-
-
Save mebjas/729c5397506a879ec704075c8a5284e8 to your computer and use it in GitHub Desktop.
<html> | |
<head> | |
<title>Html-Qrcode Demo</title> | |
<body> | |
<div id="qr-reader" style="width:500px"></div> | |
<div id="qr-reader-results"></div> | |
</body> | |
<script src="https://raw.githubusercontent.com/mebjas/html5-qrcode/master/minified/html5-qrcode.min.js"></script> | |
<script src="html5-qrcode-demo.js"></script> | |
</head> | |
</html> |
function docReady(fn) { | |
// see if DOM is already available | |
if (document.readyState === "complete" || document.readyState === "interactive") { | |
// call on next available tick | |
setTimeout(fn, 1); | |
} else { | |
document.addEventListener("DOMContentLoaded", fn); | |
} | |
} | |
docReady(function() { | |
var resultContainer = document.getElementById('qr-reader-results'); | |
var lastResult, countResults = 0; | |
var html5QrcodeScanner = new Html5QrcodeScanner( | |
"qr-reader", { fps: 10, qrbox: 250 }); | |
function onScanSuccess(decodedText, decodedResult) { | |
if (decodedText !== lastResult) { | |
++countResults; | |
lastResult = decodedText; | |
console.log(`Scan result = ${decodedText}`, decodedResult); | |
resultContainer.innerHTML += `<div>[${countResults}] - ${decodedText}</div>`; | |
// Optional: To close the QR code scannign after the result is found | |
html5QrcodeScanner.clear(); | |
} | |
} | |
// Optional callback for error, can be ignored. | |
function onScanError(qrCodeError) { | |
// This callback would be called in case of qr code scan error or setup error. | |
// You can avoid this callback completely, as it can be very verbose in nature. | |
} | |
html5QrcodeScanner.render(onScanSuccess, onScanError); | |
}); |
Hi mebjas,
I am more or less new to web development. I tried your example but I get error:
scan:57 Uncaught ReferenceError: Html5QrcodeScanner is not defined
I have put in Handlebars layout:
<script src="https://unpkg.com/html5-qrcode" type="text/javascript">
and in handlebars view this:
QR Code Scanner
I know it must be some 'basic' problem... Can you help?
hi mebjas, I tried using all ur format, but anytime I display it on browser(localhost) everything is always blank....no camera showing
Good evening. Is there a way to set it on image reader by default instead of scan first?
I seem to have an issue when the qrcode scanner is kept in between the
. When the scanner starts it prompts the permission with REQUEST CAMERA PERMISSIONS button. but since the button is not having any type"button" attribute by default, it is posting the form automatically which is unintended. how can the type="button" be added to prevent it? Thanks@mwambajoshua for now, you can do this
function validURL(str) { var pattern = new RegExp('^(https?:\\/\\/)?'+ // protocol '((([a-z\\d]([a-z\\d-]*[a-z\\d])*)\\.)+[a-z]{2,}|'+ // domain name '((\\d{1,3}\\.){3}\\d{1,3}))'+ // OR ip (v4) address '(\\:\\d+)?(\\/[-a-z\\d%_.~+]*)*'+ // port and path '(\\?[;&a-z\\d%_.~+=-]*)?'+ // query string '(\\#[-a-z\\d_]*)?$','i'); // fragment locator return !!pattern.test(str); } function onScanSuccess(qrCodeMessage) { if (validURL(qrCodeMessage)) { window.location.href = qrCodeMessage; } } var html5QrcodeScanner = new Html5QrcodeScanner( "qr-reader", { fps: 10, qrbox: 250 }); html5QrcodeScanner.render(onScanSuccess);
@mebjas how can i add this script only the back camara? html5QrcodeScanner.render({ facingMode: { exact:"environment" } }, onScanSuccess); i cant :(
@mebjas please
- how can i change the text?. For example "Request Camera permission" to be changed to something else.
- By default when the render is called, there is a "Scan an Image File", how can i remove this?.
