Last active
January 28, 2020 16:12
-
-
Save luigi-rosso/c50277341bd2681be072a575acbeb1fc to your computer and use it in GitHub Desktop.
Swap a Flare image at runtime.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import 'dart:async'; | |
import 'dart:typed_data'; | |
import 'package:flare_dart/math/mat2d.dart'; | |
import 'package:flare_flutter/flare.dart'; | |
import "package:flare_flutter/flare_actor.dart"; | |
import 'package:flare_flutter/flare_controller.dart'; | |
import "package:flutter/material.dart"; | |
import 'package:flutter/services.dart'; | |
void main() => runApp(MyApp()); | |
class MyApp extends StatelessWidget { | |
// This widget is the root of your application. | |
@override | |
Widget build(BuildContext context) { | |
return MaterialApp( | |
title: 'Flare Demo', | |
theme: ThemeData(primarySwatch: Colors.blue), | |
home: MyHomePage(title: 'Flare-Flutter'), | |
); | |
} | |
} | |
class MyHomePage extends StatefulWidget { | |
MyHomePage({Key key, this.title}) : super(key: key); | |
final String title; | |
@override | |
_MyHomePageState createState() => _MyHomePageState(); | |
} | |
class _MyHomePageState extends State<MyHomePage> { | |
String _animationName = "idle"; | |
ImageSwapController _imageSwapper = ImageSwapController(); | |
@override | |
Widget build(BuildContext context) { | |
return Scaffold( | |
backgroundColor: Colors.grey, | |
appBar: AppBar(title: Text(widget.title)), | |
body: Center( | |
child: Column( | |
mainAxisAlignment: MainAxisAlignment.center, | |
mainAxisSize: MainAxisSize.min, | |
children: [ | |
Expanded( | |
child: FlareActor( | |
"assets/ImageSwap.flr", | |
controller: _imageSwapper, | |
alignment: Alignment.center, | |
fit: BoxFit.contain, | |
animation: _animationName, | |
), | |
) | |
], | |
), | |
), | |
); | |
} | |
} | |
class ImageSwapController extends FlareController { | |
@override | |
bool advance(FlutterActorArtboard artboard, double elapsed) { | |
return false; | |
} | |
@override | |
void initialize(FlutterActorArtboard artboard) { | |
var node = artboard.getNode("avatar2"); | |
if (node is FlutterActorImage) { | |
// Change it with a bundled image. | |
node.changeImageFromBundle(rootBundle, "assets/c.png").then((bool ok) { | |
// Because changeImageFromBundle is async, the FlareActor will have | |
// already drawn with the existing image. Since this example has no | |
// animation, it will have stopped re-drawing. So we need to let | |
// the FlareActor know that we want to re-draw with the new image. | |
isActive.value = true; | |
}); | |
// ...or change it with a network image | |
node | |
.changeImageFromNetwork("https://picsum.photos/250?image=9") | |
.then((bool ok) { | |
isActive.value = true; | |
}); | |
} | |
} | |
@override | |
void setViewTransform(Mat2D viewTransform) {} | |
} | |
/// Add an extension method to FlutterActorImage to change the image | |
/// with one downloaded from the network. | |
/// This is implemented as an extension method as the dev/master | |
/// signature for PaintingBinding.instance.instantiateImageCodec is | |
/// different from the one in stable. | |
extension NetworkFlutterActorImage on FlutterActorImage { | |
Future<bool> changeImageFromNetwork(String url) async { | |
var networkImage = NetworkImage(url); | |
var val = await networkImage.obtainKey(const ImageConfiguration()); | |
var load = networkImage.load(val, (Uint8List bytes, | |
{int cacheWidth, int cacheHeight}) { | |
return PaintingBinding.instance.instantiateImageCodec(bytes, | |
cacheWidth: cacheWidth, cacheHeight: cacheHeight); | |
}); | |
final completer = Completer<bool>(); | |
load.addListener(ImageStreamListener((ImageInfo info, bool syncCall) { | |
changeImage(info.image); | |
completer.complete(true); | |
})); | |
return completer.future; | |
} | |
} |
Can you share ImageSwap.flr
?
