutoresizingMaskIntoConstraints = false } | |
NSLayoutConstraint.activate([ | |
portraitImageView.centerYAnchor.constraint(equalTo: centerYAnchor, constant: -40), | |
portraitImageView.centerXAnchor.constraint(equalTo: centerXAnchor), | |
portraitImageView.widthAnchor.constraint(equalToConstant: 128), | |
portraitImageView.heightAnchor.constraint(equalToConstant: 128), | |
quoteLabel.topAnchor.constraint(equalTo: portraitImageView.bottomAnchor, constant: 40), | |
quoteLabel.leadingAnchor.constraint(equalTo: leadingAnchor, constant: 20), | |
quoteLabel.trailingAnchor.constraint(equalTo: trailingAnchor, constant: -20), | |
authorLabel.topAnchor.constraint(equalTo: quoteLabel.bottomAnchor, constant: 20), | |
authorLabel.leadingAnchor.constraint(equalTo: quoteLabel.leadingAnchor), | |
authorLabel.trailingAnchor.constraint(equalTo: quoteLabel.trailingAnchor) | |
]) | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Used image:
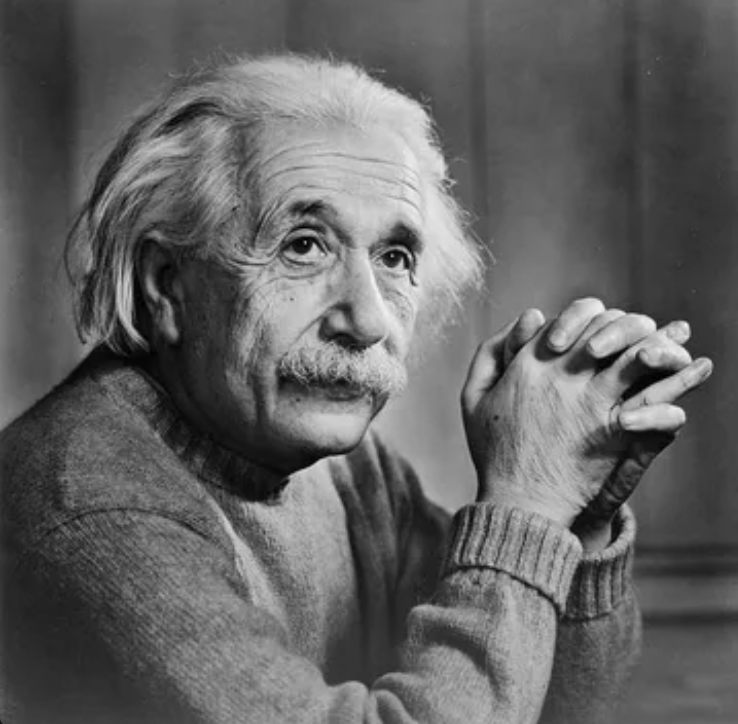