Created
March 6, 2019 12:09
-
-
Save meyt/e05d70c05b4c232bf49f5abd644dc577 to your computer and use it in GitHub Desktop.
vuetify + vue-the-mask component
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<template> | |
<div :class="classes"> | |
<label>{{ label }}</label> | |
<div class="input-group__input"> | |
<span | |
v-if="prefix" | |
class="input-group--text-field__prefix" | |
v-text="prefix" | |
/> | |
<the-mask | |
ref="textInput" | |
type="text" | |
v-model="localValue" | |
:mask="mask" | |
:tokens="tokens" | |
@focus.native="onFocus" | |
@blur.native="onBlur" | |
/> | |
<span | |
v-if="suffix" | |
class="input-group--text-field__suffix" | |
v-text="suffix" | |
/> | |
<i aria-hidden="true" class="icon material-icons input-group__append-icon input-group__icon-cb" @click="appendIconCb()">{{ appendIcon }}</i> | |
</div> | |
<div class="input-group__details"> | |
<div v-if="errorMessages !== null && errorMessages.length > 0" class="input-group__messages input-group__error"> | |
{{ errorMessages[errorMessages.length - 1] }} | |
</div> | |
</div> | |
</div> | |
</template> | |
<script> | |
import {TheMask} from 'vue-the-mask' | |
export default { | |
components: { | |
TheMask | |
}, | |
props: { | |
value: { | |
type: String | |
}, | |
label: { | |
type: String | |
}, | |
prefix: { | |
type: String, | |
default: null | |
}, | |
prefixValue: { | |
type: String, | |
default: '' | |
}, | |
suffix: { | |
type: String, | |
default: null | |
}, | |
appendIcon: { | |
type: String, | |
default: null | |
}, | |
appendIconCb: { | |
type: Function, | |
default () {} | |
}, | |
mask: { | |
type: String, | |
default: '#### — #### — #### — ####' | |
}, | |
errorMessages: { | |
type: Array, | |
default: null | |
}, | |
formatNumbers: { | |
type: Function, | |
default (value) { return value } | |
} | |
}, | |
data () { | |
return { | |
localValue: '', | |
tokens: { | |
'#': { | |
pattern: /[0-9۰-۹]/, | |
transform: this.formatNumbers | |
} | |
}, | |
isFocused: false | |
} | |
}, | |
watch: { | |
value (newVal, oldVal) { | |
if (newVal === oldVal) return | |
if (newVal.startsWith(this.prefixValue)) { | |
newVal = newVal.substr(this.prefixValue.length) | |
} | |
this.localValue = newVal | |
}, | |
localValue (newVal, oldVal) { | |
if (newVal === oldVal) return | |
this.$emit('input', this.prefixValue + newVal) | |
} | |
}, | |
computed: { | |
classes () { | |
const result = ['ltr-input input-group input-group--text-field'] | |
if (this.errorMessages !== null && this.errorMessages.length > 0) { | |
result.push('error--text input-group--error') | |
} | |
if (this.appendIcon) { | |
result.push('input-group--append-icon') | |
} | |
if (this.isFocused || this.localValue.length > 0) { | |
result.push('input-group--focused focused') | |
} | |
if (this.suffix) { | |
result.push('input-group--suffix') | |
} | |
if (this.prefix) { | |
result.push('input-group--prefix') | |
} | |
return result | |
} | |
}, | |
methods: { | |
focus () { | |
this.$refs.textInput.$el.focus() | |
}, | |
onBlur (e) { | |
this.isFocused = false | |
this.$emit('blur', e) | |
}, | |
onFocus (e) { | |
this.isFocused = true | |
this.$emit('focus', e) | |
} | |
} | |
} | |
</script> |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Normal:
Error
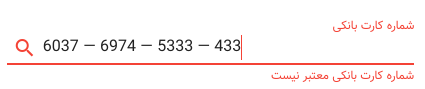
Prefix