There seems to be an issue with toggling "Is Dynamic" and I've filed a bug here: 2d-inc/support#255
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
You can see the example file here: https://www.2dimensions.com/a/castor/files/flare/image-swap
Note how avatar2 has Is Dynamic marked true.
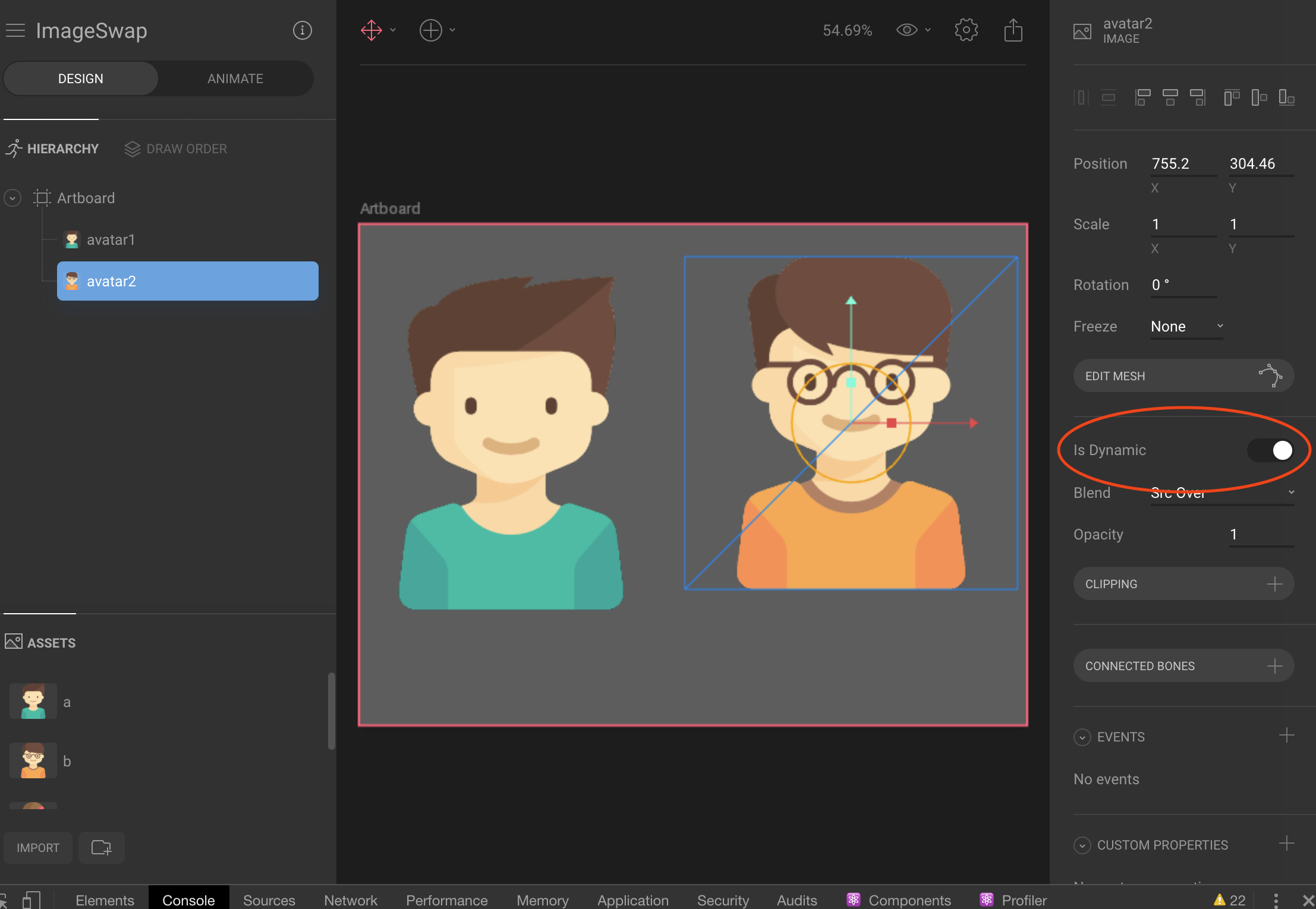