Last active
June 20, 2023 02:41
-
-
Save magiskboy/1b7a49b3f195c819829eb303e7ee1479 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import threading | |
import multiprocessing | |
import time | |
import requests | |
def measure(func): | |
def f(*args, **kwargs): | |
start = time.time() | |
ret = func(*args, **kwargs) | |
print(f"{func.__name__} takes {time.time()-start} seconds") | |
return ret | |
return f | |
def fib(n): | |
if n < 2: | |
return n | |
return fib(n - 1) + fib(n - 2) | |
@measure | |
def cpu_bounded_in_multiple_process(): | |
p1 = multiprocessing.Process(target=fib, args=(40,), daemon=True) | |
p2 = multiprocessing.Process(target=fib, args=(40,), daemon=True) | |
p1.start() | |
p2.start() | |
p1.join() | |
p2.join() | |
@measure | |
def cpu_bounded_in_multiple_thread(): | |
t1 = threading.Thread(target=fib, args=(40,), daemon=True) | |
t2 = threading.Thread(target=fib, args=(40,), daemon=True) | |
t1.start() | |
t2.start() | |
t1.join() | |
t2.join() | |
@measure | |
def cpu_bounded_in_single_thread(): | |
fib(40) | |
fib(40) | |
@measure | |
def io_bounded_in_single_thread(n_iters=10): | |
for i in range(n_iters): | |
requests.get("https://api.github.com/users/magiskboy") | |
@measure | |
def io_bounded_in_multiple_threads(n_iters=10): | |
ts = [] | |
for i in range(n_iters): | |
t = threading.Thread( | |
target=requests.get, | |
kwargs={"url": "https://api.github.com/users/magiskboy"}, | |
daemon=True, | |
) | |
t.start() | |
ts.append(t) | |
for t in ts: | |
t.join() | |
if __name__ == "__main__": | |
print("Read more https://github.com/python/cpython/blob/main/Python/ceval_gil.c#L19") | |
cpu_bounded_in_single_thread() | |
cpu_bounded_in_multiple_thread() | |
cpu_bounded_in_multiple_process() | |
io_bounded_in_single_thread() | |
io_bounded_in_multiple_threads() |
Một trong những lý do cho việc thiết kế GIL để lock toàn bộ data mà không phải lock từng phần là giảm số lượng biến lock (atomic value), tránh rơi vào tính trạng deadlock
One of the reasons for designing GIL to lock all data instead of locking each part is to reduce the number of lock variables (atomic value), avoiding falling into deadlock.
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
On Linux
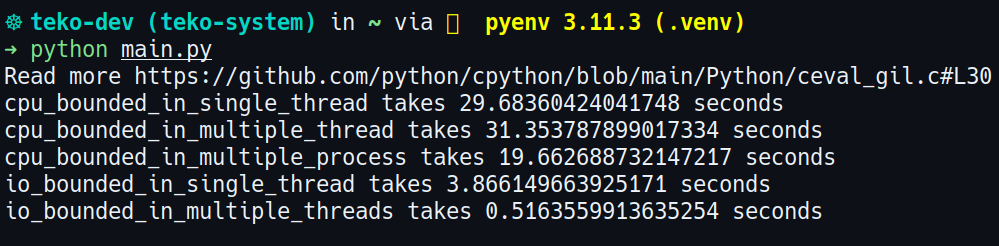