Last active
January 6, 2021 01:36
-
-
Save marble/b02c753abf19368e0f34678601c157f3 to your computer and use it in GitHub Desktop.
render-typo3-viewhelper-docs-from-php-code-whiptail-menu.sh
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#! /bin/bash | |
# This software is MIT licensed. | |
# Available from https://gist.github.com/marble/b02c753abf19368e0f34678601c157f3/edit | |
# Designed for a Linux like system that has the 'whiptail' command installed. | |
# Copyright <2020> <martin.bless@mbless.de> | |
# | |
# Permission is hereby granted, free of charge, to any person obtaining a copy | |
# of this software and associated documentation files (the "Software"), to | |
# deal in the Software without restriction, including without limitation the | |
# rights to use, copy, modify, merge, publish, distribute, sublicense, and/or | |
# sell copies of the Software, and to permit persons to whom the Software is | |
# furnished to do so, subject to the following conditions: | |
# | |
# The above copyright notice and this permission notice shall be included in | |
# all copies or substantial portions of the Software. | |
# | |
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | |
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | |
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | |
# AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | |
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING | |
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS | |
# IN THE SOFTWARE. | |
function press_key() { | |
local opts="$-" | |
echo -e "\n\n"; read -p "Press enter to continue"; echo -e "\n\n" | |
} | |
function step_1_help () { | |
result=$(cat <<- EOT | |
Render TYPO3.CMS ViewHelper documentation | |
========================================= | |
* Run all steps in ascending order choosing "yes". | |
* You may restart the script and run parts repeatedly as you like. | |
Later steps depend on preprevious steps. | |
* This script should not do harm and write to subfolders OTHERREPOS and | |
GENERATED only. It comes with MIT license - use at your own risk. | |
* Use the keys TAB, Enter and Escape for navigation and choice. | |
Enjoy! | |
EOT | |
) | |
} | |
function main() { | |
local opts="$-" | |
local OK=1 | |
local cmd="" | |
local choice="" | |
local rootdir=$(pwd) | |
local otherrepos=$rootdir/OTHERREPOS | |
local generated=$rootdir/GENERATED | |
local backtitle="Render Viewhelper Documentation - or stop" | |
local fluid_documentation_generator=${otherrepos}/fluid-documentation-generator | |
local schemas=${generated}/schemas | |
local typo3cms=${otherrepos}/TYPO3.CMS | |
local t3docs_render_documentation_container="t3docs/render-documentation:develop" | |
local includes_txt=$(cat << EOT | |
.. role:: aspect (emphasis) | |
.. role:: html(code) | |
.. role:: js(code) | |
.. role:: php(code) | |
.. role:: rst(code) | |
.. role:: sep (strong) | |
.. role:: typoscript(code) | |
.. role:: ts(typoscript) | |
:class: typoscript | |
.. role:: yaml(code) | |
.. default-role:: code | |
.. highlight:: php | |
EOT | |
) | |
menuloop | |
} | |
function menuloop() { | |
local opts="$-" | |
while [ 1 ]; do | |
choice=$( | |
