Last active
September 9, 2018 19:15
-
-
Save mencarellic/fe2c16fe982151e6089c3b3eefd15060 to your computer and use it in GitHub Desktop.
fashion-mnist-test-check
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import numpy as np | |
np.random.seed(1000) | |
from matplotlib import pyplot as plt | |
import seaborn as sns | |
sns.set(style="whitegrid") | |
sns.set_palette((sns.color_palette('colorblind', 8))) | |
dims = (11.7, 8.27) | |
%matplotlib inline | |
import math | |
import random | |
def int_to_desc(i): | |
conv = {0: 'T-shirt/top', 1: 'Trouser', 2: 'Pullover', 3: 'Dress', 4: 'Coat', 5: 'Sandal', | |
6: 'Shirt', 7: 'Sneaker', 8: 'Bag', 9: 'Ankle boot'} | |
try: | |
ret = conv[i] | |
except: | |
ret = 'Unknown' | |
return ret | |
def check_random(n, x, y, p): | |
rows = math.ceil(n/5) | |
fig, ax = plt.subplots(nrows=rows, ncols=5, sharex=True, sharey=True,) | |
ax = ax.flatten() | |
for i in range(n): | |
j = random.randint(0,len(p)-1) | |
img = x[j].reshape(28, 28) | |
ax[i].imshow(img, cmap='Greys', interpolation='nearest') | |
predicted = int_to_desc(p[j]) | |
actual = int_to_desc(y[j]) | |
ax[i].set_title('P: {}\n A: {}'.format(predicted,actual)) | |
ax[0].set_xticks([]) | |
ax[0].set_yticks([]) | |
plt.tight_layout() | |
plt.show() | |
## N = number of random items to pull | |
## x = your x test set | |
## y = the y test set | |
## p = the results from the prediction function | |
check_random(n=15, x=X_test, y=ytest_flat, p=pred_flat) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Sample output:
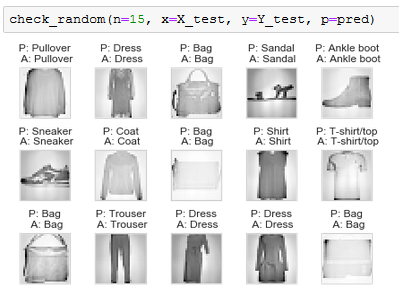