Nice QR code reader.. but... Does anyone know what permissions you need to add to Android Studio to get this to work within an Android app (as a webview)? I've added the camera and write external permissions.. but within the app it says "NotAllowedError: Permission denied". Though if I load up the url within Chrome it works fine. (The url is https)
Thanks.
are you able to use this in the web view ??
@mebjas first of all thank you very much for your this lovely project. I have some questions but right before that, I want to tell what I am trying to do. I have an inventory management system website. And while user is adding the product, I want the user to scan qr code as product id and write it into a text box there so it can be saved to mysql with that ID from qr code. For this I have added a button there which is opening a new window with this qr code scanner I do see it is reading the qr code as I can see green corners but I dont know how I can get that code to that text box which is located on the previous page. And also I want this pop up closes automatically after reading the qr code and get back to previous page. How can this be possible. I am also a new developer so it is a bit complicated for me to understand some parts of your project. So can you help me with this with code examples? Or maybe you can advice me an different way to do this instead of a pop up window?
Thank you for your help
Nice QR code reader.. but... Does anyone know what permissions you need to add to Android Studio to get this to work within an Android app (as a webview)? I've added the camera and write external permissions.. but within the app it says "NotAllowedError: Permission denied". Though if I load up the url within Chrome it works fine. (The url is https)
Thanks.
Hey did you find any solution? I am still looking for the answer?
Nice QR code reader.. but... Does anyone know what permissions you need to add to Android Studio to get this to work within an Android app (as a webview)? I've added the camera and write external permissions.. but within the app it says "NotAllowedError: Permission denied". Though if I load up the url within Chrome it works fine. (The url is https)
Thanks.are you able to use this in the web view ??
Hey did you find any solution? I am so confused.
I believe it has nothing to do with Android Studio, this plugin is somehow not interacting with the Web View. Still looking for answers.
Nice QR code reader.. but... Does anyone know what permissions you need to add to Android Studio to get this to work within an Android app (as a webview)? I've added the camera and write external permissions.. but within the app it says "NotAllowedError: Permission denied". Though if I load up the url within Chrome it works fine. (The url is https)
Thanks.
I am facing the same issue and having little progress with the solution provided here > https://stackoverflow.com/a/61609282/4679429
Basically you also need to further grant the camera permission {RESOURCE_VIDEO_CAPTURE} within webview. Include following code in the WebChromeClient().
@OverRide
public void onPermissionRequest(final PermissionRequest request) {
final String[] requestedResources = request.getResources();
for (String r : requestedResources) {
if (r.equals(PermissionRequest.RESOURCE_VIDEO_CAPTURE)) {
request.grant(new String[]{PermissionRequest.RESOURCE_VIDEO_CAPTURE});
break;
}
}
}
It open the camera successfully now but then another issue comes, which is the camera view won't move (freeze) when I moving the phone and the camera view only update when I touch any place on phone screen. Does anyone has idea on this issue ?
Updated:
I found the solution for the aforementioned issue (camera freeze), basically just need to add an animated SVG into the background of the camera to keep the android webview updated > mebjas/html5-qrcode#659
What a great tool!
Like others, I keep wondering if there's a way to prioritize the back camera, I never have the QR code on my face, it's always in front of me so I want to use the back camera first.
Yes, as @tacman says, it would be nice to prioritize back camera so that I don't need camera selection list.
Hi mebjas
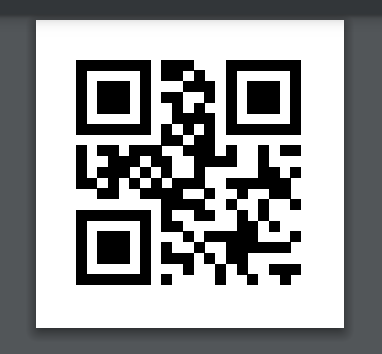
I found the one problem, I ecoded "1" to QR code, and this what I can't scan, it can't decode. Can you tell me where in the library I can see if I can fix it, thank you!