whiptail --title "Generate Viewhelper Docs" --menu "Make your choice" 16 100 9 \ | |
"1)" "Help" \ | |
"2)" "Check requirements" \ | |
"3)" "Clean folders" \ | |
"4)" "Git pull repositories" \ | |
"5)" "Run composer" \ | |
"6)" "Generate xsd from php classes" \ | |
"7)" "Generate documentation from xsd" \ | |
"9)" "End script" \ | |
3>&2 2>&1 1>&3 | |
) | |
result="Choosing $choice" | |
case $choice in | |
"1)") | |
step_1_help | |
;; | |
"2)") | |
step_2_check_requirements | |
;; | |
"3)") | |
step_3_clean | |
;; | |
"4)") | |
step_4_git_pull | |
;; | |
"5)") | |
step_5_run_composer | |
;; | |
"6)") | |
step_6_generate_xsd_from_classes | |
;; | |
"7)") | |
step_7_generate_documentation_from_xsd | |
;; | |
"9)") | |
return | |
;; | |
*) | |
result=$choice | |
exit | |
;; | |
esac | |
whiptail --msgbox "$result" 20 78 | |
done | |
} | |
function step_2_check_requirements () { | |
result="" | |
local msg="" | |
local some_missing="" | |
clear | |
echo -e "---> Entering ${FUNCNAME[0]}\n" | |
for tool in \ | |
composer \ | |
docker \ | |
git \ | |
rsync \ | |
whiptail | |
do | |
if which $tool | |
then | |
msg="found..: $tool" | |
else | |
msg="missing: $tool" | |
some_missing=yes | |
fi | |
result="$result\n$msg" | |
done | |
if [ -z "$some_missing" ] | |
then | |
result="${result}\n=========================================\nOk, all tools found.\n" | |
else | |
result="${result}\n=========================================\nSome tools are missing.\n" | |
fi | |
result="Result notes of ${FUNCNAME[0]}\n=========================================\n$result" | |
echo -e "<--- Leaving ${FUNCNAME[0]}" | |
} | |
function step_3_clean () { | |
result="" | |
local opts="$-" | |
local folder="" | |
local rough_requirement="" | |
local shortname="" | |
clear | |
echo -e "---> Entering ${FUNCNAME[0]}\n" | |
for item in \ | |
"$typo3cms OTHERREPOS OTHERREPOS/typo3cms" \ | |
"$fluid_documentation_generator OTHERREPOS OTHERREPOS/fluid_documentation_generator" \ | |
"$schemas GENERATED GENERATED/schemas" | |
do | |
set -- $item | |
folder=$1 | |
rough_requirement=$2 | |
shortname=$3 | |
echo -e "$shortname\n" | |
if [[ "$folder" =~ "$rough_requirement" ]] && [ -d "$folder" ] | |
then | |
if whiptail --yesno "Remove $shortname?" 9 60 | |
then | |
cmd="rm -rf $folder" | |
result="${result}removed $shortname\n" | |
echo "+ $cmd" | |
eval $cmd || exit 1 | |
press_key | |
fi | |
else | |
echo "Nothing to do for this one." | |
fi | |
echo | |
done | |
if [ -z "$result" ] | |
then | |
result="==============================\nNothing was done.\n" | |
else | |
result="${result}\n==============================\nOk, ${FUNCNAME[0]} done.\n" | |
fi | |
result="Result notes of ${FUNCNAME[0]}\n==============================\n$result" | |
echo -e "<--- Leaving ${FUNCNAME[0]}" | |
} | |
function git_pull_typo3cms() { | |
echo -e "---> Entering ${FUNCNAME[0]}\n" | |
if [[ "$typo3cms" =~ OTHERREPOS ]] && [ -d "$typo3cms/.git" ] | |
then | |
cmd="git pull" | |
cd "$typo3cms" | |
echo "git status" | |
git status | |
result="${result}git pull TYPO3.CMS\n" | |
echo Working directory is: $(pwd) | |
echo "$cmd" | |
eval $cmd || exit 1 | |
press_key | |
fi | |
if [[ "$typo3cms" =~ OTHERREPOS ]] && [ ! -d "$typo3cms" ] | |
then | |
cmd="git clone --depth=1 https://github.com/TYPO3/TYPO3.CMS.git $typo3cms" | |
cd $otherrepos | |
result="${result}git clone TYPO3.CMS\n" | |
echo Working directory is: $(pwd) | |
echo "$cmd" | |
eval $cmd || exit 1 | |
press_key | |
fi | |
echo -e "<--- Leaving ${FUNCNAME[0]}" | |
} | |
function git_pull_fluid_documentation_generator() { | |
echo -e "---> Entering ${FUNCNAME[0]}\n" | |
if [[ "$fluid_documentation_generator" =~ OTHERREPOS ]] && [ -d "$fluid_documentation_generator/.git" ] | |
then | |
cmd="git pull" | |
cd "$fluid_documentation_generator" | |
fi | |
if [[ "$fluid_documentation_generator" =~ OTHERREPOS ]] && [ ! -d "$fluid_documentation_generator" ] | |
then | |
cmd="git clone https://github.com/maddy2101/fluid-documentation-generator.git $fluid_documentation_generator" | |
fi | |
if [ ! -z "$cmd" ] | |
then | |
result="${result}git pull fluid-documentation-generator\n" | |
echo Working directory is: $(pwd) | |
echo "$cmd" | |
eval $cmd || exit 1 | |
press_key | |
fi | |
echo -e "<--- Leaving ${FUNCNAME[0]}" | |
} | |
function step_4_git_pull () { | |
result="" | |
local opts="$-" | |
cmd="" | |
clear | |
echo -e "---> Entering ${FUNCNAME[0]}\n" | |
mkdir -p "$otherrepos" | |
for item in \ | |
"OTHERREPOS/typo3cms" \ | |
"OTHERREPOS/fluid_documentation_generator" | |
do | |
if whiptail --yesno "git pull $item" 9 60 | |
then | |
if [[ "$item" == "OTHERREPOS/typo3cms" ]] | |
then | |
git_pull_typo3cms | |
fi | |
if [[ "$item" == "OTHERREPOS/fluid_documentation_generator" ]] | |
then | |
git_pull_fluid_documentation_generator | |
fi | |
fi | |
done | |
cd "$rootdir" | |
if [ -z "$result" ] | |
then | |
result="==============================\nNothing was done.\n" | |
else | |
result="${result}\n==============================\nOk, ${FUNCNAME[0]} done.\n" | |
fi | |
result="Result notes of ${FUNCNAME[0]}\n==============================\n$result" | |
echo -e "<--- Leaving ${FUNCNAME[0]}" | |
} | |
function git_composer_typo3cms() { | |
echo -e "---> Entering ${FUNCNAME[0]}\n" | |
cmd="" | |
if [ -e "$typo3cms"/composer.json ] | |
then | |
if [ -e "$typo3cms"/bin/generateschema ] | |
then | |
cmd="composer install" | |
else | |
cmd="composer require -o -n \"typo3/fluid-schema-generator ^2.1\"" | |
fi | |
result="${result}composer install typo3cms\n" | |
cd "$typo3cms" | |
clear | |
echo "*************************************************" | |
echo "* PLEASE BE PATIENT - this may take a while *" | |
echo "*************************************************" | |
echo -e "\n$cmd" | |
eval $cmd || exit 1 | |
press_key | |
cd "$rootdir" | |
fi | |
echo -e "<--- Leaving ${FUNCNAME[0]}" | |
} | |
function git_composer_fluid_documentation_generator() { | |
echo -e "---> Entering ${FUNCNAME[0]}\n" | |
cmd="" | |
if [ -e "$fluid_documentation_generator"/composer.json ] | |
then | |
cmd="composer install" | |
fi | |
if [ ! -z "$cmd" ] | |
then | |
result="${result}composer install fluid_documentation_generator\n" | |
cd "$fluid_documentation_generator" | |
echo Working directory is: $(pwd) | |
echo "*************************************************" | |
echo "* PLEASE BE PATIENT - this may take a while *" | |
