Last active
June 12, 2023 17:49
-
-
Save marph91/fde8307819b3001b6155e55220aac062 to your computer and use it in GitHub Desktop.
Script for generating an user API key for discourse.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
""" | |
Script for generating an user API key for discourse. | |
Requirements: | |
pip install pycryptodomex | |
Usage: | |
python generate_api_key.py --help | |
python generate_api_key.py https://meta.discourse.org | |
""" | |
import argparse | |
from base64 import b64decode | |
import json | |
from secrets import token_urlsafe | |
from urllib.parse import urlencode | |
from Cryptodome.Cipher import PKCS1_v1_5 | |
from Cryptodome.PublicKey import RSA | |
from Cryptodome.Random import get_random_bytes | |
def generate_api_key(host): | |
# generate RSA key | |
key = RSA.generate(2048) | |
# assemble the url | |
path = "/user-api-key/new" | |
query_dict = { | |
"application_name": "python", | |
"client_id": token_urlsafe(), | |
"scopes": "read", | |
"public_key": key.publickey().export_key().decode(), | |
"nonce": 1, | |
} | |
query = urlencode(query_dict) | |
url = f"{host}{path}?{query}" | |
print(f"Navigate to {url} and copy the generated ciphertext in here:\n") | |
ciphertext = input() | |
# process the ciphertext from discourse | |
sentinel = get_random_bytes(16) | |
cipher_rsa = PKCS1_v1_5.new(key) | |
plaintext = cipher_rsa.decrypt(b64decode(ciphertext), sentinel) | |
plaintext = json.loads(plaintext.decode()) | |
return plaintext["key"] | |
def main(): | |
parser = argparse.ArgumentParser() | |
parser.add_argument("host", type=str, help="Hostname of the discourse server.") | |
args = parser.parse_args() | |
api_key = generate_api_key(args.host) | |
print("\nUser API key:", api_key) | |
if __name__ == "__main__": | |
main() |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
same problem here.
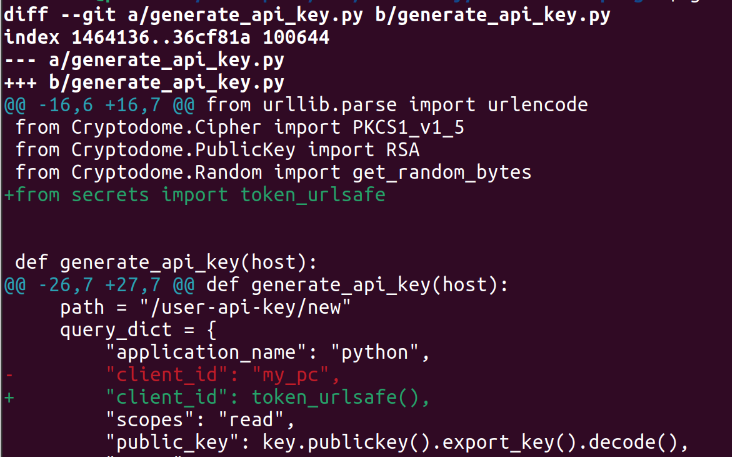
the problem is the client_id. This is how to fix it: