Created
June 7, 2019 16:28
-
-
Save mathigatti/439a0e81556f2698c7db4f41189d201f to your computer and use it in GitHub Desktop.
Convert an input number into a pattern (The number is interpreted as the rules for a 2D Grid Cellular automata with Von Neumann neighbourhood)
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import numpy as np | |
import cv2 | |
ON = 255 | |
OFF = 0 | |
N = 200 | |
def rule(neighbours,rules): | |
if neighbours in rules: | |
return ON | |
else: | |
return OFF | |
def update(grid,rules): | |
newGrid = grid.copy() | |
for i in range(1,N-1): | |
for j in range(1,N-1): | |
newGrid[i,j] = rule((grid[i-1,j],grid[i,j+1],grid[i+1,j],grid[i,j-1]),rules) | |
return newGrid | |
def toTupla(n): | |
neighbours = [] | |
n = "{0:b}".format(n).zfill(4) | |
for c in n: | |
if c == "0": | |
neighbours.append(OFF) | |
else: | |
neighbours.append(ON) | |
return tuple(neighbours) | |
def toNumber(tupla): | |
n = '' | |
for t in tupla: | |
if t == ON: | |
n += "1" | |
else: | |
n += "0" | |
return int(n,2) | |
def generate(n): | |
tuplas = [] | |
i = 0 | |
for c in n[::-1]: | |
if c == "1": | |
tuplas.append(toTupla(i)) | |
i+=1 | |
return tuplas | |
def numberToAutomata(number, steps): | |
grid = np.random.choice([ON, OFF], N*N, p=[0.5, 0.5]).reshape(N, N) | |
index = "{0:b}".format(number).zfill(256) | |
rules = generate(index) | |
for step_index in range(steps): | |
grid = update(grid,rules) | |
cv2.imwrite('images/'+str(number)+'.jpg', grid) | |
cv2.imwrite('images/'+str(number)+'.tiff', grid) | |
cv2.imwrite('images/'+str(number)+'.png', grid) | |
import sys | |
# Usage example | |
# python number2pattern.py 108780 30 | |
numberToAutomata(int(sys.argv[1]),int(sys.argv[2])) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Pattern for number 108780 with 30 iterations
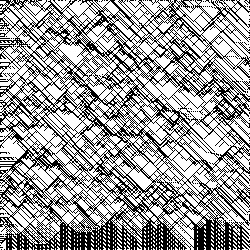