echo "*************************************************" | |
echo -e"\n$cmd" | |
eval $cmd || exit 1 | |
press_key | |
fi | |
echo -e "<--- Leaving ${FUNCNAME[0]}" | |
} | |
function step_5_run_composer () { | |
local opts="$-" | |
result="" | |
cmd="" | |
clear | |
echo -e "---> Entering ${FUNCNAME[0]}\n" | |
for item in \ | |
"OTHERREPOS/typo3cms" \ | |
"OTHERREPOS/fluid_documentation_generator" | |
do | |
if whiptail --yesno "composer install $item" 9 60 | |
then | |
if [[ "$item" == "OTHERREPOS/typo3cms" ]] | |
then | |
git_composer_typo3cms | |
fi | |
if [[ "$item" == "OTHERREPOS/fluid_documentation_generator" ]] | |
then | |
git_composer_fluid_documentation_generator | |
fi | |
fi | |
done | |
cd "$rootdir" | |
if [ -z "$result" ] | |
then | |
result="==============================\nNothing was done.\n" | |
else | |
result="${result}\n==============================\nOk, ${FUNCNAME[0]} done.\n" | |
fi | |
result="Result notes of ${FUNCNAME[0]}\n==============================\n$result" | |
echo -e "<--- Leaving ${FUNCNAME[0]}" | |
} | |
function step_4_get_and_update_fluid-documentation-generator () { | |
local opts="$-" | |
result="" | |
clear | |
echo -e ${FUNCNAME[0]} | |
echo $fluid_documentation_generator | |
ls -la $fluid_fluid_documentation_generator | |
press_key | |
mkdir -p $otherrepos | |
if [[ "$fluid_documentation_generator" =~ OTHERREPOS ]] && [ -d "$fluid_documentation_generator/.git" ] | |
then | |
cd $fluid_documentation_generator | |
cmd="git pull" | |
else | |
cd $otherrepos | |
cmd="git clone https://github.com/maddy2101/fluid-documentation-generator.git" | |
fi | |
if [[ "$fluid_documentation_generator" =~ OTHERREPOS ]] && [ -d $fluid_documentation_generator ] | |
then | |
cmd="composer install" | |
result="${result}$cmd\n" | |
set -x -- ${-:+"-$-"} | |
cd $fluid_documentation_generator | |
eval $cmd || exit 1 | |
cd "$rootdir" | |
set +x "$@" | |
fi | |
if [ -z "$result" ] | |
then | |
result="Nothing was done.\n" | |
else | |
result="${result}\nOk, done.\n" | |
press_key | |
fi | |
result="Result notes of ${FUNCNAME[0]}\n==============================\n$result" | |
press_key | |
} | |
function step_6_generate_xsd_from_classes () { | |
local opts="$-" | |
local errormsg="" | |
local generateschema="$typo3cms"/bin/generateschema | |
local destdir="" | |
result="" | |
cmd="" | |
clear | |
echo -e "---> Entering ${FUNCNAME[0]}\n" | |
if [ ! -e "$generateschema" ] | |
then | |
errormsg="$errormsg\n'$typo3cms/bin/generateschema' is missing." | |
fi | |
if [ ! -z "$errormsg" ] | |
then | |
result=$errormsg | |
return | |
fi | |
cd "$rootdir" | |
schemas=${rootdir}/GENERATED/schemas | |
mkdir -p $schemas | |
rm -rf $schemas/* $schemas/.* >/dev/null 2>&1 | |
for class_target in \ | |
"TYPO3Fluid\\Fluid typo3fluid/fluid/latest "\ | |
"TYPO3\\CMS\\Backend typo3/backend/latest "\ | |
"TYPO3\\CMS\\Core typo3/core/latest "\ | |
"TYPO3\\CMS\\Fluid typo3/fluid/latest "\ | |
"TYPO3\\CMS\\Form typo3/form/latest "\ | |
"TYPO3\\CMS\\IndexedSearch typo3/indexed_search/latest " | |
do | |
set -- $class_target | |
class=$1 | |
target=$2 | |
echo $class_target | |
destdir=${schemas}/${target} | |
mkdir -p "$destdir" | |
cd "$typo3cms" | |
