-
-
Save mjclemente/e13995c29376f0924eb2eacf98eaa5a6 to your computer and use it in GitHub Desktop.
NestJS Integration/E2E Testing Example with TypeORM, Postgres, JWT
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import { Test, TestingModule } from '@nestjs/testing' | |
import { INestApplication, LoggerService } from '@nestjs/common' | |
import * as request from 'supertest' | |
import { AppModule } from './../src/app.module' | |
class TestLogger implements LoggerService { | |
log(message: string) {} | |
error(message: string, trace: string) {} | |
warn(message: string) {} | |
debug(message: string) {} | |
verbose(message: string) {} | |
} | |
describe('AppController (e2e)', () => { | |
let app: INestApplication | |
beforeAll(async () => { | |
const moduleFixture: TestingModule = await Test.createTestingModule({ | |
imports: [AppModule], | |
}).compile() | |
app = moduleFixture.createNestApplication() | |
app.useLogger(new TestLogger()) | |
await app.init() | |
// tip: access the database connection via | |
// const connection = app.get(Connection) | |
// const a = connection.manager | |
}) | |
afterAll(async () => { | |
await Promise.all([ | |
app.close(), | |
]) | |
}) | |
it('/ (GET)', () => { | |
return request(app.getHttpServer()) | |
.get('/') | |
.expect(200) | |
.expect('Hello World!') | |
}) | |
describe('Authentication', () => { | |
let jwtToken: string | |
describe('AuthModule', () => { | |
// assume test data includes user test@example.com with password 'password' | |
it('authenticates user with valid credentials and provides a jwt token', async () => { | |
const response = await request(app.getHttpServer()) | |
.post('/auth/login') | |
.send({ email: 'test@example.com', password: 'password' }) | |
.expect(200) | |
// set jwt token for use in subsequent tests | |
jwtToken = response.body.accessToken | |
expect(jwtToken).toMatch(/^[A-Za-z0-9-_=]+\.[A-Za-z0-9-_=]+\.?[A-Za-z0-9-_.+/=]*$/) // jwt regex | |
}) | |
it('fails to authenticate user with an incorrect password', async () => { | |
const response = await request(app.getHttpServer()) | |
.post('/auth/login') | |
.send({ email: 'test@example.com', password: 'wrong' }) | |
.expect(401) | |
expect(response.body.accessToken).not.toBeDefined() | |
}) | |
// assume test data does not include a nobody@example.com user | |
it('fails to authenticate user that does not exist', async () => { | |
const response = await request(app.getHttpServer()) | |
.post('/auth/login') | |
.send({ email: 'nobody@example.com', password: 'test' }) | |
.expect(401) | |
expect(response.body.accessToken).not.toBeDefined() | |
}) | |
}) | |
describe('Protected', () => { | |
it('gets protected resource with jwt authenticated request', async () => { | |
const response = await request(app.getHttpServer()) | |
.get('/protected') | |
.set('Authorization', `Bearer ${jwtToken}`) | |
.expect(200) | |
const data = response.body.data | |
// add assertions that reflect your test data | |
// expect(data).toHaveLength(3) | |
}) | |
}) | |
}) | |
}) |
I forked this from another gist - https://gist.github.com/firxworx/575f019c5ebd67976da164f48c2f4375
Best to check with the original. Sorry I can't be of more help.
thank you sir , problem got solved
Change line 9 src to the relative directory... e.g ../../
Change line 9 src to the relative directory... e.g ../../
For Future reference
solution
In package.json should update
"jest":{
//
"moduleNameMapper": {
"^src/(.*)$": "<rootDir>/../src/$1"
}
}
Your jest config needs to have the following added:
{
//
"transform": {
"^.+\\.(t|j)s$": ["ts-jest"]
},
"moduleDirectories": ["<rootDir>/../", "node_modules"]
}
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
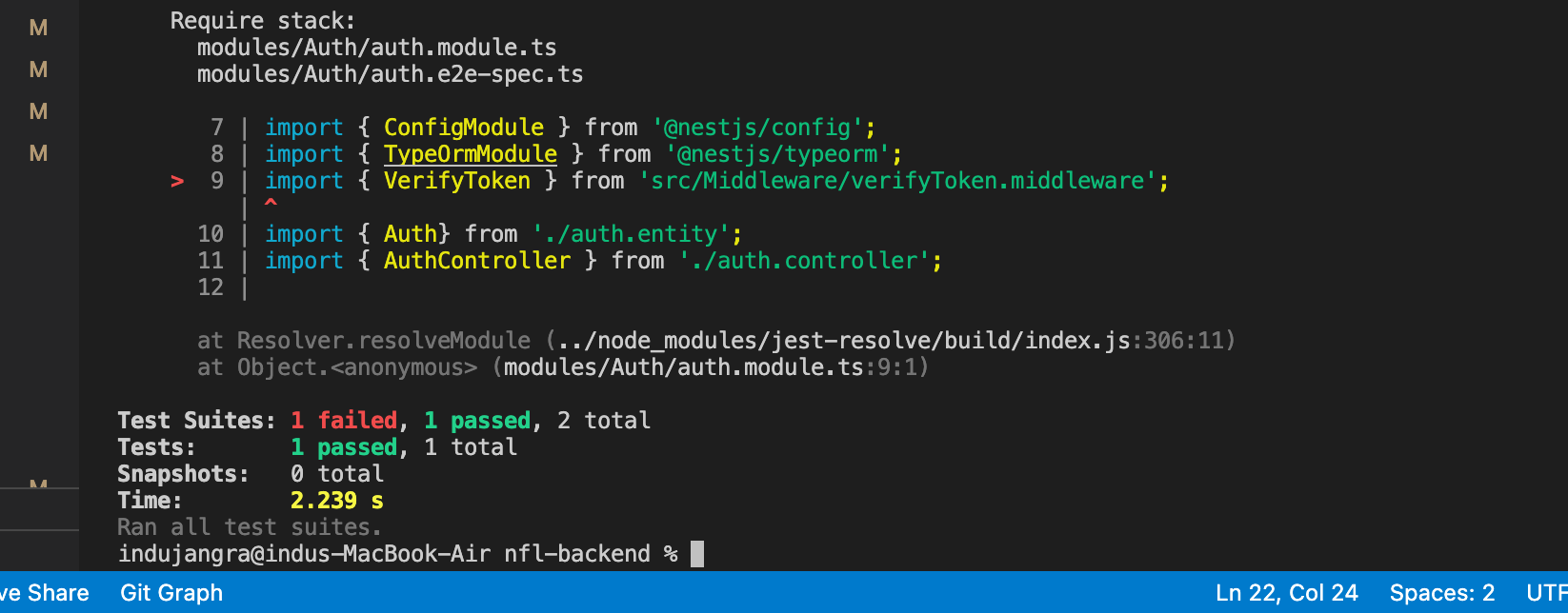
showing error ..... can you please help sir