Last active
May 11, 2020 12:35
-
-
Save markusmo3/ee46e5fe81d4dacea7110134f4ca953f to your computer and use it in GitHub Desktop.
An IntelliJ IDEA Live Plugin to change the window icon to something more useful. (LivePlugin: https://github.com/dkandalov/live-plugin)
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import com.intellij.ide.plugins.PluginUtil | |
import java.awt.* | |
import javax.imageio.* | |
import com.intellij.openapi.project.* | |
import com.intellij.openapi.wm.* | |
import com.intellij.openapi.wm.impl.* | |
import sun.misc.* | |
import java.awt.image.BufferedImage | |
import static liveplugin.PluginUtil.invokeLaterOnEDT | |
import static liveplugin.PluginUtil.registerProjectListener | |
invokeLaterOnEDT { | |
IdeFrameImpl.frames.each { Frame frame -> | |
if (frame instanceof IdeFrameImpl) { | |
def project = frame.getProject() | |
println("frame found $frame $project") | |
setIdeaWindowIcon(project, frame, getIdentifyingLetters(project)) | |
} | |
} | |
registerProjectListener("icon-changer-live", new ProjectManagerListener() { | |
@Override | |
void projectOpened(Project project) { | |
invokeLaterOnEDT { | |
setIdeaWindowIcon(project, getIdentifyingLetters(project)) | |
} | |
} | |
}) | |
} | |
static def setIdeaWindowIcon(Project project, IdeFrameImpl frame = findFrameForProject(project), | |
String overlayText) { | |
if (frame == null) { | |
return | |
} | |
def moreThanTwoChars = overlayText.length() > 2 | |
def img = toBufferedImage(frame.getIconImage()) | |
def g = img.createGraphics() | |
g.color = Color.BLACK | |
g.fillRect(5, 5, 20, 16) | |
g.color = Color.WHITE | |
g.font = new Font("Consolas", Font.BOLD, moreThanTwoChars ? 13 : 15) | |
g.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_OFF) | |
g.setRenderingHint(RenderingHints.KEY_TEXT_ANTIALIASING, RenderingHints.VALUE_TEXT_ANTIALIAS_ON) | |
g.drawString(overlayText, | |
moreThanTwoChars ? 5 : 6, | |
moreThanTwoChars ? 14 : 16) | |
frame.setIconImage(img) | |
} | |
static String getIdentifyingLetters(Project project, int i = 0) { | |
if (project == null) { | |
return "???" | |
} | |
def prjName = project.name | |
if (i >= Constants.identLettersSplitRegexes.length) { | |
return prjName.substring(0, Math.min(3, prjName.length())) | |
} | |
def regex = Constants.identLettersSplitRegexes[i] | |
def split = prjName.split(regex) - "" | |
if (split.length >= 3) { | |
return split[0].substring(0, 1) + split[1].substring(0, 1) + split[2].substring(0, 1) | |
} else if (split.length == 2) { | |
return split[0].substring(0, 1) + split[1].substring(0, 1) | |
// } else if (split.length == 1) { | |
// return split[0].substring(0, 1) | |
} else { | |
return getIdentifyingLetters(project, i + 1) | |
} | |
} | |
static IdeFrame findFrameForProject(Project project) { | |
for (Frame frame : IdeFrameImpl.frames) { | |
if (frame instanceof IdeFrame && frame.getProject() == project) { | |
return frame | |
} | |
} | |
return null | |
} | |
/** | |
* Converts a given Image into a BufferedImage | |
* | |
* @param img The Image to be converted | |
* @return The converted BufferedImage | |
*/ | |
public static BufferedImage toBufferedImage(Image img) { | |
if (img instanceof BufferedImage) { | |
return (BufferedImage) img; | |
} | |
// Create a buffered image with transparency | |
BufferedImage bimage = new BufferedImage(img.getWidth(null), img.getHeight(null), BufferedImage.TYPE_INT_ARGB); | |
// Draw the image on to the buffered image | |
Graphics2D bGr = bimage.createGraphics(); | |
bGr.drawImage(img, 0, 0, null); | |
bGr.dispose(); | |
// Return the buffered image | |
return bimage; | |
} | |
class Constants { | |
static String ideaIconWithBar_base64_28px = "iVBORw0KGgoAAAANSUhEUgAAABwAAAAcCAYAAAByDd+UAAAAAXNSR0IArs4c6QAAAARnQU1BAACxjwv8YQUAAAAJcEhZcwAADsQAAA7EAZUrDhsAAAASdEVYdFNvZnR3YXJlAEdyZWVuc2hvdF5VCAUAAANSSURBVEhLtVZBaxNBFP5md5ukSdqKjUhLpV4FsRepHix4EEEPgt4sHrwoHoqIgqAULx4EqT/BPyFaFA+ilyJFLHjQQotCtQ1FbZs0abPZ2fF7s5tYZbWXzYMvM3n7Zr/33rydeWp+9KLJOC52iiG0UoSDkAgImcsYItIL5nqG8KR0hBpAnpj4meFasQupDzmX//xBGDahPh+/ZIZzReH5Q1qLd5L9PQpm+w7iWekwtp2uiIQ6IdF2HhNTJ+/bqizTsVhCY+CHIRqhtvDDAE3dRCCgZ3YktIUfj02MrC3iwspbeM0tGOqNbsAEDShBcxuKOofvi3KDKMID2QK2jcYZMx+xpyzFkUeAl0NtsxxFKLne0gFzHHYEJtiCw4BcZjFOqUHT8AEVnYBi6h2+3+G8vYciSd6lAdm/39ghScZpwEbWQsxla0hr3REIUbR/JJdvqeF48Ikk4zQQRRal02lkgIbrWiSlIw24LEohrfLTcOpjc1g9PYevJxcSvUsDYIVWSFZ1siRmmHLs2PMzwTgN1LwsfnhFeySS0B44EnSicRooZ3rhc8vahFKhnYzQFqQExMPcEraQZJwG7O3B6LRyfxNKWoMg6Agisr8i1MZLNE4DcjcKoWxdu2hWy4cS05EKSNa6uEkYfRa1yv5E79JAq2BkVG8mj5psfgDv5k7g9vR90gODg4OYmpqy83/J+Ph4PNtdeq/MQuX7EVTLUK8nR029MYovCwdx68U9azA0NIT5+f/f/oVCIZ7tLj3X3seEK1CvJo+Z2uYYVj7vx8TzSWswPDxso/yfzMzMxLPdpXfiA5AvQQvhyztjprp2CuvL3bg6fTc2SVd6bnwiYb8ldHy/gOrGgC2cpJM+FSjPtooC9fTmWfNz+Ty0X0elUWMzxRaQpWukd6UTtortSaHYe3pYyhexWOxDNcMuzC2gjhx8k4Unl4LYiuMSFu0NG2yT4V53l2CyPQgr36AeX75usttHSMIzj99MwKtEFlgyWcRRyFaLGXzcuwffu9hSIsP7M4NNVcQm8uxjs3CF0Nr/udawQTZdOTvqjSWoB+ce0r/Ys3ZUrQOd3XJBY2Wfg6qbw7qXR83kbVR1EtVMN2roZtMc3XPtdSQTab3D3vdUqdDHLz+773FTc2IpAAAAAElFTkSuQmCC" | |
static String[] identLettersSplitRegexes = ["[a-z0-9_-]+", "[0-9_-]+"] | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Showcase:
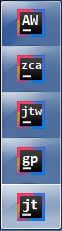