./bin/generateschema "$class" > ${destdir}/schema.xsd || exit 1 | |
result="$result\n$class_target" | |
done | |
if [ -z "$result" ] | |
then | |
result="Nothing was done.\n" | |
else | |
result="${result}\n============================================================\nOk, done.\n" | |
press_key | |
fi | |
result="Result notes of ${FUNCNAME[0]}\n============================================================\n$result" | |
} | |
function step_7_generate_documentation_from_xsd () { | |
local opts="$-" | |
local errormsg="" | |
local generatefluiddocumentation="$fluid_documentation_generator"/bin/generate-fluid-documentation | |
local destdir="" | |
result="" | |
cmd="" | |
clear | |
echo -e "---> Entering ${FUNCNAME[0]}\n" | |
if [ ! -e "$generatefluiddocumentation" ] | |
then | |
errormsg="$errormsg\n'$fluid_documentation_generator/bin/generate-fluid-documenation' is missing." | |
fi | |
if [ ! -d "${fluid_documentation_generator}/schemas" ] | |
then | |
errormsg="$errormsg\nfolder 'fluid_documentation_generator/schemas' is missing" | |
fi | |
if [ ! -d "${generated}/schemas" ] | |
then | |
errormsg="$errormsg\nfolder 'GENERATED/schemas' is missing" | |
fi | |
if [ ! -z "$errormsg" ] | |
then | |
result=$errormsg | |
return | |
fi | |
if [ -z "$errormsg" ] | |
then | |
cd "$rootdir" | |
find $fluid_documentation_generator/schemas -type f -name "*.xsd" -delete | |
rsync -av "$schemas/" "$fluid_documentation_generator/schemas/" | |
cd $fluid_documentation_generator | |
$generatefluiddocumentation | |
result="Done" | |
rsync -av "$fluid_documentation_generator/public/" "$generated/Documentation" | |
echo -e "$includes_txt" >"$generated/Documentation/Includes.txt" | |
# cp -f ${rootdir}/Includes.txt "$generated/Documentation/Includes.txt" | |
cd "$generated" | |
mkdir -p Documentation-GENERATED-temp | |
rm -rf Documentation-GENERATED-temp/* | |
docker run --rm --user=$(id -u):$(id -g) \ | |
-v $(pwd):/PROJECT:ro \ | |
-v $(pwd)/Documentation-GENERATED-temp:/RESULT \ | |
$t3docs_render_documentation_container makehtml-no-cache | |
fi | |
result="$result\nFind the results in:" | |
result="$result\n(1) OTHERREPOS/fluid_documentation_generator/public/" | |
result="$result\n(2)GENERATED/Documentation/ (is a copy of (1))" | |
result="$result\n(3)GENERATED/Documentation-GENERATED-temp/" | |
if [ -z "$result" ] | |
then | |
result="Nothing was done.\n" | |
else | |
result="${result}\n============================================================\nOk, done.\n" | |
press_key | |
fi | |
result="Result notes of ${FUNCNAME[0]}\n============================================================\n$result" | |
} | |
# | |
### do the work | |
main |
It would be great to have some things configurable: e.g. use maddy or namelesscoder generator, build from master or build latest release, build only fluid or TYPO3 Viewhelper etc.
@sypets I hope others feel motivated. I justed wanted to keep somewhere what I had figured out. But I don't really have a clue what's going on there in detail. So yes: Would be could to have that. But no: I'm not the one. Just wanted to show a way!
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Should look like:
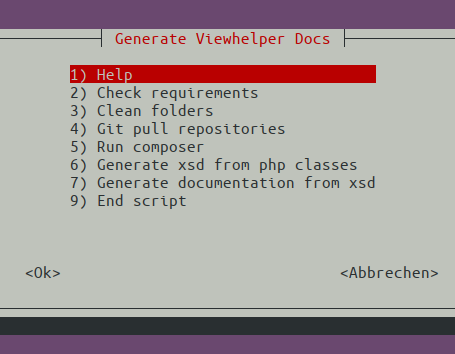