Created
January 20, 2020 15:51
-
-
Save marcuswu/c0aaeca835cd8f442214502cf7f0cd32 to your computer and use it in GitHub Desktop.
Create a taurus and a cube with cylinders cut from it
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const MakerCad = require('../index.js'); | |
const Cube = function (face) { | |
let sketch = MakerCad.Sketch(face); | |
let line1 = sketch.line(sketch.point(0.0, 0.0), sketch.point(5.0, 0.0)); | |
line1.length(10).horizontal(); | |
let line2 = sketch.line(sketch.point(5.0, 0.0), sketch.point(5.0, 6.0)); | |
line2.length(10).vertical(); | |
let line3 = sketch.line(sketch.point(5.0, 5.0), sketch.point(0.0, 6.0)); | |
line3.horizontal(); | |
let line4 = sketch.line(sketch.point(0.0, 5.0), sketch.point(0.0, 1.0)); | |
line4.vertical(); | |
line1.getEnd().coincident(line2.getStart()); | |
line2.getEnd().coincident(line3.getStart()); | |
line3.getEnd().coincident(line4.getStart()); | |
line4.getEnd().coincident(line1.getStart()); | |
let line1Midpoint = sketch.point(0.0, 0.0).vertical(sketch.getOrigin()); | |
let line2Midpoint = sketch.point(0.0, 0.0).horizontal(sketch.getOrigin()); | |
line1.midpoint(line1Midpoint); | |
line2.midpoint(line2Midpoint); | |
let squareFace = MakerCad.Face(sketch); | |
return squareFace.extrude(10); | |
}; | |
const Cylinder = function (face, shapes = [], operation = MakerCad.MergeType.New) { | |
let sketch = MakerCad.Sketch(face); | |
let holeCenter = sketch.point(0, 0); | |
holeCenter | |
.horizontalDistance(sketch.getOrigin(), 0) | |
.verticalDistance(sketch.getOrigin(), 0); | |
sketch.circle(holeCenter, 9).diameter(9); | |
let cylinderFace = MakerCad.Face(sketch); | |
return cylinderFace.extrude(10, operation, shapes); | |
}; | |
const Taurus = function(face) { | |
let sketch = MakerCad.Sketch(face); | |
let center = sketch.point(25, 5) | |
.horizontalDistance(sketch.getOrigin(), 25) | |
.verticalDistance(sketch.getOrigin(), 5); | |
sketch.circle(center, 10).diameter(10); | |
let axis = sketch.line( | |
sketch.point(0.0, 0.0), | |
sketch.point(0.0, 0.5) | |
); | |
axis.construction(true) | |
.length(5.0) | |
.vertical(); | |
axis.getStart().coincident(sketch.getOrigin()); | |
let taurusFace = MakerCad.Face(sketch); | |
return taurusFace.revolve(axis, Math.PI * 2); | |
}; | |
let cubeOperation = Cube(MakerCad.Plane(MakerCad.Base.Top)); | |
let topFace = MakerCad.stream([cubeOperation]) | |
.faces() | |
.filter(f => f.isAlignedNormal(MakerCad.Plane(MakerCad.Base.Top))) | |
.collectList()[0]; | |
let cylinderOperation1 = Cylinder(topFace, [cubeOperation.shape()], MakerCad.MergeType.Remove); | |
frontFace = MakerCad.stream([cylinderOperation1]) | |
.faces() | |
.filter(f => f.isAlignedNormal(MakerCad.Plane(MakerCad.Base.Front))) | |
.collectList()[0]; | |
let cylinderOperation2 = Cylinder(frontFace, [cylinderOperation1.shape()], MakerCad.MergeType.Remove); | |
rightFace = MakerCad.stream([cylinderOperation2]) | |
.faces() | |
.filter(f => f.isAlignedNormal(MakerCad.Plane(MakerCad.Base.Right))) | |
.collectList()[0]; | |
let cylinderOperation3 = Cylinder(rightFace, [cylinderOperation2.shape()], MakerCad.MergeType.Remove); | |
let taurusOperation = Taurus(MakerCad.Plane(MakerCad.Base.Front)); | |
let exportOperations = [cylinderOperation3, taurusOperation]; | |
let shapes = MakerCad.streamOf(exportOperations).shapes().collectList(); | |
console.log('exporting shapes', shapes); | |
MakerCad.exportStl("test.stl", shapes); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
The above source produces the following model (saved as "test.stl"):
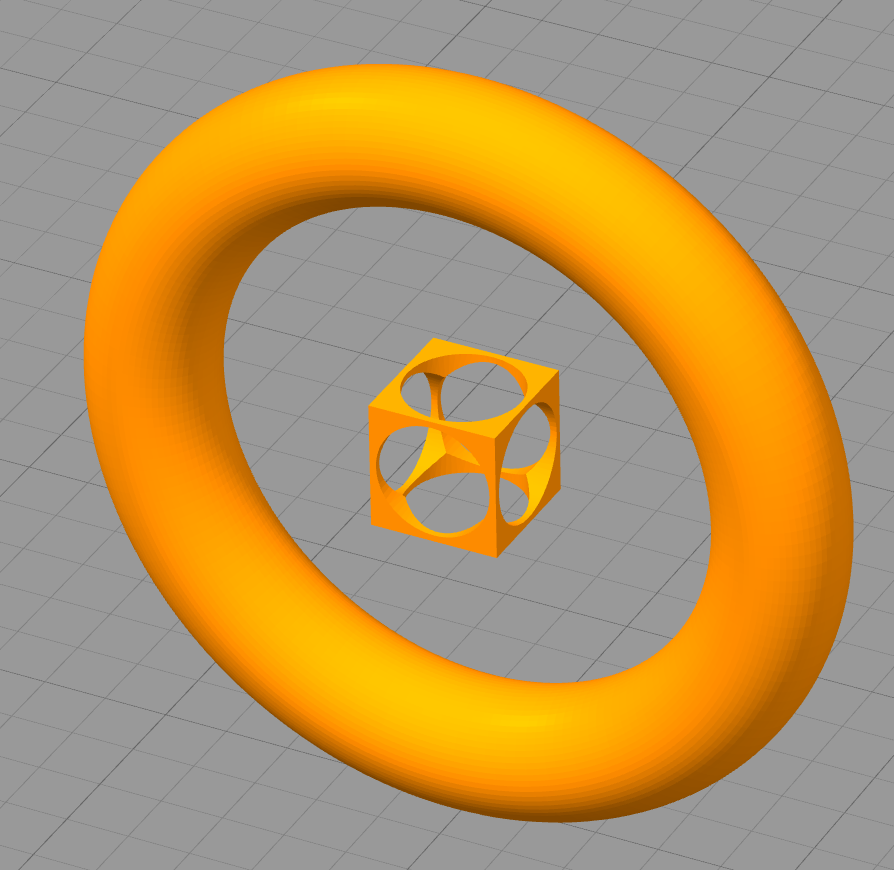