Main reference: https://github.com/csurfer/pyheatmagic
See also https://stackoverflow.com/a/52461806/9746916
Step 1. Install py-heat-magic
%pip install py-heat-magic # run this only once for installation
Step 2. Load the heat
module
%load_ext heat
Step 3. Use %%heat
inside the cell with your codes
%%heat
import numpy as np
def multiply(a, b):
""" Matrix multiplication """
assert a.shape[1] == b.shape[0], 'a columns should match b rows'
c = np.zeros((a.shape[0], b.shape[1]))
# C_ij = sum_k a_ik b_kj
for i in range(a.shape[0]):
for j in range(b.shape[1]):
sum_k = 0
for k in range(a.shape[1]):
sum_k += a[i, k]*b[k, j]
c[i, j] = sum_k
return c
a = np.array([[1, 2, 3, 4, 5, 6, 7, 8],
[-1, -2, -3, -4, -5, -6, -7, -8]])
b = np.array([[1., 1.],
[1., 1.],
[1., 1.],
[1., 1.],
[1., 1.],
[1., 1.],
[1., 1.],
[1., 1.]])
c = multiply(a, b)
You will get something like this:
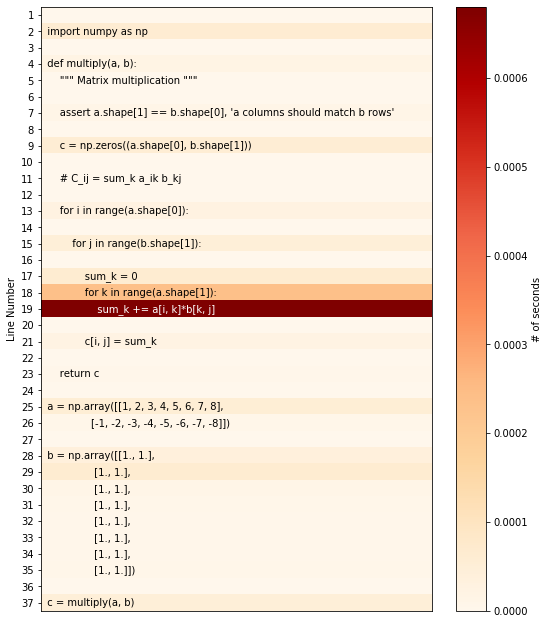