-
-
Save mithunsatheesh/8adad40b059866e892ed to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
//require the mongoClient from mongodb module | |
var MongoClient = require('mongodb').MongoClient; | |
//mongodb configs | |
var connectionUrl = 'mongodb://localhost:27017/myproject', | |
sampleCollection = 'chapters'; | |
//We need to insert these chapters into mongoDB | |
var chapters = [{ | |
'Title': 'Snow Crash', | |
'Author': 'Neal Stephenson' | |
},{ | |
'Title': 'Snow Crash', | |
'Author': 'Neal Stephenson' | |
}]; | |
MongoClient.connect(connectionUrl, function(err, db) { | |
console.log("Connected correctly to server"); | |
// Get some collection | |
var collection = db.collection(sampleCollection); | |
collection.insert(chapters,function(error,result){ | |
//here result will contain an array of records inserted | |
if(!error) { | |
console.log("Success :"+result.ops.length+" chapters inserted!"); | |
} else { | |
console.log("Some error was encountered!"); | |
} | |
db.close(); | |
}); | |
}); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
I needed to create a mongodb atlas database, you can do it for free and create a cluster and you can get the connection by choosing drivers option
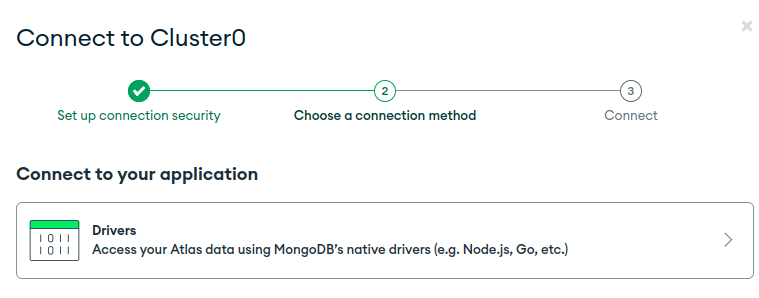